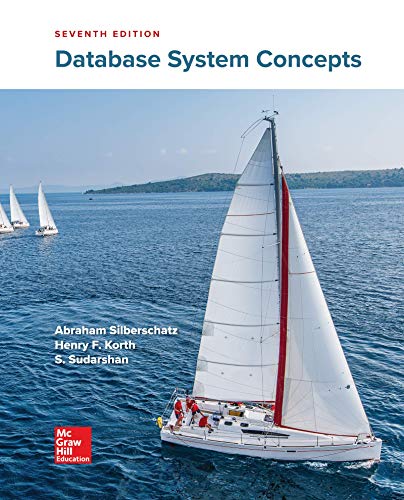
Concept explainers
8.16 LAB: Mileage tracker for a runner C++
Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node.
Ex. If the input is:
3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18the output is:
2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18#include "MileageTrackerNode.h"
#include <string>
#include <iostream>
using namespace std;
int main (int argc, char* argv[]) {
// References for MileageTrackerNode objects
MileageTrackerNode* headNode;
MileageTrackerNode* currNode;
MileageTrackerNode* lastNode;
double miles;
string date;
int i;
// Front of nodes list
headNode = new MileageTrackerNode();
lastNode = headNode;
// TODO: Read in the number of nodes
// TODO: For the read in number of nodes, read
// in data and insert into the linked list
// TODO: Call the PrintNodeData() method
// to print the entire linked list
// MileageTrackerNode Destructor deletes all
// following nodes
delete headNode;
}
MileageTrackerNode.h
#ifndef MILEAGETRACKERNODEH
#define MILEAGETRACKERNODEH
#include <string>
using namespace std;
class MileageTrackerNode {
public:
// Constructor
MileageTrackerNode();
// Destructor
~MileageTrackerNode();
// Constructor
MileageTrackerNode(double milesInit, string dateInit);
// Constructor
MileageTrackerNode(double milesInit, string dateInit, MileageTrackerNode* nextLoc);
/* Insert node after this node.
Before: this -- next
After: this -- node -- next
*/
void InsertAfter(MileageTrackerNode* nodeLoc);
// Get location pointed by nextNodeRef
MileageTrackerNode* GetNext();
void PrintNodeData();
private:
double miles; // Node data
string date; // Node data
MileageTrackerNode* nextNodeRef; // Reference to the next node
};
#endif
MileageTracker.cpp
#include "MileageTrackerNode.h"
#include <iostream>
// Constructor
MileageTrackerNode::MileageTrackerNode() {
miles = 0.0;
date = "";
nextNodeRef = nullptr;
}
// Destructor
MileageTrackerNode::~MileageTrackerNode() {
if(nextNodeRef != nullptr) {
delete nextNodeRef;
}
}
// Constructor
MileageTrackerNode::MileageTrackerNode(double milesInit, string dateInit) {
miles = milesInit;
date = dateInit;
nextNodeRef = nullptr;
}
// Constructor
MileageTrackerNode::MileageTrackerNode(double milesInit, string dateInit, MileageTrackerNode* nextLoc) {
miles = milesInit;
date = dateInit;
nextNodeRef = nextLoc;
}
/* Insert node after this node.
Before: this -- next
After: this -- node -- next
*/
void MileageTrackerNode::InsertAfter(MileageTrackerNode* nodeLoc) {
MileageTrackerNode* tmpNext;
tmpNext = nextNodeRef;
nextNodeRef = nodeLoc;
nodeLoc->nextNodeRef = tmpNext;
}
// Get location pointed by nextNodeRef
MileageTrackerNode* MileageTrackerNode::GetNext() {
return nextNodeRef;
}
void MileageTrackerNode::PrintNodeData(){
cout << miles << ", " << date << endl;
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps

- Please help with the follow question in C++arrow_forwardC++ Consider the following function as a property of a LinkedBag that contains a Doubly Linked List. Assume a Node has pointers prev and next, which can be read and changed with the standard get and set methods. Assume that the doubly linked list is: 1 <--> 2 <--> 3 <--> 4 <--> 5 <-->6 If you are uncertain what the above diagram depicts, it is a doubly linked list such that: The head of this doubly linked list is the node that contains the value 1. The tail of this doubly linked list is the node that contains the value 6. The 3rd node in this list contains the value 3. The contents of this list are the values 1 through 6 in sequential order. The following questions are regarding the linked list after after the test_function is run. A. The head of the list after the test_function is run contains the value: B. The tail of the list after the test_function is run contains the value: C. The 3rd node in the list after the test_function is run…arrow_forwardJava (LinkedList) - Grocery Shopping Listarrow_forward
- c++ Please Dont use Chegg for this problem 15.7 In-Lab Activity 7: Shapes (I/O operator overloading) In this program, you must implement I/O operator overloading (<< and >>) to match a shape's functionality. You must modify the following files: Triangle.h Implement the declaration for the overloaded I/O operator functions. Triangle.cpp Implement the calculateArea() function to find the area of the triangle. Implement the definitions for the overloaded I/O operator functions. The input function will take in two variables: The base and height (cin >> base >> height) Call the object's calculateArea() function after inputting the variables. The output function will send the following to the output stream (for base = 10 and height = 20): Triangle Object: Base: 10 Height: 20 Area is: 100 Square.h Implement the declaration for the overloaded I/O operator functions. Square.cpp Implement the calculateArea() function to find the area of the square. Implement…arrow_forwardc++arrow_forwardJAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forward
- Explain the concept of two-way data binding with custom data types and complex objects. What challenges may arise when implementing it?arrow_forwardC++arrow_forward] ] is_bipartite In the cell below, you are to write a function "is_bipartite (graph)" that takes in a graph as its input, and then determines whether or not the graph is bipartite. In other words, it returns True if it is, and False if it is not. After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (is_bipartite({"A" : ["B", "C"], "B" : ["A"], "C" : ["A"]}), is_bipartite({"A" : ["B", "C"], "B" : ["A", "C"], "C" : ["A", "B"]})) This should return True False Python Pythonarrow_forward
- Java (Generic Method) - What Order?arrow_forward1 Write a C++ code using a while statement to compute the average of all alternative nodes (1st, 3rd, 5th ...) in the given linked list where the first node is pointed to by first. NOTE: Each node has data member that stores the data and the next member that stores the address of the next node. ( means; there are any number of nodes) Use the following declaration: first Node* next; struct Node { }; int data; double total = double average; Node* temp = first; int count 0; = 0;arrow_forward@6 The Reference-based Linked Lists: Select all of the following statements that are true. options: As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
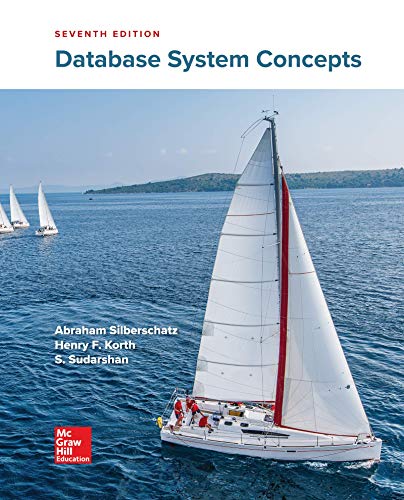
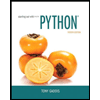
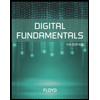
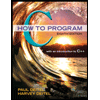
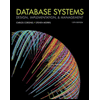
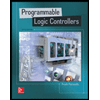