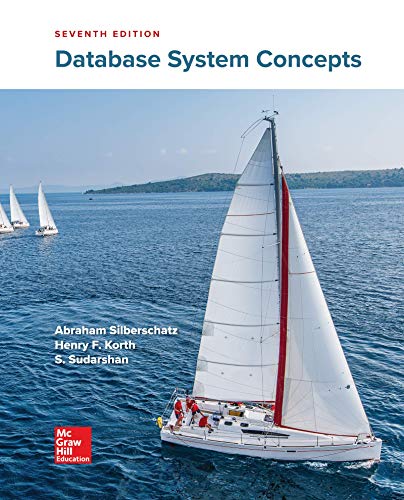
Concept explainers
in java
you have to implement some basic methods for BST's (with a little twist). Each node
in the tree has a data element (an integer) and links (possibly null) to its left and right children (you
can also include parent but not compulsory).
1. Sample TreeNode
class TreeNode
{
int key;
TreeNode left;
TreeNode right;
TreeNode parent; //optional
}
You have to implement the given functions in Binary search Tree class.
2. Binary Search Trees (BST)
You are required to implement a Binary Search Tree (BST) ADT (Abstract Data Type). Following
functionalities are expected.
2.1 Constructor
Creates an empty Binary Search Tree.
2.3 Inserts a new node in BST (Standard Insert)
Inserts a new node in BST.
2.4 Delete
Deletes a node from a BST (Standard Delete)
2.5 Lookup
Find a value in the tree, lookup is a fancy name of find function
2.6 Size
Returns the total number of nodes in a BST
2.7 MaxDepth
Returns the depth of the longest path in a Binary Search Tree.

Trending nowThis is a popular solution!
Step by stepSolved in 8 steps

- Question Assume that we have the following definition of a Linear Unordered Singly integer data. class Node { }; int data; Node "next; Node "previous; public: Node(); //getter setter for all private variables are present class List { Node *first; public: List(); //function to be implemented bool DeleteMin(); };arrow_forwardConsider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable { // First Node of the List private Node<E> first; // Last Node of the List private Node<E> last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the…arrow_forwardin C++, create a binary search tree class using the class templatr below. class BinarySearchTree{ public:BinarySearchTree(); ~BinarySearchTree(); const int & findMin() const; const int & findMax() const; bool contains(cons tint &x) const; bool isEmpty(); void insert(const int &x); void remove(const int &x); private: struct BinaryNode{ int element; BinaryNode *left; BinaryNode *right; BinaryNode(const int &el, BinaryNode *lt, BinaryNode *rt) :element(el), left(lt), right(rt){} }; BinaryNode *root; void insert(const int &x, BinaryNode *&t) const; void remove(const int &x, BinaryNode *&t) const; BinaryNode * findMin(BinaryNode *t) const; BinaryNode * findMax(BinaryNode *t) const; bool contains(cons tint &x, BinaryNode *t) const; };arrow_forward
- All the methods as well as the main method/tester statements must be written in one class. DO NOT write a different class for each method. NOTE: USE PYTHON AD SOLVE BOTH QUESTIONS IF YOU WANT A THUMBS_UP. 1. Print elements of all the Nodes of a tree using In-order Traversal. 2. Print elements of all the Nodes of a tree using Post-order Traversal.arrow_forwardplease write code both in java an pythonarrow_forwardI need help in converting the following Java code related to BST to a pseudocode. public static class Node { //instance variable of Node class public String data; public Node left; public Node right; //constructor public Node(String data) { this.data = data; this.left = null; this.right = null; } } public void deleteANode(Node node) { deleteNode(this.root, node); } private Node deleteNode(Node root, Node node) { // check for node initially if (root == null) { return null; } else if (node.data.length() < root.data.length()) { // process the left sub tree root.left = deleteNode(root.left, node); } else if (node.data.length() > root.data.length()) { // process the right sub tree root.right = deleteNode(root.right, node); } else if(root.data==node.data){ // case 3: 2 child…arrow_forward
- Question 01: Implement the following UML class diagram. Student -name:String -address:String -numCourses: int - 0 -courses:String[30] = {} -grades:int[30] - (} +Student (name:String, address:String) +getName ():String +getAddress():String +setAddress (address:String):void +tostring():String •- +addCourseGrade(course:String,grade:int):void +printGrades ():void +getAverageGrade():double "name (address)" "name coursel:grade1, course2:grade2,..." Suppose that the application requires you to model students. A student has a name and an address. You are required to keep track of the courses taken by each student, together with the grades (between 0 and 100) for each of the courses. A student shallarrow_forwardcomptuer sciecne helparrow_forwardAssume we have an IntBST class, which implements a binary search tree of integers. The field of the class is a Node variable called root that refers to the root element of the tree. 1) Write a recursive method for this class that computes and returns the sum of all integers less than the root element. Assume the tree is not empty and there is at least one element less than the root. 2) Write a recursive method that prints all of the leaves, and only the leaves, of a binary search tree. 3) Write a method, using recursion or a loop, that returns the smallest element in the tree.arrow_forward
- Create a function that uses Node * pointing to root of AVL Tree as an input and returns the height of the AVL tree in O(log(n)).arrow_forwardWhich one is NOT an example of software reuse? A I have an abstract class Game, I will define Chess, Backgammon, and Checkers as subclasses of class Game. B I have a LinkedList implementation, I will use it to implement a Set class through delegation. C I need to develop a structure that has recursive composition, I will make use of composite design pattern. D I have already written a code segment that scans a list and finds duplicates, I will copy and paste that code segment into another program that I am developing. E I have an implementation of List class which contains elements of type integer, I will use it to develop a list that contains elements of type Car.arrow_forwardIn Java I'm trying to make a Red Black Tree through inheritance with the Binary Tree I created (as provided along with the Binary Node) yet I'm having a lot of difficulty knowing where and how to start as well as how to override and even create the Insert and Remove functions for the Red Black Tree. Code and assistance in this topic will be greatly appreciated... here is the code I'm utilizing for this project. Binary Node I created: public class BinNode{private String data;private BinNode left;private BinNode right;private int height; //height fieldpublic BinNode(){data = "";left = null;right = null;}public int getHeight() {return height;}public void setHeight(int height) {this.height = height;}public BinNode(String d){data = d;left = null;right = null;}public void setData(String d){this.data = d;}public String getData(){return this.data;}public void setLeft(BinNode l){this.left = l;}public BinNode getLeft(){return this.left;}public void setRight(BinNode r){this.right = r;}public…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
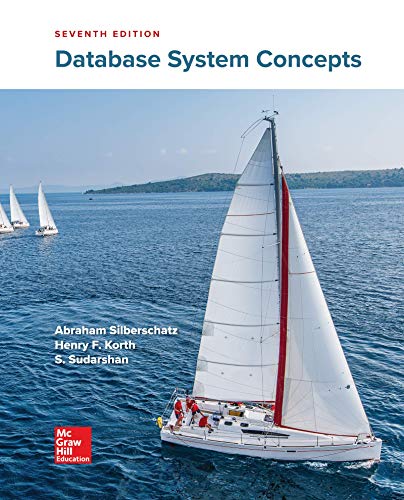
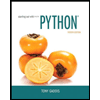
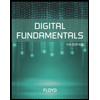
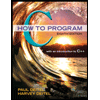
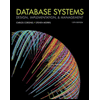
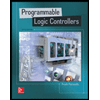