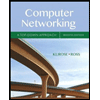
Use C++ Programming language.
The header file below defines a class for a simple hash table:
- hashMap.h Download hashMap.h
- Write a driver program to test the class with the following data:
(520, "3100 Main St, Houston TX ");
(37, "2200 Hayes Rd, Austin TX");
(226, "1775 West Airport St, San Antonio TX");
(273, "3322 Walnut Bend, Houston TX");
(491, "5778 Alabama, Waco TX");
(94, "3333 New St, Paris TX");
Make sure to test the find function for exiting and non-existing data
2. Modify the type of member key in class HashEntry from int to a string (this is useful is the key is e.g. the phone number or e-mail address). Use the string hash function discussed in class (see ppt notes) or search online for alternative functions that work on strings.
3. Modify the collision strategy of class HashMap to do separate chaining instead of linear probing. Be sure to modify the display function so that items in the bucket chains are also displayed in a pre-defined order for debugging purpose.
4. Add a delete function to class HashMap.
Note: You may NOT use the C++ STL map data structure to implement the hash table in this assignment.
Deliverables:
a) Indicate which tasks you completed.
b) Since the tasks above are cumulative, you only need to submit your code for the last task as indicated in (a).
c) Submit screenshots of your program execution showing your program meets the specs of the last task completed.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- A WriteOnly attribute may be implemented automatically. True vs. Falsearrow_forwardIt is python language Write the code that creates a new Node class. It will store data and next attributes. You only need to create the __init__ method. data and next variables will have default values, both set to None. Assume you are using the Node class from the previous connection to create a LinkedList. You have the code below, create a method that removes the first node from the LinkedList. class LinkedList: def __init__(self): self.head = None Based on the code from the last two questions, create a new LinkedList. Add 2 values to the LinkedList (there is an add method that accepts data as an argument, called add). Then call the removeFront method created in the previous question. Based on the previous questions, create a Queue class that uses the LinkedList for its data storage. Create the __init__, isEmpty, insert, remove, and size methods. Assume that LinkedList class has the add, removeFront and size methods defined. Based on the LinkedList code already…arrow_forwarddef upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0arrow_forward
- For any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardFIX THIS CODE Using python Application CODE: import csv playersList = [] with open('Players.csv') as f: rows = csv.DictReader(f) for r in rows: playersList.append(r) teamsList = [] with open('Teams.csv') as f: rows = csv.DictReader(f) for r in rows: teamsList.append(r) plays=len(playersList) for i in range(plays): if playersList[i]['team']=='Argentina' and int(playerlist[i]['minutes played'])<200 and int(playersList[i] ['shots'])>20: print(playersList[i]['last name']) c0=0 c1=0 c2=0 for i in range(len(teamsList)): if int(teamsList[i]['redCards'])==0: c0=c0+1 if int(teamsList[i]['redCards'])==1: c1=c1+1 if int(teamsList[i]['redCards'])==2: c2=c2+1 print("Number of teams with zero redcards:",c0) print("Number of teams with zero redcards:",c1) print("Number of teams with zero redcards:",c2) ratio=0 for i in range(len(teamsList)): if int(teamsList[i]['games']>3) and if int(teamsList[i]['goalsFor'])/int(teamsList[i]['goalsAgainst'])<ratio:…arrow_forwardPythonarrow_forward
- Background Often, data are stored in a very compact but not human-friendly way. Think of how dishes in a menu can be stored in a restaurant database somewhere in the cloud. One way a dish object can be stored is this: { "name": "Margherita", "calories": 800, "price": 18.90, "is_vegetarian": "yes", "spicy_level": 2 } Obviously, this format is not user-readable, so the restaurant's frontend team produced many lines of code to render and display this content in a way that's more understandable to us, the restaurant users. We have a mini-version of the same task coming up. Given a list of similar complex objects (represented by Python dictionaries), display them in a user-friendly way that we'll define in these instructions. What is a "dish"? In this project, one dish item is a dictionary object that is guaranteed to have the following keys: "name": a string that stores the dish's name. "calories": an integer representing the calorie intake for one serving of the dish. "price": a float to…arrow_forwardin C++ Write definition of search, isItemAtEqual, retrieve, remove, print, constructor, and destructor for class hashT based upon a method of your choice . Write a test program to test with at least 3 different hashing functions. No PLAGIARISM please . Thank you!arrow_forwardComplete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forward
- True or False: Given the below code and assuming all syntax is correct, at Point 1, "3" is printed to the console: HashSet-String> hs = new HashSet-String>0; hs.adda"); ha addb); hs adda"); Syrtem.out printinchs size(), // Point 1 True Falsearrow_forward1. Goals and Overview The goal of this assignment is to practice creating a hash table. This assignment also has an alternative, described in Section 5. A hash table stores values into a table (meaning an array) at a position determined by a hash function. 2. Hash Function and Values Every Java object has a method int hashCode() which returns an int suitable for use in a hash table. Most classes use the hashCode method they inherit from object, but classes such as string define their own hashCode method, overriding the one in object. For this assignments, your hash table stores values of type public class HashKeyValue { K key; V value; } 3. Hash Table using Chained Hashing Create a hash table class (called HashTable211.java) satisfying the following interface: public interface HashInterface { void add(K key, V value); // always succeeds as long as there is enough memory V find (K key); // returns null if there is no such key } Internally, your hash table is defined as a private…arrow_forwardIn Perl Prepare a program using package that insert the name of a student (“Jonothan”). The main program will show the name. Hint: can use: constructor that returns objects, and hash reference that holds the attribute name.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
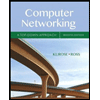
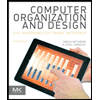
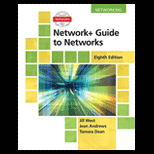
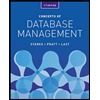
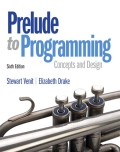
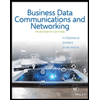