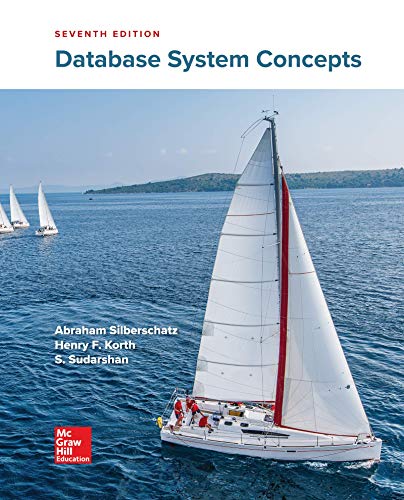
The Schedule class contains several courses. Each Course has a name, start time, and end time. The toString method for a Course should return a string containing the name, start time, and end time. For example, if the course is "Networking I" and it is held from 8am to 8:50am, the toString method for that course should return:
"Networking I: 8am - 8:50am"
The toString method for a Schedule should return "Your Schedule:" followed by all of the courses in the schedule. Below is an example showing what the Schedule's toString method should return if it contained five courses (Networking I,
"Your Schedule
Networking I: 8am - 8:50am
Database: 9am - 9:50am
Web Development: 10am - 10:50am
Java I: 12pm - 12:50pm
Yoga: 1pm - 1:50pm"
Include a Main.java to test your code
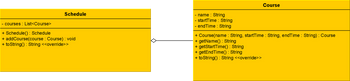

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Hangman gameWrite a terminal based on the game of Hangman. In a hangman game, the player is given a wordthat they need to guess, with each letter of the word represented by an underscore/blank. Theplayer tries to guess a letter of the word by entering it into the terminal. If the letter is correct, theblank corresponding to that letter is filled in. If the letter is incorrect, a part of a stick figure isdrawn. The player has a limited number of incorrect guesses before the stick figure is fully drawnand the game is lost. The player wins the game if they guess all the letters of the word before thestick figure is fully drawn.The program should have an array of possible words to choose from. A different word should berandomly chosen from the array every time we run the program.Others: Do not use GOTO statementsarrow_forwardPendant Publishing edits multi-volume manuscripts for many authors. For each volume, they want a label that contains the author’s name, the title of the work, and a volume number in the form Volume 9 of 9. For example, a set of three volumes requires three labels: Volume 1 of 3, Volume 2 of 3, and Volume 3 of 3. Design an application that reads records that contain an author’s name, the title of the work, and the number of volumes. The application must read the records until eof is encountered and produce enough labels for each work. The flowchart must include a call symbol, at the beginning, to redirect the input to the external data file. create a solution algorithm using pseudocode create a flowchart using RAPTORarrow_forwardAssignment Submission Instructions:This is an individual assignment – no group submissions are allowed. Submit a script file that contains the SELECT statements by assigned date. The outline of the script file lists as follows:/* ******************************************************************************** * Name: YourNameGoesHere * * Class: CST 235 * * Section: * * Date: * * I have not received or given help on this assignment: YourName * ***********************************************************************************/USE RetailDB;####### Tasks: Write SQL Queries ######### -- Task 1 (Customer Information):-- List your SELECT statement below. Make sure the SQL script file can be run successfully in MySQL and show the outcome of the code on MySQLarrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardpython class Student:def __init__(self, first, last, gpa):self.first = first # first nameself.last = last # last nameself.gpa = gpa # grade point average def get_gpa(self):return self.gpa def get_last(self):return self.last def to_string(self):return self.first + ' ' + self.last + ' (GPA: ' + str(self.gpa) + ')' class Course:def __init__(self):self.roster = [] # list of Student objects def drop_student(self, student):# Type your code here def add_student(self, student):self.roster.append(student) def count_students(self):return len(self.roster) if __name__ == "__main__":course = Course() course.add_student(Student('Henry', 'Nguyen', 3.5))course.add_student(Student('Brenda', 'Stern', 2.0))course.add_student(Student('Lynda', 'Robinson', 3.2))course.add_student(Student('Sonya', 'King', 3.9)) print('Course size:', course.count_students(),'students')course.drop_student('Stern')print('Course size after drop:', course.count_students(),'students')arrow_forward// Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forward
