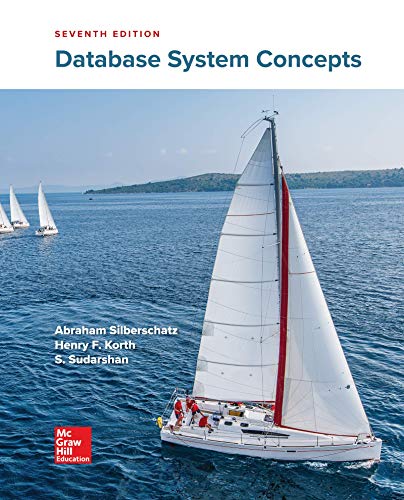
I need help in converting the following Java code related to BST to a pseudocode.
public static class Node {
//instance variable of Node class
public String data;
public Node left;
public Node right;
//constructor
public Node(String data) {
this.data = data;
this.left = null;
this.right = null;
}
}
public void deleteANode(Node node) {
deleteNode(this.root, node);
}
private Node deleteNode(Node root, Node node) {
// check for node initially
if (root == null) {
return null;
} else if (node.data.length() < root.data.length()) {
// process the left sub tree
root.left = deleteNode(root.left, node);
} else if (node.data.length() > root.data.length()) {
// process the right sub tree
root.right = deleteNode(root.right, node);
} else if(root.data==node.data){
// case 3: 2 child
if (root.left != null && root.right != null) {
String lmax = findMaxData(root.left);
root.data = lmax;
root.left = deleteNode(root.left, new Node(lmax));
return root;
}
//case 2: one child
// case i-> has only left child
else if (root.left != null) {
return root.left;
}
// case ii-> has only right child
else if (root.right != null) {
return root.right;
}
//case 1:- no child
else {
return null;
}
}
return root;
}
// inorder successor of given node
public String findMaxData(Node root) {
if (root.right != null) {
return findMaxData(root.right);
} else {
return root.data;
}
}

Step by stepSolved in 2 steps

- Draw a UML class diagram for the following code: class Node: def __init__(self, value): self.value = value self.next = None class Stack: def __init__(self): self.top = None def isEmpty(self): return self.top is None def push(self, item): new_node = Node(item) new_node.next = self.top self.top = new_node def pop(self): if self.isEmpty(): raise Exception("Stack is empty") popped_item = self.top.value self.top = self.top.next return popped_item def popAll(self): items = [] while not self.isEmpty(): items.append(self.pop()) return items[::-1] def peek(self): if self.isEmpty(): raise Exception("Stack is empty") return self.top.value # Verificationstack = Stack()print("Is stack empty?", stack.isEmpty()) stack.push(10)stack.push(20)stack.push(30)print("Top item:", stack.peek()) print("Popped item:", stack.pop())print("Popped…arrow_forwardcomptuer sciecne helparrow_forwardExplain the following code : public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
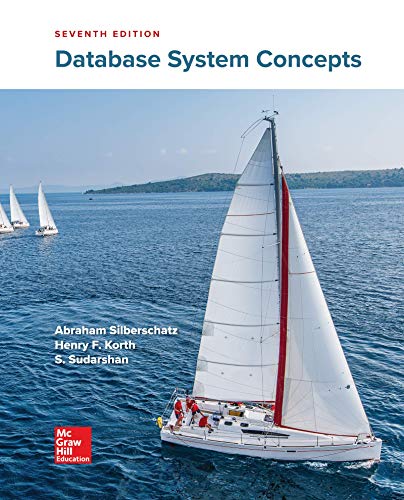
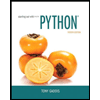
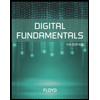
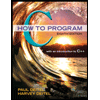
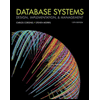
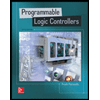