Suppose you are tasked with implementing a reverse queue in which elements are enqueued at the front and dequeued at the rear The implementatio is dynamic, and your queue class has Node pointers called front and rear, which point to the front and rear nodes in the queue respectively The Node struct is given below: struct Node { char element; Node * next; Implement the dequeue function of the reverse queue which should return the dequeued value. The function prototype is T dequeue ()
Suppose you are tasked with implementing a reverse queue in which elements are enqueued at the front and dequeued at the rear The implementatio is dynamic, and your queue class has Node pointers called front and rear, which point to the front and rear nodes in the queue respectively The Node struct is given below: struct Node { char element; Node * next; Implement the dequeue function of the reverse queue which should return the dequeued value. The function prototype is T dequeue ()
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Suppose you are tasked with implementing a reverse queue in which elements are enqueued at the front and dequeued at the rear The umplementation
is dynamic, and your queue class has Node pointers called front and rear, which point to the front and rear nodes in the queue respectively. The
Node struct is given below:
struct Node
char element;
Node * next ;
};
Implement the dequeue function of the reverse queue which should return the dequeued value. The function prototype is T dequeue ()
For the toolbar
(PCLor ALT+FN+F10 (Mac).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
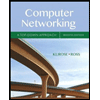
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
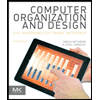
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
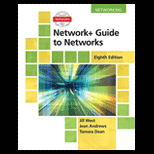
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
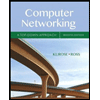
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
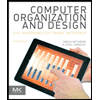
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
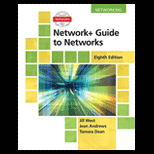
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
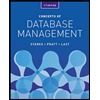
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
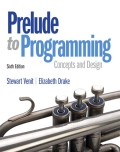
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
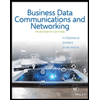
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY