using System; using System.Linq; class main { publicstaticvoid Main (string[] args) { Console.WriteLine("Hi, my name is Stevie. I am thinking of an Animal.\nGuess which animal I am thinking of> "); string[] words = newstring[7]{"sheep","goat","lion","horse","dog","cat","lizard"}; Random rnd = new Random(); int random = rnd.Next(0, 5); string secretWord = words[random]; secretWord = secretWord.ToUpper(); int lives = 5; int counter = -1; int wordLength = secretWord.Length; char[] secretArray = secretWord.ToCharArray(); char[] printArray = newchar[wordLength]; char[] guessedLetters = newchar[26]; int numberStore = 0; bool victory = false; foreach(char letter in printArray) { counter++; printArray[counter] = '-'; } while(lives > 0) { counter = -1; string printProgress = String.Concat(printArray); bool letterFound = false; int multiples = 0; if (printProgress == secretWord) { victory = true; break; } if (lives > 1) { Console.WriteLine("You have {0} lives!", lives); } else { Console.WriteLine("You only have {0} life left!!", lives); } Console.WriteLine(printProgress); Console.Write("\n"); Console.Write("Guess a letter: "); string playerGuess = Console.ReadLine(); bool guessTest = playerGuess.All(Char.IsLetter); while (guessTest == false || playerGuess.Length != 1) { Console.WriteLine("Please enter only a single letter!"); Console.Write("Guess a letter: "); playerGuess = Console.ReadLine(); guessTest = playerGuess.All(Char.IsLetter); } playerGuess = playerGuess.ToUpper(); char playerChar = Convert.ToChar(playerGuess); if (guessedLetters.Contains(playerChar) == false) { guessedLetters[numberStore] = playerChar; numberStore++; foreach(char letter in secretArray) { counter++; if (letter == playerChar) { printArray[counter] = playerChar; letterFound = true; multiples++; } } if (letterFound) { Console.WriteLine("Found {0} letter {1}!", multiples, playerChar); } else { Console.WriteLine("No letter {0}!", playerChar); lives--; } Console.WriteLine((lives)); } else { Console.WriteLine("You already guessed {0}!!", playerChar); } } if (victory) { Console.WriteLine("\n\nThe word was: {0}", secretWord); Console.WriteLine("\n\nYOU WIN!!!!!!!!!!!"); } else { Console.WriteLine("\n\nThe word was: {0}", secretWord); Console.WriteLine("\n\nYOU LOSE!!!!!!!!!"); } } }
using System; using System.Linq; class main { publicstaticvoid Main (string[] args) { Console.WriteLine("Hi, my name is Stevie. I am thinking of an Animal.\nGuess which animal I am thinking of> "); string[] words = newstring[7]{"sheep","goat","lion","horse","dog","cat","lizard"}; Random rnd = new Random(); int random = rnd.Next(0, 5); string secretWord = words[random]; secretWord = secretWord.ToUpper(); int lives = 5; int counter = -1; int wordLength = secretWord.Length; char[] secretArray = secretWord.ToCharArray(); char[] printArray = newchar[wordLength]; char[] guessedLetters = newchar[26]; int numberStore = 0; bool victory = false; foreach(char letter in printArray) { counter++; printArray[counter] = '-'; } while(lives > 0) { counter = -1; string printProgress = String.Concat(printArray); bool letterFound = false; int multiples = 0; if (printProgress == secretWord) { victory = true; break; } if (lives > 1) { Console.WriteLine("You have {0} lives!", lives); } else { Console.WriteLine("You only have {0} life left!!", lives); } Console.WriteLine(printProgress); Console.Write("\n"); Console.Write("Guess a letter: "); string playerGuess = Console.ReadLine(); bool guessTest = playerGuess.All(Char.IsLetter); while (guessTest == false || playerGuess.Length != 1) { Console.WriteLine("Please enter only a single letter!"); Console.Write("Guess a letter: "); playerGuess = Console.ReadLine(); guessTest = playerGuess.All(Char.IsLetter); } playerGuess = playerGuess.ToUpper(); char playerChar = Convert.ToChar(playerGuess); if (guessedLetters.Contains(playerChar) == false) { guessedLetters[numberStore] = playerChar; numberStore++; foreach(char letter in secretArray) { counter++; if (letter == playerChar) { printArray[counter] = playerChar; letterFound = true; multiples++; } } if (letterFound) { Console.WriteLine("Found {0} letter {1}!", multiples, playerChar); } else { Console.WriteLine("No letter {0}!", playerChar); lives--; } Console.WriteLine((lives)); } else { Console.WriteLine("You already guessed {0}!!", playerChar); } } if (victory) { Console.WriteLine("\n\nThe word was: {0}", secretWord); Console.WriteLine("\n\nYOU WIN!!!!!!!!!!!"); } else { Console.WriteLine("\n\nThe word was: {0}", secretWord); Console.WriteLine("\n\nYOU LOSE!!!!!!!!!"); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
using System;
using System.Linq;
class main
{
publicstaticvoid Main (string[] args)
{
Console.WriteLine("Hi, my name is Stevie. I am thinking of an Animal.\nGuess which animal I am thinking of> ");
string[] words = newstring[7]{"sheep","goat","lion","horse","dog","cat","lizard"};
Random rnd = new Random();
int random = rnd.Next(0, 5);
string secretWord = words[random];
secretWord = secretWord.ToUpper();
int lives = 5;
int counter = -1;
int wordLength = secretWord.Length;
char[] secretArray = secretWord.ToCharArray();
char[] printArray = newchar[wordLength];
char[] guessedLetters = newchar[26];
int numberStore = 0;
bool victory = false;
foreach(char letter in printArray)
{
counter++;
printArray[counter] = '-';
}
while(lives > 0)
{
counter = -1;
string printProgress = String.Concat(printArray);
bool letterFound = false;
int multiples = 0;
if (printProgress == secretWord)
{
victory = true;
break;
}
if (lives > 1)
{
Console.WriteLine("You have {0} lives!", lives);
}
else
{
Console.WriteLine("You only have {0} life left!!", lives);
}
Console.WriteLine(printProgress);
Console.Write("\n");
Console.Write("Guess a letter: ");
string playerGuess = Console.ReadLine();
bool guessTest = playerGuess.All(Char.IsLetter);
while (guessTest == false || playerGuess.Length != 1)
{
Console.WriteLine("Please enter only a single letter!");
Console.Write("Guess a letter: ");
playerGuess = Console.ReadLine();
guessTest = playerGuess.All(Char.IsLetter);
}
playerGuess = playerGuess.ToUpper();
char playerChar = Convert.ToChar(playerGuess);
if (guessedLetters.Contains(playerChar) == false)
{
guessedLetters[numberStore] = playerChar;
numberStore++;
foreach(char letter in secretArray)
{
counter++;
if (letter == playerChar)
{
printArray[counter] = playerChar;
letterFound = true;
multiples++;
}
}
if (letterFound)
{
Console.WriteLine("Found {0} letter {1}!", multiples, playerChar);
}
else
{
Console.WriteLine("No letter {0}!", playerChar);
lives--;
}
Console.WriteLine((lives));
}
else
{
Console.WriteLine("You already guessed {0}!!", playerChar);
}
}
if (victory)
{
Console.WriteLine("\n\nThe word was: {0}", secretWord);
Console.WriteLine("\n\nYOU WIN!!!!!!!!!!!");
}
else
{
Console.WriteLine("\n\nThe word was: {0}", secretWord);
Console.WriteLine("\n\nYOU LOSE!!!!!!!!!");
}
}
}
Hello! This program is written in C# to play a word guessing game with the user. How would a method to organize the code be used? Like taking some code out of main to put it in a separate method and giving the method a name. Thank you!
![dableCurr..
Run
main.cs x
Console
Shell
using System;
> mcs -out:main.exe main.cs
> mono main.exe
Hi, my name is Stevie. I am thinking of an Animal.
Guess which animal I am thinking of>
You have 5 lives!
using System.Linq;
2
class main
{
public static void Main (string[] args)
4
{
Console.Writeline ("Hi, my name is Stevie. I am thinking of an Animal.\nGuess which
animal I am thinking of> ");
string[] words = new string[7]{"sheep","goat","lion","horse","dog","cat","lizard"};
Random rnd = new Random();
int random = rnd. Next(0, 5);
7
Guess a letter: a
No letter A!
8
4
9
You have 4 lives!
10
string secretword = words[random];
secretword - secretword.TOUpper ();
11
Guess a letter: q
Found 1 letter G!
12
13
int lives - 5;
4
14
int counter = -1;
You have 4 lives!
--G
int wordLength - secretword. Length;
char[] secretArray = secretword.TocharArray ();
char[] printArray = new char[wordLength];
char[] guessedLetters = new char[26];
15
16
Guess a letter: d
17
Found 1 letter D!
18
4
19
int numberStore = 0;
You have 4 lives!
D-G
bool victory - false;
foreach(char letter in printArray)
{
20
21
Guess a letter: o
22
Found 1 letter O!
23
counter++;
4
printArray[counter] = '-';
}
while(lives > 0)
{
24
25
The word was: DOG
26
27
28
counter - -1;
YOU WIN!!!!!!!!!!!
string printProgress = String.Concat (printArray);
29
bool letterFound = false;
int multiples = 0;
if (printProgress == secretword)
{
victory = true;
30
31
32
33
34
35
break;
}
if (lives > 1)
{
Console.Writeline("You have {0} lives!", lives);
}
36
37
38
39
40
41
else
{
Console.Writeline("You only have {0} life left!!", lives);
}
42
43
44](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a85ba4d-6f5c-41bf-97e5-182adb23b838%2Fa281eb4e-1ddf-415e-b782-9e07986c616b%2F8em0fv_processed.png&w=3840&q=75)
Transcribed Image Text:dableCurr..
Run
main.cs x
Console
Shell
using System;
> mcs -out:main.exe main.cs
> mono main.exe
Hi, my name is Stevie. I am thinking of an Animal.
Guess which animal I am thinking of>
You have 5 lives!
using System.Linq;
2
class main
{
public static void Main (string[] args)
4
{
Console.Writeline ("Hi, my name is Stevie. I am thinking of an Animal.\nGuess which
animal I am thinking of> ");
string[] words = new string[7]{"sheep","goat","lion","horse","dog","cat","lizard"};
Random rnd = new Random();
int random = rnd. Next(0, 5);
7
Guess a letter: a
No letter A!
8
4
9
You have 4 lives!
10
string secretword = words[random];
secretword - secretword.TOUpper ();
11
Guess a letter: q
Found 1 letter G!
12
13
int lives - 5;
4
14
int counter = -1;
You have 4 lives!
--G
int wordLength - secretword. Length;
char[] secretArray = secretword.TocharArray ();
char[] printArray = new char[wordLength];
char[] guessedLetters = new char[26];
15
16
Guess a letter: d
17
Found 1 letter D!
18
4
19
int numberStore = 0;
You have 4 lives!
D-G
bool victory - false;
foreach(char letter in printArray)
{
20
21
Guess a letter: o
22
Found 1 letter O!
23
counter++;
4
printArray[counter] = '-';
}
while(lives > 0)
{
24
25
The word was: DOG
26
27
28
counter - -1;
YOU WIN!!!!!!!!!!!
string printProgress = String.Concat (printArray);
29
bool letterFound = false;
int multiples = 0;
if (printProgress == secretword)
{
victory = true;
30
31
32
33
34
35
break;
}
if (lives > 1)
{
Console.Writeline("You have {0} lives!", lives);
}
36
37
38
39
40
41
else
{
Console.Writeline("You only have {0} life left!!", lives);
}
42
43
44
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
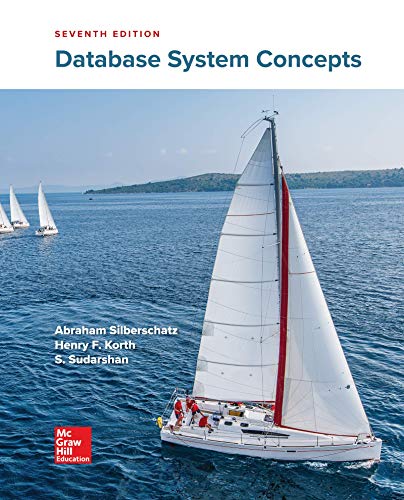
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
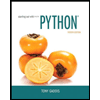
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
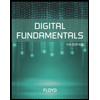
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
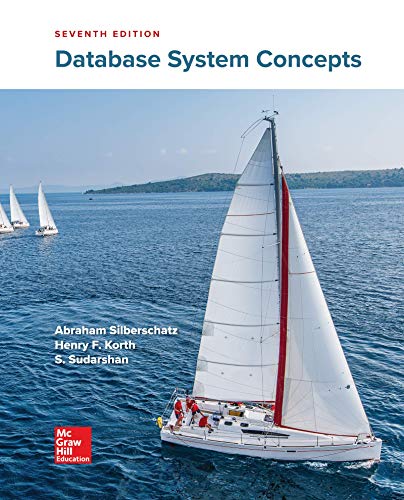
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
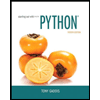
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
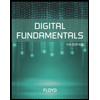
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
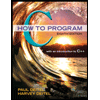
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
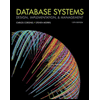
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
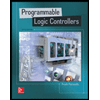
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education