- There are a number of national and state parks available to tourists. Create a Park class. Include datamembers such as name of park, location, type of (i.e., national, state, local) facility, fee, number ofemployees, number of visitors recorded for the past 12 months, and annual budget. Write separateinstance methods that a) return a string representing name of the park, the location and type of park;b) return a string representing the name of the park, the location and facilities available; c) computecost per visitor based on annual budget and the number of visitors during the last 12 months; and d)compute revenue from fees for the past year based on number of visitors and fee. Also include aToString( ) method that returns all data members with appropriate labels. Create a second class totest your Park class.arrow_forwardC++ program Write a method that displays information about a movie. The method accepts the movie title, running time in minutes and the release year as arguments. Provide a default value for the running time so that if you call the method without minutes, it defaults to 90. Write a main() method that demonstrates you can call the method with two or three arguments. Save the file as Movie.cpparrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- MadLib Game bigGame.py Write a program that plays a MadLib game with the user. The program should ask the user to enter the following words: A plural noun (PNOUN1) A person’s first name (FNAME) A noun (NOUN1) A person’s last name (LNAME) A second plural noun (PNOUN2) A place (PLACE1) A third plural noun (PNOUN3) Another place (PLACE2) A fourth plural noun (PNOUN4) A second noun (NOUN2) An adjective (ADJECTIVE1) A second adjective (ADJECTIVE2) A verb (VERB) A third adjective (ADJECTIVE3) After the user has entered these items, the program should display the following story, inserting the user’s input into the appropriate locations: Hello there, sports PNOUN1!This is FNAME, talking to you from the press NOUN1in LNAME Stadium, where 57,000 cheering PNOUN2Have gathered to watch (the) PLACE1 PNOUN3take on (the) PLACE2 PNOUN4.Even though the NOUN2 is shining, it’s a/an ADJECTIVE1cold day with the temperature in the ADJECTIVE2 20s.We’ll be back for the opening VERB -off after a few…arrow_forwardThe program must include two classesclGradesGraph and GradesGrapghDemo. GradesGraphDemo (one method)- Main method only GradesGraph- Include at least 4 methods (No credits without at least 4 methods!!!) Create a class that represents the grade distribution for a given course. In this class you should write methods to perform the following tasks: Read the number of each of the letter grades A, B, C D and F Set the number of letter grades A, B, C, D and F Return the total number of grades Return the percentage of each letter grade as a whole number between 0 and 100 inclusive Draw a bar graph of the grade distributionThe graph should have five bars, one per grade. Each bar can be a horizontal row of asterisks, such that the number of asterisks in a row is proportionate to the percentage of grades in each category. For example, let on asterisk represent 2%, so 50 asterisks correspond to 100%. Mark the horizontal axis at 10% increments from 0 to 100% and label each line with a letter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
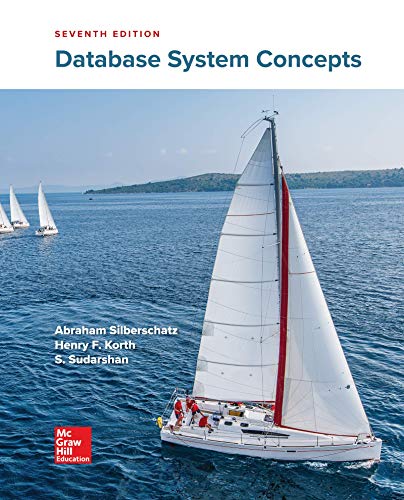
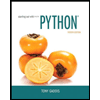
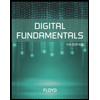
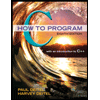
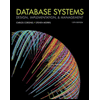
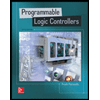