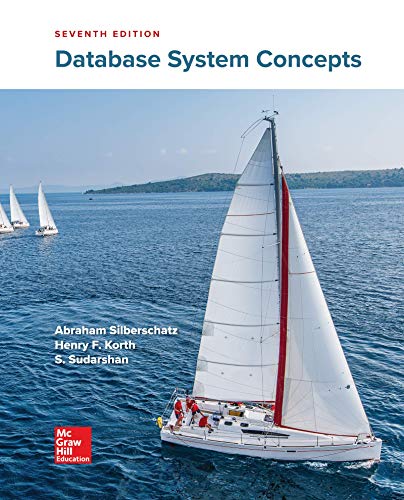
Java
Write a RandomNumberGuesser game that extends from the NumberGuesser Game.
your program should have : main.java, NumberGuesser.java and a RandomNumberGuesser.java that is an extended class of NumberGuesser.
public class RandomNumberGuesser extends NumberGuesser {
// your code here
}
Somewhere in the code in main.java should look something like this:
NumberGuesser guesser = new RandomNumberGuesser(1, 100);

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

Here is another feature we are asked to add to Number Guessing game. A "Guess History". When the program guesses the correct number, it should show the guesses it made along the way.
We'll return to our command line game. Here is a sample run with the new feature:
Guess a number from 1 to 100.
Is it 27? (h/l/c): h
Is it 86? (h/l/c): l
Is it 68? (h/l/c): l
Is it 59? (h/l/c): l
Is it 40? (h/l/c): c
Here are the guesses: 27 86 68 59 40
Great! Do you want to play again? (y/n): y
Guess a number from 1 to 100.
Is it 44? (h/l/c): l
Is it 27? (h/l/c): c
Here are the guesses: 44 27
Great! Do you want to play again? (y/n): n
The last lines of each game are outcome of new added feauture.
You can add the feature by adding a method to NumberGuesser that returns the guesses using this data type: ArrayList<Integer>
The signature should look like this:
public ArrayList<Integer> getGuessHistory()
The method will be inherited by RandomNumberGuesser. Make sure that it works in both classes. Once it is working you will be able to print out the guess history like this:
System.out.print("Here are the guesses: ");
ArrayList<Integer> guesses = guesser.getGuessHistory();
for (Integer g: guesses) {
System.out.print(g + " ");
}
System.out.println();
HINT:
You can add a member field to NumberGeusser that will hold the ArrayList:
private ArrayList<Integer> guessHistory;
You should initialize that member field in the constructor, and re-initialize it in the reset() method.
Your getGuessHistory method should return a copy of that member field. You can make a copy using the ArrayList constructor:
return new ArrayList<Integer>(this.guessHistory);
You could add a protected helper method to NumberGuesser that adds a guess into that array list:
protected void addGuess(int guess) {
this.guessHistory.add(guess);
}
That could be called where it is that you want to add the guesses to the history. Make sure not to add duplicates.
We are asked to add an exception to the game. Please see below.
For this part of the assignment you can start by creating your own exception in a file named NumberGuesserIllegalStateException.java, with this code:
public class NumberGuesserIllegalStateException extends Exception {
public NumberGuesserIllegalStateException(String errorMessage) {
super(errorMessage);
}
}
Next modify your code so that the higher and lower methods of both the NumberGuesser and RandomNumberGuesser should throw the exception if there are no more remaining numbers to guess. You might be able to achieve this by adding the logic to your NumberGuesser and letting RandomNumberGuesser inherit the behavior. Or you might need to add the logic to both classes. It will depend on your implementation.
Finally add a try-catch block to your number guessing game so that the user is notified if the user cheats.
Here is another feature we are asked to add to Number Guessing game. A "Guess History". When the program guesses the correct number, it should show the guesses it made along the way.
We'll return to our command line game. Here is a sample run with the new feature:
Guess a number from 1 to 100.
Is it 27? (h/l/c): h
Is it 86? (h/l/c): l
Is it 68? (h/l/c): l
Is it 59? (h/l/c): l
Is it 40? (h/l/c): c
Here are the guesses: 27 86 68 59 40
Great! Do you want to play again? (y/n): y
Guess a number from 1 to 100.
Is it 44? (h/l/c): l
Is it 27? (h/l/c): c
Here are the guesses: 44 27
Great! Do you want to play again? (y/n): n
The last lines of each game are outcome of new added feauture.
You can add the feature by adding a method to NumberGuesser that returns the guesses using this data type: ArrayList<Integer>
The signature should look like this:
public ArrayList<Integer> getGuessHistory()
The method will be inherited by RandomNumberGuesser. Make sure that it works in both classes. Once it is working you will be able to print out the guess history like this:
System.out.print("Here are the guesses: ");
ArrayList<Integer> guesses = guesser.getGuessHistory();
for (Integer g: guesses) {
System.out.print(g + " ");
}
System.out.println();
HINT:
You can add a member field to NumberGeusser that will hold the ArrayList:
private ArrayList<Integer> guessHistory;
You should initialize that member field in the constructor, and re-initialize it in the reset() method.
Your getGuessHistory method should return a copy of that member field. You can make a copy using the ArrayList constructor:
return new ArrayList<Integer>(this.guessHistory);
You could add a protected helper method to NumberGuesser that adds a guess into that array list:
protected void addGuess(int guess) {
this.guessHistory.add(guess);
}
That could be called where it is that you want to add the guesses to the history. Make sure not to add duplicates.
We are asked to add an exception to the game. Please see below.
For this part of the assignment you can start by creating your own exception in a file named NumberGuesserIllegalStateException.java, with this code:
public class NumberGuesserIllegalStateException extends Exception {
public NumberGuesserIllegalStateException(String errorMessage) {
super(errorMessage);
}
}
Next modify your code so that the higher and lower methods of both the NumberGuesser and RandomNumberGuesser should throw the exception if there are no more remaining numbers to guess. You might be able to achieve this by adding the logic to your NumberGuesser and letting RandomNumberGuesser inherit the behavior. Or you might need to add the logic to both classes. It will depend on your implementation.
Finally add a try-catch block to your number guessing game so that the user is notified if the user cheats.
- Write a second constructor as indicated. Sample output:User1: Minutes: 0, Messages: 0 User2: Minutes: 1000, Messages: 5000 // ===== Code from file PhonePlan.java =====public class PhonePlan { private int freeMinutes; private int freeMessages; public PhonePlan() { freeMinutes = 0; freeMessages = 0; } // FIXME: Create a second constructor with numMinutes and numMessages parameters. /* Your solution goes here */ public void print() { System.out.println("Minutes: " + freeMinutes + ", Messages: " + freeMessages); }}// ===== end ===== // ===== Code from file CallPhonePlan.java =====public class CallPhonePlan { public static void main(String [] args) { PhonePlan user1Plan = new PhonePlan(); // Calls default constructor PhonePlan user2Plan = new PhonePlan(1000, 5000); // Calls newly-created constructor System.out.print("User1: "); user1Plan.print(); System.out.print("User2: "); user2Plan.print(); }}// ===== end =====arrow_forwardPlease answer question. This is pertaining to Java programming language 3-20arrow_forwardIn Java code: Write the class encapsulating the concept of money, assuming that money has the following attributes: dollars, cents In addition to the constructors, the accessors and mutators, write the following methods: public Money() public Money(int dollars, int cents) public Money add(Money m) public Money substract(Money m) public Money multiply(int m) public static Money[] multiply(Money[] moneys, int amt) public boolean equals(Money money) public String toString() private void normalize() // normalize dollars and cents field Add additional helper methods if necessary. Use the following test driver program to test your Money class: public class MoneyTester{public static void main(String[] args){Money m1 = new Money(8, 75); // set dollars to 8 and cents to 75Money m2 = new Money(5, 80); // set dollars to 5 and cents to 80 MoneyMoney m3 = new Money(); // initialize dollars to 0 and cents to 0System.out.println("\tJane Doe " + "CIS35A Spring 2021 Lab 4"); // useyour…arrow_forward
