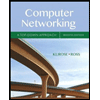
Make C# (Sharp): console project named MyPlayList.
Create an Album class with 4 fields to keep track of title, artist, genre, and copies sold. Each field should have a get/set property. Your Album class should have a constructor to initialize the title (other 3 variables should default to null or 0). In addition, create a public method in the Album class to calculate amount sold for the album (copies sold * cost_of_album). You determine the cost of each album. Also create a method in the Album class to display the information. Print out of information should look something like this:
The album Tattoo You by the Rolling Stones, a Rock group, made $25,000,000.
In the main method, create 3 instances of the Album class with different albums, use setters to set values of the artist, genre, and copies sold. And then use getters to display information about each on the console.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- __eq__(self, other): Method that returns True if self and other are considered the same Flight: if the origin and destination are the same for both Flights. Make sure that if “other” variable is not a Flight object, this means False should be returned. getFlightNumber(self): Getter that returns the Flight number getStart(self): Getter that returns the Plane Start getgoingTo(self): Getter that returns the Plane destination isDomesticFlight(self): Method that returns True if the flight is domestic, EX within a country (the Start and goingTo are in the same country); returns False if the flight is international (the Start and goingTo are in different countries) setStart(self, origin): Setter that sets (updates) the Plane Start setgoingTo(self, destination): Setter that sets (updates) the Plane GoingToarrow_forwardJAVA Use the numbers 65, -264, -43 to test out code.arrow_forwardStockReader Class • This class will contain the method necessary to read and parse the .csv file of stock information. • This class shall contain a default constructor with no parameters. The constructor should be made private. . This is to prevent the class from being instantiated since the class will only contain static methods. • This class shall contain no data fields. • This class shall have a method called readStockData: . This method shall be a public, static, method. . This method shall return a StockList object once all data has been processed. . This method will take a File object as a parameter. This File object should link to the stock market data in your files folder created previously. . This method must validate that the given File object is a .csv file. If it is not, then this method shall throw an IllegalArgumentException. . This method shall read the File object and process all of the stock data into TeslaStock objects and store each object in a StockList. NOTE: The…arrow_forward
- StockList Class • This class will contain the methods necessary to display various information about the stock data. • This class shall be a subclass of ArrayList. • This class shall not contain any data fields. (You don't need them for this class if done correctly). • This class shall have a default constructor with no parameters. o No other constructors are allowed. • This class shall have the following public, non-static, methods: • printAllStocks: This method shall take no parameters and return nothing. ▪ This method should print each stock in the StockList to an output file. ▪ NOTE: This method shall NOT print the data to the console. The output file shall be called all_stock_data.txt. The data should be printed in a nice and easy to read format. o displayFirstTenStocks: This method shall take no parameters and return nothing. ▪ This method should display only the first 10 stocks in the StockList in a nice and easy to read format. ▪ This method should display the data in the…arrow_forwardAttributes item_name (string) item_price (int) item_quantity (int) Default constructor Initializes item's name="none", item's price = 0, item's quantity=0 Method print_item_cost() Ex of print_item_cost() output: Bottled Water 10 @ $1 = $10 in the main section of your code, prompt the user for two items and create two objects of the ItemToPurchase class. Exc Item 1 Enter the item name: Chocolate Chips Enter the item price: 3 Enter the item quantity: 1 Item 2 Enter the item name: Bottled Water Enter the item price: 1 Enter the item quantity: 10 Add the costs of the two items together and output the total cost Ex TOTAL COST Chocolate Chips 1 @ $3-$3 Bottled Water 10 @ $1= $10 Total: $13arrow_forwardInvoicing.java Create a class named Invoicing (no instance private data fields) that includes three overloaded computeInvoice() methods and one displayDetails() method for a book store: see pages 140 and 183 for examples... * Define a constant for the 8% tax rate at the top of the Invoicing class. Set the TAXRATE double constant to 0.08. Do not set to a whole number. When computeInvoice() receives a single parameter, it represents the price of one book ordered. Calculate the tax using the TAXRATE constant. Add the tax to the price and save the total due in a variable. Call the displayDetails() method, defined in the computeInvoice() class, being sure to pass the total due as an argument to the display method. * When computeInvoice() receives two parameters, they represent the price of a book and the quantity ordered. Multiply the price times quantity, calculate the tax using the TAXRATE constant, and calculate the total due. Call the displayDetails() method, defined in the…arrow_forward
- 1. Create a class as described below: Class Name: Room Fields: standard room fee amenities total cost Constructors:A default constructor to initialize the fields. The standard room fee field should be set to $200 and the amenities field to zero. Another constructor with one argument. It should assign this value to the standard room fee and the amenities field to the default value of $75. Methods: getStandardFee() - This methods returns the standard room fee field value. getAmenities() - This method returns the amenities field value. getTotalCost() - This method returns the total cost field value. calcTotalCost() - This method calculated the total cost for an instance of the class setStandard Fee() - This method has one argument representing the standard room fee. setAmenities() - This method has one argument representing the amenities for the room.arrow_forwarda - Create a FitnessTracker class that includes data fields for a fitness activity, the number of minutes spent participating, and the date. The class includes methods to get each field. In addition, create a default constructor that automatically sets the activity to running, the minutes to 0, and the date to January 1 of the current year. Save the file as FitnessTracker.java. Create an application that demonstrates each method works correctly, and save it as TestFitnessTracker.java. b - Create an additional overloaded constructor for the FitnessTracker class you created in Exercise 3a. This constructor receives parameters for each of the data fields and assigns them appropriately. Add any needed statements to the TestFitnessTracker application to ensure that the overloaded constructor works correctly, save it, and then test it. c - Modify the FitnessTracker class so that the default constructor calls the three-parameter constructor. Save the class as FitnessTracker2.java. Create an…arrow_forwardFor your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
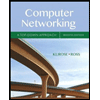
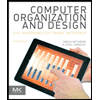
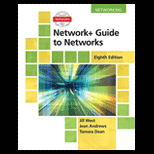
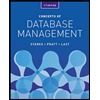
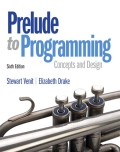
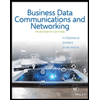