What is a pointer?
The concept of pointers in high-level languages was introduced in C programming. Pointers are simply the variables that are used to store memory addresses of another variable. These special variables are generally used in the languages such as C Programming and C++ Programming, where it allows the programmer to work more deeply with memory and its addresses.
Basics of a pointer
Every variable declared in a program is stored in the allocated memory.
For instance, int x = 10;
This integer variable is represented in the memory as:
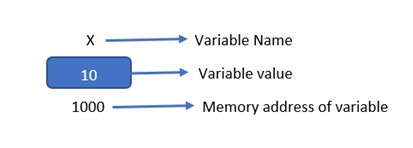
Now, to declare/define a pointer that stores the address of ‘X’, the following syntax is used in C/C++
int *ptr = &x;
This can be represented as:
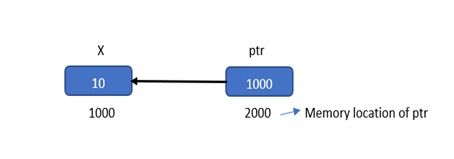
Here, this is generally spoken as “ptr is pointing to integer X” and that is why an arrow is used to represent them diagrammatically.
Size of a pointer variable
In an operating system, the size of a pointer variable is always 4 bytes independent of what type of variable it points to.
Hence, a statement such as,
std::cout<<size(ptr); //printf (“%d”, size(ptr )) in C
will give the output 4.
The data type of a pointer variable is always the same as the variable it is pointing to. While declaring a pointer, it is necessary to give a special sign to it so that the compiler may differentiate between a normal variable and a pointer variable. In C/C++, this special sign is the asterisk (*). Hence, an asterisk is always used while declaring a pointer. When the pointer is not associated with a data type, it is known as a void pointer.
Address-of operator (&)
The ampersand (symbol - &) is known as the address-of operator that returns the memory location of any variable. This operator requires only one operand and is used to assign a value to a pointer variable.
Consider the following statement:
int *ptr = &x;
This statement says, “ptr is a pointer variable (denoted by *) that stores the address of (&) x”.
The memory address which is represented in byte_size is returned using the malloc function. Malloc function is available in the C++ programming language.
A collection of different addresses create an address space. If the collection is made by discrete memory addresses, then it is known as memory space.
Dereferencing pointer
Pointers can also be used to directly assign a new value at the location where it is pointed. This is done by dereferencing a pointer. Dereferencing can also be done by an asterisk (*) operator.
For instance, int main()
{
int x = 10;
int *ptr = &x;
*ptr = 20;
return 0;
}
(i) An integer named X is created and initialized with a value of 10.
(ii) A pointer variable ptr is created and is made to point X variable.
(iii) ptr is dereferenced to store 20.
Uninitialized pointers
One of the common mistakes done by beginner programmers is not to initialize the pointer. When a pointer variable is declared, it is initialized by any random memory chunk.
For instance, int *unin_pointer;
This pointer is left uninitialized. Now, it may point to any memory address. Also, note that memory addresses may contain some useful information. Consider this statement:
*unin_pointer = 0;
This will lead to the loss of important information that was stored in memory.
So always initialize pointers while declaring them. If not needed, then initialize it as NULL (means pointing nowhere or a null pointer). Define the null pointer as:
int *pointer = NULL; OR int *pointer (nullptr);
The second statement can only be used in modern c++11 standards (because the keyword nullptr is introduced in C++11). Also, in the second statement, the constructor is called to create the variable “pointer”.
Arithmetic operations on pointers
The arithmetic calculation can be performed on the pointer. The operation that can be performed on pointers are:
- Incrementing / Decrementing pointers (These are unary operators)
- Subtracting pointers
- Checking for equality of two-pointers.
A pointer is incremented or decremented based on the size of the data type of the variable to which it refers.
The following formula is used to increment a pointer:
(Current Value + sizeof(data type) * n)
Where ‘n’ is the value by which the pointer’s value is to be incremented.
For instance, int x = 10;
int *ptr = &x;
ptr = ptr++;
Let’s say that the address of x is 1000.
Now, a value of ptr is incremented by 1 which means 1000 + 4 * 1 = 1004. So, ptr can be now pointing to memory location 1004.
