tackQueuePostfix A. Pointer_based queue a. Define the class PoiQueue with no implementation; i.e. declare the data members, and the function members only (Enqueue, Dequeue, IsEmpty, GetHead etc.). b. Implement the Enqueue method of the above class B. Array_based non-circular queue: a. Define the class Queue using one dimensional array representation with no implementation; i.e. declare the data members, and the function members only (Enqueue, Dequeue, IsEmpty, GetHead etc.). b. Implement the Denqueue method of the above class
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
StackQueuePostfix
A. Pointer_based queue
a. Define the class PoiQueue with no implementation; i.e. declare the data
members, and the function members only (Enqueue, Dequeue, IsEmpty,
GetHead etc.).
b. Implement the Enqueue method of the above class
B. Array_based non-circular queue:
a. Define the class Queue using one dimensional array representation with no
implementation; i.e. declare the data members, and the function members
only (Enqueue, Dequeue, IsEmpty, GetHead etc.).
b. Implement the Denqueue method of the above class

Step by step
Solved in 8 steps with 4 images

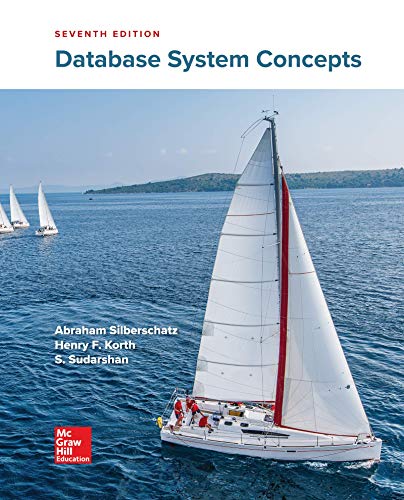
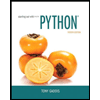
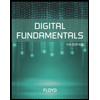
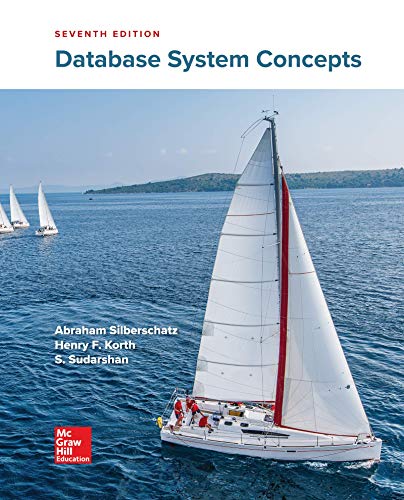
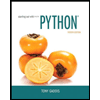
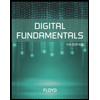
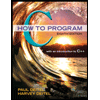
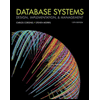
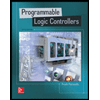