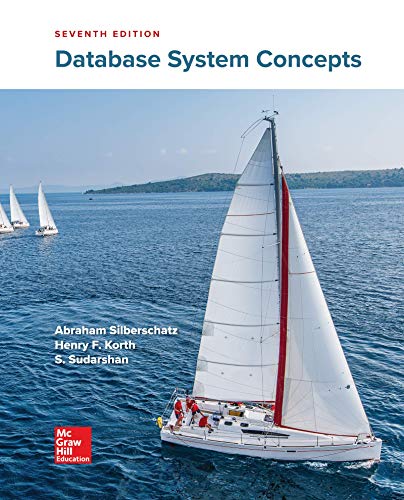
Concept explainers
Test #1
Create a class called Rectangle. Use the UML below to define the methods in the class. Create a folder on the linux server called test1 and write and test your code in that area. An executable program must be on the linux server. Hit submit on the assignment page when you have completed the test.
Use a similar driver as below to test your class.
#include<iostream>
#include"Rectangle.h"
int main(){
Rectangle r1(1.2, 3.4);
cout<<r1.toString()<<endl;
Rectangle r2;
cout<<r2.toString()<<endl;
// Test setters and getters
r1.setLength(5.6);
r1.setWidth(7.8);
cout<<r1.toString()<<endl;
cout<<"length is: " <<r1.getLength()<<endl;
cout<<"width is: "<<r1.getWidth()<<endl;
// Test getArea() and getPerimeter()
cout<<"area is: "<<r1.getArea()<<endl;
cout<<"perimeter is: "<<r1.getPerimeter()<<endl;
}
Output Should be something like the following:
Rectangle[length=1.2,width=3.4]
Rectangle[length=1.0,width=1.0]
Rectangle[length=5.6,width=7.8]
length is: 5.6
width is: 7.8
area is: 43.68
perimeter is: 26.80
![Rectangle
-length: float 1.0f
=
-width: float = 1.0f
+Rectangle()
+Rectangle (length: float, width: float)
+getLength(): float
+setLength (length: float):void
+getWidth(): float
+setWidth (width: float):void
+getArea(): double
+getPerimeter(): double
+toString(): String
"Rectangle[length=?,width=?]"](https://content.bartleby.com/qna-images/question/0fe20004-301e-41c6-8c04-c95d974b1869/dd16621d-1dca-4976-bf52-3b8390af37b2/rhmf7hi_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- This is the question - Create a UsedCarException class that extends Exception; its constructor receives a value for a vehicle identification number (VIN) that is passed to the parent constructor so it can be used in a getMessage() call. Create a UsedCar class with fields for VIN, make, year, mileage, and price. The UsedCar constructor throws a UsedCarException when the VIN is not four digits; when the make is not Ford, Honda, Toyota, Chrysler, or Other; when the year is not between 1997 and 2017 inclusive; or either the mileage or price is negative. Write an application that establishes an array of at least seven UsedCar objects and handles any Exceptions. Display a list of only the UsedCar objects that were constructed successfully. Here is the code I have - public class ThrowUsedCarException { public static void main(String[] args) { // Write your code here } } public class UsedCar { String vin; String make; int year; int mileage; int…arrow_forwardUse the FlightInfo class in the attached FlightInfo.h file for this assignment. Task 1: Modify the FlightInfo.h file to include the following (empty) Exception Classes. Invalid Direction Invalid Fuel Level Invalid Altitude Invalid Speed Modify the setter methods to throw an exception for invalid data. Note that these setters are void. They create exceptions instead of returning a value for invalid data. Task 2: Create a driver program that will instantiate a FlightInfo object. Driver Program steps. Prompt the user for the following inputs values Speed Direction Altitude Fuel Level Call the flightInfo's showAll() method. Catch these exceptions in the driver program and display a message anytime invalid data is given indicating the issue. The valid ranges are commented in the FlightInfo.h file. As usual, work on a small section like Invalid Direction and confirm that works as expected. Once that is good, the rest are very similar. 6 point Bonus. Create an additional Exception…arrow_forwardThe Mid-Term Exam: A program to write (below). Note that you must submit and return directly to me with your name and the date included as an upload Canvas (file) submission. Make sure that your name is used as the file name. (You will not get credit if your exam cannot be read.) No notes, books, cells, Internet Search, computer/device, etc., may be used during this exam. Write a Java program that lets the user enter a series of integers with an input range from 2 to 199 (in random order). The user must enter a value of -1, 0, or +1 (a sentinel which must be an integer and not part of the series of numbers) in order to signal the end of the series. • After all the numbers have been entered, the program should display the smallest and largest numbers entered, and not include the sentinel. Note that this must be a Java implementatiọn, and not pseudo-code, etc. You must use a Loop and If-Else constructs as well.arrow_forward
- I am looking for the following in your program: Python GUI-based MP3 Player Project #3: Python GUI-based MP3 Player 1. Ability to select a folder / directory for MP3s. (HINT: There’s a function/method in filedialog of tkinter to do this. In other words, you'll need the following import: from tkinter import messagebox, filedialog 2. Ability to play selected MP3s. 3. Ability to pause the current MP3. 4. Quit button/function to exit application. 