C++ Complete the program that will allow the user to enter data on 10 Halloween costumes. The program will create Costume objects and then store the Costume objects in a Hash Table. The Costume ID will be the key and a pointer to the Costume object will be the value. You are given Lab7.cpp, HashEntry.h, and Costume.h and you should not have to make any changes to these files at all. You will need to write HashTable.h, which implements the HashTable class. This should NOT be a template class. Your HashTable class should resolve collisions by linear probing and should use the HashEntry class for the linkedlist nodes. Because we’re using probing, there is no removal function. This is okay for this application, since we want to keep a record of costumes that are no longer being kept in stock. ---GIVEN--- Lab.cpp #include #include "HashTable.h" #include "Costume.h" using namespace std; int main() { int size; int key; Costume *newCostume; string name; float price; int stock; cout << "\nHello.\n\n"; cout <<"What size is the table? "; cin >> size; //create a Hash Table HashTable myHashTable(size); //fill the table for(int i=0; i> key; cin.ignore(); cout << "NAME: "; getline(cin, name); cout << "PRICE: $"; cin >> price; cout << "STOCK: "; cin >> stock; newCostume = new Costume(key, name, price, stock); myHashTable.putValue(key, newCostume); } cout << "\n\nHERE IS THE TABLE:\n\n"; myHashTable.printHashTable(); cout << endl << endl; return 0; } HashEntry.h #ifndef HASHENTRY_H #define HASHENTRY_H #include #include "Costume.h" using namespace std; class HashEntry { private: int key; Costume* value; public: HashEntry(int k, Costume* v) { this->key = k; this->value = v; } int getKey() { return key; } Costume* getValue() { return value; } void setValue(Costume* v) { value = v; } }; #endif Costume.h #ifndef COSTUME_H #define COSTUME_H #include using namespace std; class Costume { private: //attributes int id; // costume id string costumeName; float price; int stock; public: Costume(int id, string name, float price, int stock){ this->id = id; this->costumeName = name; this->price = price; this->stock = stock; } int getID() const { return this->id; } string getCostumeName() const { return this->costumeName; } float getPrice() const { return this->price; } int getStock() const { return this->stock; } void setStock(int newStock) { stock = newStock; } friend ostream & operator<< (ostream & os, Costume c) { os << c.getID() << ", " << c.getCostumeName(); return os; } }; #endif
C++
Complete the program that will allow the user to enter data on 10 Halloween costumes. The program will create Costume objects and then store the Costume objects in a Hash Table. The Costume ID will be the key and a pointer to the Costume object will be the value.
You are given Lab7.cpp, HashEntry.h, and Costume.h and you should not have to make any changes to these files at all.
You will need to write HashTable.h, which implements the HashTable class. This should NOT be a template class. Your HashTable class should resolve collisions by linear probing and should use the HashEntry class for the linkedlist nodes. Because we’re using probing, there is no removal function. This is okay for this application, since we want to keep a record of costumes that are no longer being kept in stock.
---GIVEN---
Lab.cpp
#include <iostream>
#include "HashTable.h"
#include "Costume.h"
using namespace std;
int main()
{
int size;
int key;
Costume *newCostume;
string name;
float price;
int stock;
cout << "\nHello.\n\n";
cout <<"What size is the table? ";
cin >> size;
//create a Hash Table
HashTable myHashTable(size);
//fill the table
for(int i=0; i<size; i++)
{
cout << "\n*****COSTUME " << i+1 << "*****\n";
cout << "COSTUME ID: ";
cin >> key;
cin.ignore();
cout << "NAME: ";
getline(cin, name);
cout << "PRICE: $";
cin >> price;
cout << "STOCK: ";
cin >> stock;
newCostume = new Costume(key, name, price, stock);
myHashTable.putValue(key, newCostume);
}
cout << "\n\nHERE IS THE TABLE:\n\n";
myHashTable.printHashTable();
cout << endl << endl;
return 0;
}
HashEntry.h
#ifndef HASHENTRY_H
#define HASHENTRY_H
#include <iostream>
#include "Costume.h"
using namespace std;
class HashEntry
{
private:
int key;
Costume* value;
public:
HashEntry(int k, Costume* v)
{
this->key = k;
this->value = v;
}
int getKey()
{
return key;
}
Costume* getValue()
{
return value;
}
void setValue(Costume* v)
{
value = v;
}
};
#endif
Costume.h
#ifndef COSTUME_H
#define COSTUME_H
#include <iostream>
using namespace std;
class Costume
{
private:
//attributes
int id; // costume id
string costumeName;
float price;
int stock;
public:
Costume(int id, string name, float price, int stock){
this->id = id;
this->costumeName = name;
this->price = price;
this->stock = stock;
}
int getID() const
{
return this->id;
}
string getCostumeName() const
{
return this->costumeName;
}
float getPrice() const
{
return this->price;
}
int getStock() const
{
return this->stock;
}
void setStock(int newStock)
{
stock = newStock;
}
friend ostream & operator<< (ostream & os, Costume c)
{
os << c.getID() << ", " << c.getCostumeName();
return os;
}
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

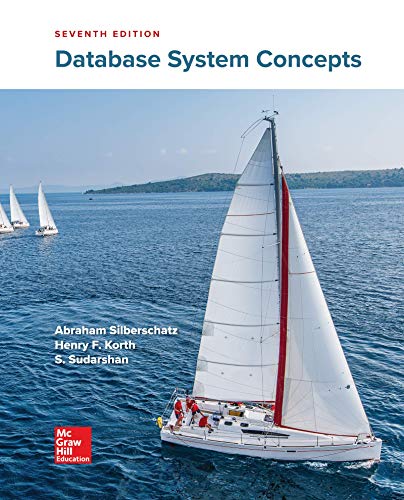
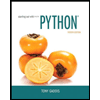
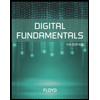
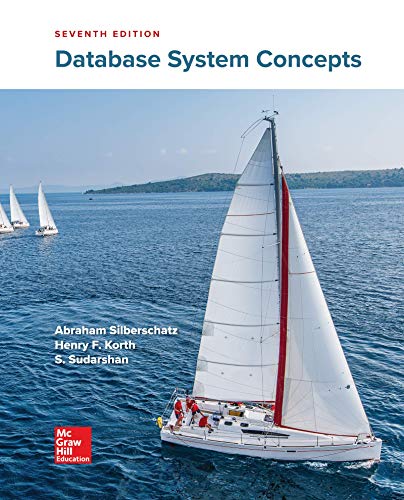
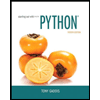
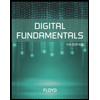
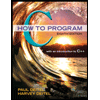
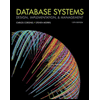
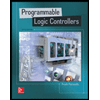