Pointers and arrays
Arrays are contiguous blocks of memory stored with the same name. When an array is created, the array name points to the first address block of the array.
Pointers can also be used to store the address of the cells of an array. That means a pointer can be used to access the values in an array.
Consider the following array,
int arr[] = {10, 20, 30, 40, 50};
In memory, it can be represented as:

Since the array is contiguous and of integer type, the difference between each element's address is 4 bytes(size of integer type value).
Additionally, one more variable called the pointer variable with the name ‘arr’ is created. It consists of the address of the first element of the array that is, arr[0].
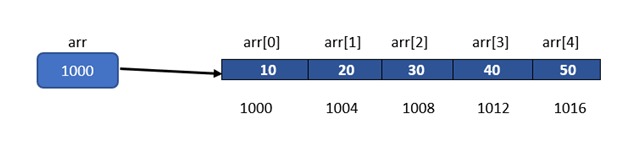
This means if this pointer variable is accessed, using the statement:
cout<<*arr;
then it will display arr[0] or 10.
Now, another pointer can also be made to point arr[0] by the following ways
int *ptr = &arr[0]; Or int *ptr = arr;
This will graphically appear as,
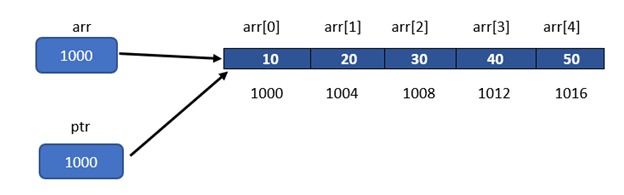
Now, to print complete array, any of the following code can be used:
- for(int i = 0; i < 5; i++)
cout<<arr[i]; //array subscript notation
2. for(int i = 0; i < 5; i++)
cout<<ptr[i]; //pointer subscript notation
3. for(int i = 0; i < 5; i++)
cout<<*(arr + i); //pointer method
4. for(int i = 0; i < 5; i++)
cout<<*(ptr + i); //pointer method
New and delete keywords
Sometimes, it is required to allocate memory dynamically. “new” and “delete” keywords are used for the same purpose. These operators are used in the context of pointers.
The new keyword returns a pointer pointing to a particular data type.
For instance,
int *ptr {nullptr};
ptr = new int;
Now, ptr points to an int memory chunk. (All this happens at the back-end, this is also known as abstraction)
For other data types, the same can be done,
ptr = new char;
ptr = new double;
or more.
The delete keywords are used to release the allocated memory so that it can be reused again.
For instance,
delete ptr;
Delete keyword also works at the back-end and the user does not have to worry about how all this works (Again, abstraction is working here)
After deleting, memory is deallocated and the physical address is available for another entity or local variable.
Context and Applications
- Bachelors in Computer Science
- Masters in Computer Science
Practice Problems
1. What is a pointer?
- A keyword
- Instance variable
- Special variable that stores address
- Constructor
Answer: Option c
Explanation- Pointer is a special variable that stores address.
2. Which of the following is represented by int*ptr?
- Pointer that represents an integer
- Pointer that represents an integral
- Pointer that represents a float value
- Variable that run-time information of two-dimensional array
Answer: Option a
Explanation- It represents a pointer of an integer data type.
3. Which of the following operators is used for dereferencing a pointer?
- &
- ->
- *
- $
Answer: Option c
Explanation- The asterisk (*) operator is used as a dereferencing operator.
4. Which of the following is the correct way to initialize a pointer?
- Assigning the pointer to 0
- Assigning the pointer to a random variable
- Assigning the pointer to a constant value
- Assigning the pointer to a random memory block
Answer: Option d
Explanation- Initialization of pointer is done by random memory chunk.
5. What is the difference between each element's address in an integer array?
- 2 byte
- 4 byte
- 6 byte
- 8 byte
Answer: Option b
Explanation- The difference between each variable’s address is 4 bytes.
Related Concepts
- Smart pointers
- Passing a pointer to functions
- Pointer of functions
- Linked list
- Stack
- Queue
- Other data structures
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Concept of memory addresses in pointers Homework Questions from Fellow Students
Browse our recently answered Concept of memory addresses in pointers homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.