Question 1 You are given a list of characters in Harry Potter. Imagine you are Minerva McGonagall, and you need to pick the students from your own house, which is Gryffindor, from the list. To do so ...
python:
def character_gryffindor(character_list):
"""
Question 1
You are given a list of characters in Harry Potter.
Imagine you are Minerva McGonagall, and you need to pick the students from your
own house, which is Gryffindor, from the list.
To do so ...
- THIS MUST BE DONE IN ONE LINE
- First, remove the duplicate names in the list provided
- Then, remove the students that are not in Gryffindor
- Finally, sort the list of students by their first name
- Don't forget to return the resulting list of names!
Args:
character_list (list)
Returns:
list
>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter,
Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "Luna
Lovegood, Ravenclaw"])
['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']
>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger,
Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "James
Potter, Gryffindor"])
['Hermione Granger, Gryffindor', 'James Potter, Gryffindor', 'Sirius Black,
Gryffindor']
"""
print(character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter,
Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "Luna
Lovegood, Ravenclaw"]))
print(character_gryffindor(["Hermione Granger, Gryffindor", "Hermione
Granger, Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor",
"James Potter, Gryffindor"]))

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

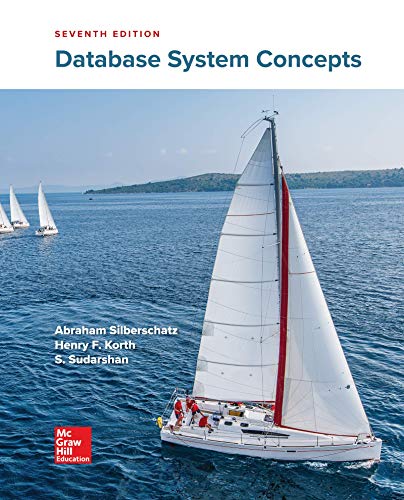
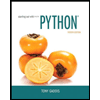
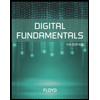
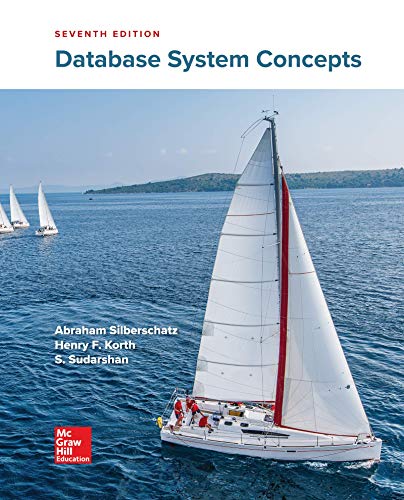
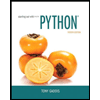
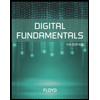
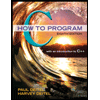
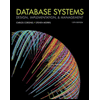
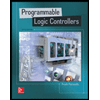