5. Use a list to store and display the mp3 file names. You will need to demonstrate your mastery of lists, loops, and GUI elements within this program. Submission will include: 1. Python program named myplayer.py 2. Data Flow Diagrams / Pseudocode 3. If choosing an alternate library to tkinter or pygame, provide instructions and download links for the alternate library.arrow_forwardChallenge 2: InvalidNumbers.java Write a program InvalidNumbers that asks the user to enter a positive integer. The program will warn the user if a valid integer is not entered using the InputMismatchException and ask to enter again. The program should also throw an exception if the user enters negative numbers. Write the program and test it using negative numbers, doubles, and very large numbers.arrow_forwardCompulsory Task 2 Create a file named method_override.py and follow the instructions below: Take user inputs that ask for the name, age, hair colour, and eye colour of a person. Create an adult class with the following attributes and method: name, age, eye_colour, and hair_colour Method called can_drive() that prints the name of the person and that they are old enough to drive. Create a subclass of the adult class named “Child" that has the same attributes, but overrides the can_drive method to print the persons name and that they are too young to drive. Create some logic that determines if the person is 18 or older and create an instance of the Adult class if this is true. Otherwise, create an instance of the Child class. Once the object has been created, call the can_drive() method to print out whether the person is old enough to drive or not. O Ask a SE L1T29 - Introduction to OOP II - Inheritance.pdf 212% 8 of 9 C 1 of 2 work! > xarrow_forward
- JAVA PROGRAM ASAP The program does not run in hypergrade can you please MODIFY THIS program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. I have provided the correct test cases as a screenshot as well as the failed test cases. It says 0 out of 4 passed. The program must pass the test case when uploaded to Hypergrade. Files data are down below: text1.txt StopAndSmellTheRoses. text2.txt ATrueRebelYouAre!EveryoneWasImpressed.You'llDoWellToContinueInTheSameSpirit.PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.Scanner;class Main { // Driver code public static void main(String[] args) { // scanner object used to take user input Scanner sc = new Scanner(System.in); // loop iterates until user enters "quit" or "QUIT" while (true) { System.out.print("Please enter…arrow_forwardDevelop an exception class named InvalidMonthException that extends the Exception class. Instances of the class will be thrown based on the following conditions: The value for a month number is not between 1 and 12 The value for a month name is not January, February, March, … December Develop a class named Month. The class should define an integer field named monthNumber that holds the number of a month. For example, January would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-argument constructor that sets the monthNumberto 1. An overloaded constructor that accepts the number of the month as an argument. The constructor should set the monthNumberfield to the parameter value if the parameter contains the value 1 – 12. Otherwise, throw an InvalidMonthException exception back to the caller. The exception should note that the month number was incorrect. An overloaded constructor that accepts a string containing the name of the month,…arrow_forwardA thrown exception may contain more information about what caused the exception. True or Falsearrow_forward
- Define an exception class called tornadoException. The class should have two constructors including the default constructor. If the exception is thrown with the default constructor, the method what should return "Tornado: Take cover immediately!". The other constructor has a single parameter, say m, of the int type. If the exception is thrown with this constructor, the method what should return "Tornado: m miles away; and approaching!"arrow_forwardWhen JUnit testing, which is the best practice? Group of answer choices: 1. When the method or constructor takes a numeric value, generate many random values for those values and test if all of them work. 2. You only need to test three possibilities, positive values, negative values, and 0. 3. Convert all numbers to String, and always compare those instead of the numbers themselves. 4. Never test an input that will throw an exception, because you should never need to pass an invalid input to a method or constructor.arrow_forwardCreate a new Python file in your project named FullName.py. In this module, create a class named FullName. When creating a FullName object, the client will pass a string for first name and a string for last name, which the constructor will assign to two attributes. Your class should have the following methods: __init__, __str__, and __gt__. __gt__ will compare two FullName objects using the following logic: If person A’s last name comes after person B’s last name alphabetically, person A is “greater than” person B. If their last names are equal, check their first names to see which is greater. Hint: The > and < operators are already overloaded for string objects, so your __gt__ method should be relatively simple. In your main.py file, demonstrate creating a few FullName objects and calling these methods on them.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
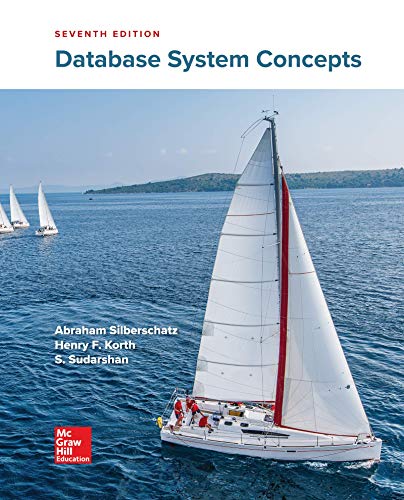
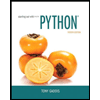
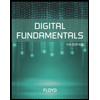
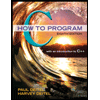
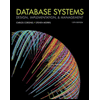
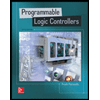