This exercise assesses the skills required to develop user defined functions, and pointers. Bonus Task: Develop the program with Graphics properties to give a better look to your program. You need to explore the graphics libraries available in C++ e.g. * I need to solve this Q with #include and #include Not other libary .* The cod must Run in DEV C++. Please do not use std:: or getliner for cod . Exercise 2 Write a C++ program to develop a Vehicle Fine Management System for Police. The program will have the following features. 1. A user defined function called “Student Registration”. This function prompts a user to enter a student’s personal data i.e., Student Number, Student Name, Age and City and stores them. 2. A user defined function called “Module Enrolment”. This function takes student number from themain function as an argument and prompts a user to enter student two modules details i.e. Module name, Module Code and Module Credits Hours (15 or 30) and stores them. The programs should display an error message if a user enters credit hour other than 15 or 30. 3. A user defined function called “Student Assessment”. This function takes student number from the main function as an argument and prompts a user to enter Module 1 and Module 2 marks and stores them. 4. A user defined function which takes a Student ID and search option (1 for Personal data search, 2 for Module Search and 3 for Assessment Search) from the main function and Searches Student data according to the option (1,2 or 3) You are also required to create a Menu in the main function which displays all the above options to a user. Upon selection of an option from the Menu, program will call the relevant function and will pass the arguments. The program will also have an option to exit the menu that will end the program. Sample Output: ************* * Welcome to Student Management System * * ************ 1- Student Registration 2- Module Enrollment 3- Student Assessment 4- Search Student 5- Exit Enter your choice (1-5): 2 Enter Student ID: 11111111 Enter Module 1 Name: PDI Enter Module 1 Code: UFCEXX-30-1 Modules Enrolled Similarly, you will have output for all the above options.
This exercise assesses the skills required to develop user defined functions, and pointers.
Bonus Task:
Develop the program with Graphics properties to give a better look to your program. You need to explore the graphics libraries available in C++ e.g. <Graphic.h>
* I need to solve this Q with #include <iostream> and #include <straing> Not other libary .*
The cod must Run in DEV C++.
Please do not use std:: or getliner for cod .
Exercise 2
Write a C++ program to develop a Vehicle Fine Management System for Police. The program will have the following features.
1. A user defined function called “Student Registration”. This function prompts a user to enter a student’s personal data i.e., Student Number, Student Name, Age and City and stores them.
2. A user defined function called “Module Enrolment”. This function takes student number from themain function as an argument and prompts a user to enter student two modules details i.e. Module name, Module Code and Module Credits Hours (15 or 30) and stores them. The programs should display an error message if a user enters credit hour other than 15 or 30.
3. A user defined function called “Student Assessment”. This function takes student number from the main function as an argument and prompts a user to enter Module 1 and Module 2 marks and stores
them.
4. A user defined function which takes a Student ID and search option (1 for Personal data search, 2 for Module Search and 3 for Assessment Search) from the main function and Searches Student data according to the option (1,2 or 3)
You are also required to create a Menu in the main function which displays all the above options to a user. Upon selection of an option from the Menu, program will call the relevant function and will pass the
arguments. The program will also have an option to exit the menu that will end the program.
Sample Output:
*************
* Welcome to Student Management System *
* ************
1- Student Registration
2- Module Enrollment
3- Student Assessment
4- Search Student
5- Exit
Enter your choice (1-5): 2
Enter Student ID: 11111111
Enter Module 1 Name: PDI
Enter Module 1 Code: UFCEXX-30-1
Modules Enrolled
Similarly, you will have output for all the above options.

Step by step
Solved in 3 steps with 1 images

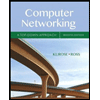
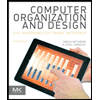
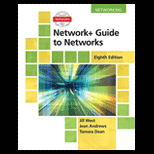
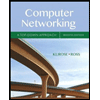
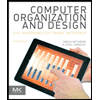
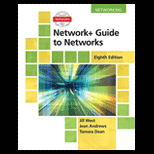
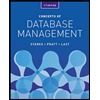
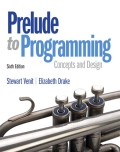
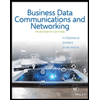