use Advanced C++ techniques, containers and features to refactor the C/C++ algorithm. The purpose is to replace appropriate declarations and code segments with Advanced C++ declarations and code. * Replace all arrays with appropriate STL containers. * Use STL algorithms to REPLACE existing logic where appropriate. * Use smart and move pointers where pointers are needed. * Use lambda expressions where appropriate. * Use C++ style casting when needed. * Look for opportunities where tuples could be used. * The code listing is in C and C++. If there are logic or syntax problems, please fix. char algorithm1(char c,int s) { if (isalpha(c)) { c = toupper(c); c = (((c - 65) + s) % 26) + 65; } return c; } int algorithm2(string input) { do { string output = ""; int shift = rand() % 26; for (int x = 0; x < input.length(); x++) output += algorithm1(input[x],shift); cout << output << endl; } while (!input.length() == 0); }
use Advanced C++ techniques, containers and features to refactor the C/C++
replace appropriate declarations and code segments with Advanced C++ declarations and code.
* Replace all arrays with appropriate STL containers.
* Use STL algorithms to REPLACE existing logic where appropriate.
* Use smart and move pointers where pointers are needed.
* Use lambda expressions where appropriate.
* Use C++ style casting when needed.
* Look for opportunities where tuples could be used.
* The code listing is in C and C++.
If there are logic or syntax problems, please fix.
char algorithm1(char c,int s)
{
if (isalpha(c))
{
c = toupper(c);
c = (((c - 65) + s) % 26) + 65;
}
return c;
}
int algorithm2(string input)
{
do
{
string output = "";
int shift = rand() % 26;
for (int x = 0; x < input.length(); x++)
output += algorithm1(input[x],shift);
cout << output << endl;
}
while (!input.length() == 0);
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

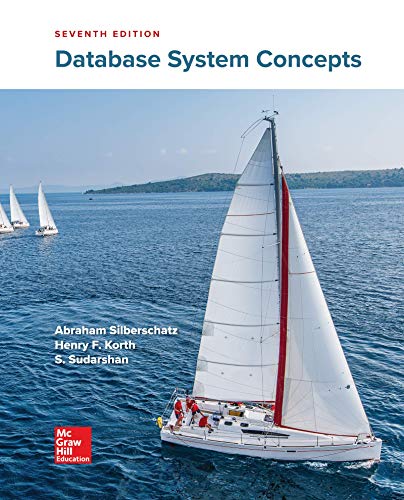
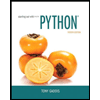
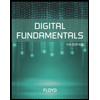
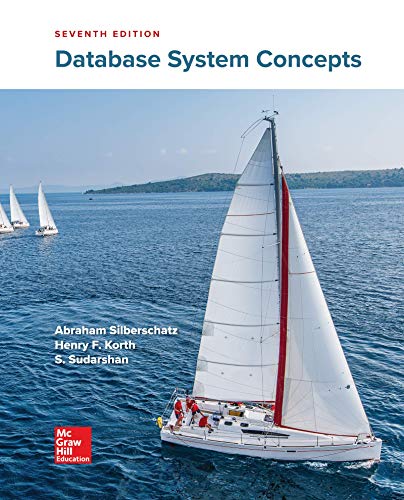
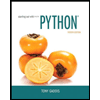
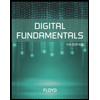
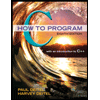
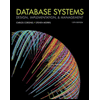
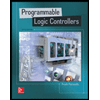