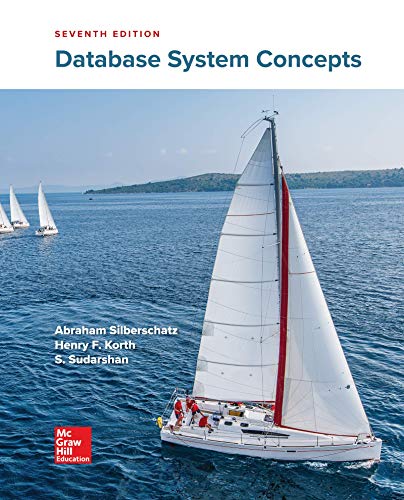
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Please answer in C++ and give explanation
LAB 17.1-B Linked List
Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program.
-------------------------------------------------------------------------------------------------------------------------------
class ItemList
{
private:
struct ListNode
{
int value;
ListNode * next;
};
ListNode * head;
public:
ItemList();
// Post: List is the empty list.
bool IsThere(int item) const;
// Post: If item is in the list IsThere is
// True; False, otherwise.
void Insert(int item);
// Pre: item is not already in the list.
// Post: item is in the list.
void Delete(int item);
// Pre: item is in the list.
// Post: item is no longer in the list.
void Print() const;
// Post: Items on the list are printed on the screen.
int GetLength() const;
// Post: Length is equal to the number of items in the
// list.
~ItemList();
// Post: List has been destroyed.
};
-------------------------------------------------------------------------------------------------------------------------------
Exercise 1: Write the definitions for the member functions. Save the code in file ItemList.cpp.
Exercise 2: Write a driver program that reads values from file int.dat, inserts them into the list, print the length of the final list, and prints the items. Be sure that your driver checks for the preconditions on the member functions of class ItemList before calling them. How many items are in the list? If your answer is not 11, your driver did not adhere to the preconditions. Correct your driver and return your program.
Exercise 3: Add code to your driver to test the remaining member functions. Delete -47, 1926, and 2000 and print the list to confirm that they are gone.
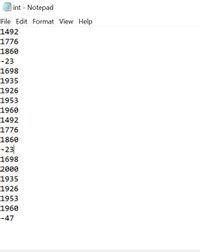
Transcribed Image Text:int - Notepad
File Edit Format View Help
1492
1776
1860
-23
1698
1935
1926
1953
1960
1492
1776
1860
-23
1698
2000
1935
1926
1953
1960
-47
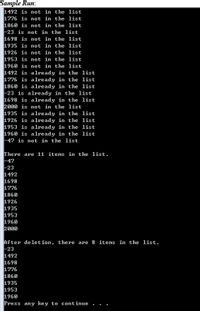
Transcribed Image Text:Sample Run:
1492 is not in the list
1776 is not in the list
1860 is not in the list
23 is not in the list
1698 is not in the list
1935 is not in the list
1926 is not in the list
1953 is not in the list
1960 is not in the list
1492 is already in the list
1776 is already in the list
1860 is already in the list
-23 is already in the list
1698 is already in the list
2000 is not in the list
1935 is already in the list
1926 is already in the list
1953 is already in the list
1960 is already in the list
47 is not in the list
There are 11 items in the list.
F47
-23
1492
1698
1776
1860
1926
1935
1953
1960
2000
After deletion, there are 8 items in the list.
23
1492
1698
1776
1860
1935
1953
1960
Press any ke y to continue
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please help me design a Passsenger class in C++. THANK YOU! Develop a high-quality, object-oriented C++ program that performs a simulation using a heap implementation of a priority queue. A simulation creates a model of a real-world situation, allowing us to introduce a variety of conditions and observe their effects. For instance, a flight simulator challenges a pilot to respond to varying conditions and measures how well the pilot responds. Simulation is frequently used to measure current business practices, such as the number of checkout lines in a grocery store or the number of tellers in a bank, so that management can determine the fewest number of employees required to meet customer needs. Airlines have been experimenting with different boarding procedures to shorten the entire boarding time, keep the flights on-time, reduce aisle congestion, and make the experience more pleasant for passengers and crew. A late-departing flight can cause a domino effect: the departure gate is…arrow_forwardDescribe how polymorphism enables you to program "in the general" rather than "in the specific"? Give some examples, not the ones used in the book (C++ How to Program by Paul J. Deitel, Harvey Deitel Tenth Edition).arrow_forwardUse Python for this question. Also please comment what each line of code means for this question as well: Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty,…arrow_forward
- What exactly are you referring to when you say something is an Abstract Data Type?arrow_forwardI am learning OOD in C++. Is there a good example of a dice game that can help teach me how to understand C++ and object oriented design? Concepts such as Using classes abstract data types including: using constructors and destructors, using pointers, using inheritance, providing for exception handling, and using recursive definitions? I myself would like to come up with a game that titled 'high roller' where a player could play a computer where each have 3 dice and the highest score wins. Pretty simple but I am still learning these concepts. Thank you,arrow_forwardPlease use Python for this question: 1. Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using inheritance. Note that this means you may be able to inherit some of the methods below: a. Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. b. push() – take an item as input and push it on the top of the stack c. pop() – remove and return the item at the top of the stack d. isEmpty() – returns True if the stack is empty, False otherwise e. [] – return the item at a given location, [0] is at the bottom of the stack f.…arrow_forward
- An Abstract Data Type's interface is comprised of what? Here's where you put your reply.arrow_forwardExplain how to use wrapper classes for non-object data types so that you don't end up with a jumbled bag of non-object and object values?arrow_forwardPlease help me design a Airworthy class in C++. THANK YOU! Develop a high-quality, object-oriented C++ program that performs a simulation using a heap implementation of a priority queue. A simulation creates a model of a real-world situation, allowing us to introduce a variety of conditions and observe their effects. For instance, a flight simulator challenges a pilot to respond to varying conditions and measures how well the pilot responds. Simulation is frequently used to measure current business practices, such as the number of checkout lines in a grocery store or the number of tellers in a bank, so that management can determine the fewest number of employees required to meet customer needs. Airlines have been experimenting with different boarding procedures to shorten the entire boarding time, keep the flights on-time, reduce aisle congestion, and make the experience more pleasant for passengers and crew. A late-departing flight can cause a domino effect: the departure gate is…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
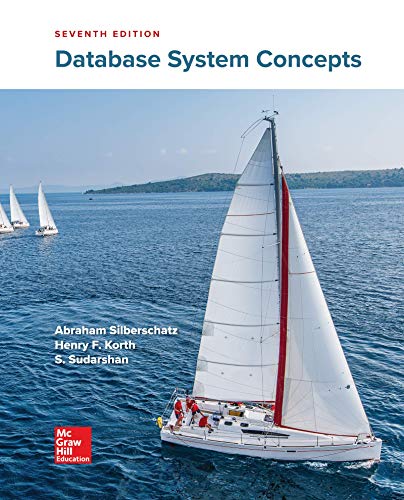
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
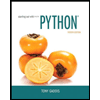
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
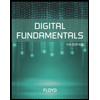
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
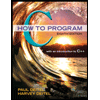
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
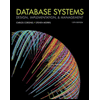
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
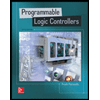
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education