Write a class named Car that has the following fields: The yearModel field is an int that holds the car’s year model. The make field references a String object that holds the make of the car. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods. The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Constructor: The constructor should accept no arguments. Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed. Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and speed fields. The accelerate method should add 5 to the speed field each time it is called. The brake method should subtract 5 from the speed field each time it is called. Prompt the user to enter year and make of the car. Demonstrate the class in a program that creates a Car object, and then calls the accelerate method five times using a loop. After each call to the accelerate method, get the current speed of the car and display it. Then call the brake method five times using a loop. After each call to the brake method, get the current speed of the car and display it.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
java
Assignment Description:
Write a class named Car that has the following fields:
- The yearModel field is an int that holds the car’s year model.
- The make field references a String object that holds the make of the car.
- The speed field is an int that holds the car’s current speed.
In addition, the class should have the following constructor and other methods.
- The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field.
- Constructor: The constructor should accept no arguments.
- Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed.
- Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields.
- Appropriate accessor methods should get the values stored in an object’s yearModel, make, and speed fields.
- The accelerate method should add 5 to the speed field each time it is called.
- The brake method should subtract 5 from the speed field each time it is called.
Prompt the user to enter year and make of the car.
Demonstrate the class in a program that creates a Car object, and then calls the accelerate method five times using a loop. After each call to the accelerate method, get the current speed of the car and display it. Then call the brake method five times using a loop. After each call to the brake method, get the current speed of the car and display it.
Sample output:
Current status of the car:
Year model: 2004
Make: Porsche
Speed: 0
Accelerating...
Now the speed is 5
Now the speed is 10
Now the speed is 15
Now the speed is 20
Now the speed is 25
Braking...
Now the speed is 20
now the speed is 15
Now the speed is 10
Now the speed is 5
Now the speed is 0
Additional Notes/Requirements:
- Always use variables instead of hardcoded values.
- Add comments.
- Format the program properly e.g. indentation, brackets line up, etc. similar to the book examples.
- Instance variables should be declared as private

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

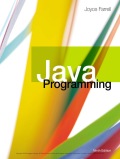
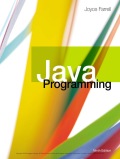