- Write a code for the following using Console.WriteLine.arrow_forwardI got an error message that says the grid item is not abstarct. Error message: /GridItem.java:2: error: GridItem is not abstract and does not override abstract method containsPoint(int,int) in GridItempublic class GridItem { ^./Square.java:12: error: cannot find symbol return xValue >= x && ^ symbol: variable xValue location: class Square./Square.java:13: error: cannot find symbol xValue <= x + side && ^ symbol: variable xValue location: class Square ALSO THE GRIDWRITER CLASS YOU WROTE IS DIFFERENT FROM THE ONE GIVEN FOR THE HOMEWORK. PLEASE SEE BELOW public class GridWriter { private GridItem items[]; private int size; private int rows; private int columns; private static final int INITIAL_CAPACITY = 4; /**** * Create a new GridWriter. It is initially empty. It has the capacity * to store four GridItems before it will need to double its array size. * The row and column arguments are used in the display…arrow_forwardin Javaarrow_forward
- public class utils { * Modify the method below. The method below, myMethod, will be called from a testing function in VPL. * write one line of Java code inside the method that adds one string * to another. It will look something like this: * Assume that 1. String theInput is 2. string mystring is ceorge неllo, пу nane is * we want to update mystring so that it is неllo, пу nane is Ceorge */ public static string mymethod(string theInput){ System.out.println("This method combines two strings."); Systen.out.println("we combined two strings to make mystring); return mystring;arrow_forwardIn C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forwardPlease use java only. In this assignment, you will implement a simple game in a class called SimpleGame. This game has 2 options for the user playing. Based on user input, the user can choose to either convert time, from seconds to hours, minutes, and seconds, or calculate the sum of all digits in an integer. At the beginning of the game, the user will be prompted to input either 1 or 2, to indicate which option of the game they want to play. 1 will indicate converting time, and 2 will indicate calculating the sum of digits in an integer. For converting time, the user will be prompted to input a number of seconds (as an int) and the program will call a method that will convert the seconds to time, in the format hours:minutes:seconds, and print the result. For example, if the user enters 6734, the program will print the time, 1:52:14. As another example, if the user enters 10,000, the program should print 2:46:39. For calculating the sum of digits in an integer, the user will be…arrow_forward
- Can you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forwardHi, can i get assistance with this book class in Java please? The comments with the "TODO" is what I need assistance with, thank you! The author class is attached to the question in case there is any relevance for it. public class Book {private String title;private double averageRating;private String ISBN;private int numPages;// TODO: insert an appropriate collection for associating Authors and initialise within constructors public Book(){} public Book(String title, double averageRating, String isbn, int numPages){ this.title = title;this.averageRating = averageRating;this.ISBN = isbn;this.numPages = numPages;}//accessorspublic String getTitle(){return title;}public double getAverageRating(){return averageRating;}public String getISBN(){return ISBN;}public int getNumPages(){return numPages;}// TODO: provide an accessor for the book collection public String getAuthorList(){String authorList ="";// TODO: insert code to return all author names separated by commas if multiple authorsreturn…arrow_forwardJAVA PROGRAM Lab #1 Enhancements:1. For the maximum and minimum rainfall amount, also display the month where that happened. For example:a. Maximum rainfall: January, 88.2 inchesb. Minimum rainfall: July, 12.3 inches2. Format all numbers with 1 decimal pointsMain class name: RainFall2 (no package name) HERE IS A WORKING CODE, PLEASE MODIFY THIS CODE SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL THE TEST CASSES. IT HAS TO PASS ALL THE TEST CASSES BECAUSE RIGHT KNOW IT DOES NOT PASS THE TEST CASES. THANK YOU import java.util.*;public class Main{ public static void main(String[] args) { String maxRainfallMonth = "",minRainfallMonth = ""; double maxRainfall,minRainfall,rainFall,avg,tot=0; Scanner sc = new Scanner(System.in); System.out.println("Enter the rainfall for month 1: "); double rainfall = sc.nextDouble(); maxRainfall = rainfall; minRainfall = rainfall; tot = rainfall; String[] months =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
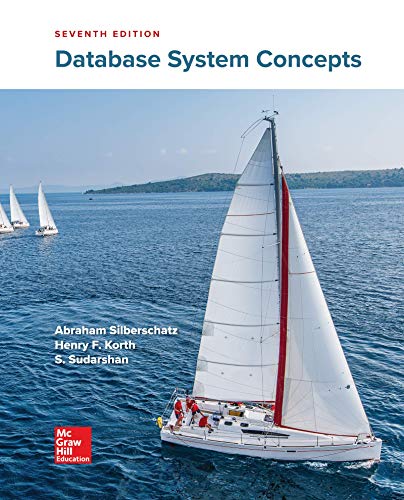
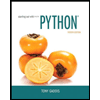
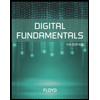
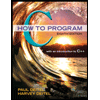
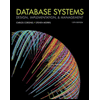
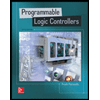