All the requirements from that class are still in force. You'll be making five major changes to the class. Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction. Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include . (Note, I don't expect the variable to be named "denominatorParameter," that's just my placeholder for the example.) assert() is not the best way to handle this, but it will have to do until we study exception handling.
All the requirements from that class are still in force. You'll be making five major changes to the class. Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction. Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include . (Note, I don't expect the variable to be named "denominatorParameter," that's just my placeholder for the example.) assert() is not the best way to handle this, but it will have to do until we study exception handling.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
For this assignment you will be building on the Fraction class you began last week. All the requirements from that class are still in force. You'll be making five major changes to the class.
- Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction.
Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include <cassert>. (Note, I don't expect the variable to be named "denominatorParameter," that's just my placeholder for the example.)
assert() is not the best way to handle this, but it will have to do until we study exception handling. - Add the const keyword to your class wherever appropriate (and also make sure to pass objects by reference -- see lesson 15.9). Your class may still work correctly even if you don't do this correctly, so this will require extra care!!
- Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object.
Expert Solution

Step 1 Introduction
In C++ which refers to an object-oriented programming language which gives a clear structure to programs and also which allows code to be reused, lowering development costs. C++ which is portable and that can be used to develop applications that can be adapted to multiple platforms. C++ which is also a general-purpose programming language
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
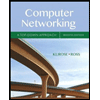
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
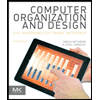
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
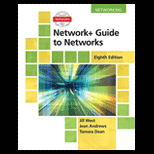
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
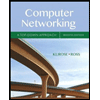
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
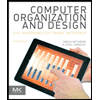
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
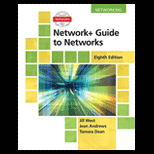
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
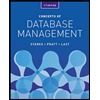
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
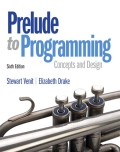
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
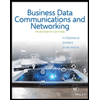
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY