The xxx_Student class:– Name - the name consists of the First and Last name separated by a space.– Student Id – a whole number automatically assigned in the student class– Student id numbers start at 100. The numbers are assigned using a static variable in the Student class• Include all instance variables• Getters and setters for instance variables• A static variable used to assign the student id starting at 100• A toString method which returns a String containing the student name and id in the format below:Student: John Jones ID: 101 The xxx_Course classA Course has the following information (modify your Course class):– A name– An Array of Students which contains an entry for each Student enrolled in the course (allow for up to 10 students)– An integer variable which indicates the number of students currently enrolled in the course. Write the constructor below which does the following:Course (String name)Sets courseName to nameCreates the students array of size 10Sets number of Students to 0 Write the 3 getters+getCourseName() : String+getStudents() : Student []+getNumberOfStudents() : int Write the 2 setterspublic void addStudent (Student student)public void addStudent (String studentName) Write toStringReturn a String that contains the following information concatenated so that the information prints on separate lines as shown in the sample output:Course: xxxxx Number of Students: xxThe students in the class are:Each of the Student objects in the students array followed by a \n so they print on separate lines Write a class xxx_TestCourse which willPrompt the user for the name of a Course and create a Course objectIn a loop, until the user enters a q or a Q,Prompt the user to enter student names or Q to endFor each student entered,create a Student object and add it to the Course using the addStudent method of the Course classAt the end of the loop, print the Course object. Its toString method will format the output as shown on the next slide Sample OutputEnter the course nameCPS2231-04Enter the name of a student or Q to quitJonEnter the name of a student or Q to quitMaryEnter the name of a student or Q to quitTomEnter the name of a student or Q to quitqCourse: CPS2231-04 Number of Students: 3The students in the class are:Student: Jon ID: 100Student: Mary ID: 101Student: Tom ID: 102
The xxx_Student class:
– Name - the name consists of the First and Last name separated by a space.
– Student Id – a whole number automatically assigned in the student class
– Student id numbers start at 100. The numbers are assigned using a static variable in the Student class
• Include all instance variables
• Getters and setters for instance variables
• A static variable used to assign the student id starting at 100
• A toString method which returns a String containing the student name and id in the format below:
Student: John Jones ID: 101
The xxx_Course class
A Course has the following information (modify your Course class):
– A name
– An Array of Students which contains an entry for each Student enrolled in the course (allow for up to 10 students)
– An integer variable which indicates the number of students currently enrolled in the course.
Write the constructor below which does the following:
Course (String name)
Sets courseName to name
Creates the students array of size 10
Sets number of Students to 0
Write the 3 getters
+getCourseName() : String
+getStudents() : Student []
+getNumberOfStudents() : int
Write the 2 setters
public void addStudent (Student student)
public void addStudent (String studentName)
Write toString
Return a String that contains the following information concatenated so that the information prints on separate lines as shown in the sample output:
Course: xxxxx Number of Students: xx
The students in the class are:
Each of the Student objects in the students array followed by a \n so they print on separate lines
Write a class xxx_TestCourse which will
Prompt the user for the name of a Course and create a Course object
In a loop, until the user enters a q or a Q,
Prompt the user to enter student names or Q to end
For each student entered,
create a Student object and add it to the Course using the addStudent method of the Course class
At the end of the loop, print the Course object. Its toString method will format the output as shown on the next slide
Sample Output
Enter the course name
CPS2231-04
Enter the name of a student or Q to quit
Jon
Enter the name of a student or Q to quit
Mary
Enter the name of a student or Q to quit
Tom
Enter the name of a student or Q to quit
q
Course: CPS2231-04 Number of Students: 3
The students in the class are:
Student: Jon ID: 100
Student: Mary ID: 101
Student: Tom ID: 102

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

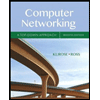
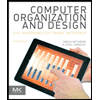
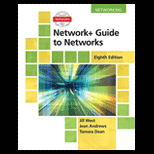
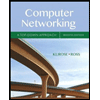
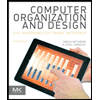
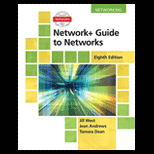
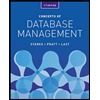
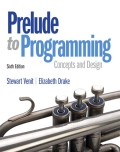
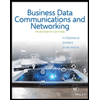