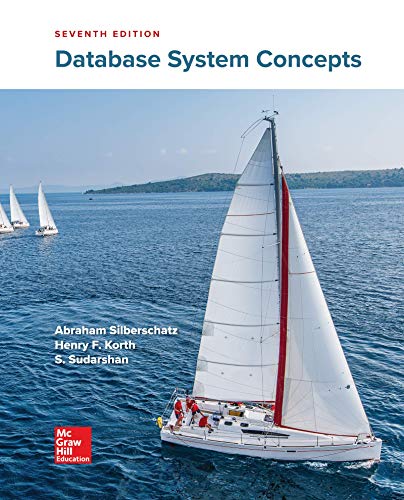
Write code in C++, C# or Python to solve the following problem:
In a party there are N persons and we have M raffles. One person may participate in any number of raffles (from 0 to M). At the end of the day, host of the party comes to you and gives you M lists. Each list contains multiple integer numbers which are the "raffle ID numbers" of the participants. Then, for each list an integer number, I, is announced to determine the winner of the raffle: the winner is the person with raffle ID number K such that K is larger than exactly I numbers in that list. To help the host to find the winners you want to write a program.
Input Format
First line of the input is N and M. Then, there are M lines, each one contains integer numbers between 1 and N. Each list may contain at most N numbers. At the end of each line there is -1. After these lines there is one line which contains M integers to announce the winners. If the j-th number in this line is I it means that the winner of list j is the person whose ID is exactly larger than I other persons in that list. If I is larger than number of people in list j, then that list does not have a winner.
Comment: there are 8 people in this party and we have 3 raffles. In the first raffle, participants' IDs are 1, 4, 2, and 3. In the second raffle, IDs are 5, 2, ... Winner of the first raffle is 3 (3 is exactly larger than 2 other numbers in that list). Winner of the second raffle is 7 (7 is larger than 1, 2, 3, 5, and 6 in the second raffle). Third raffle does not have a winner (there are only 5 participants but the winner's ID has to be larger than 8 other IDs, which is impossible).
Constraints
NA
Output Format
For each list print the ID of the winner. If there is no winner, print -1.
Example: For the example in the Input part we will have: 3 7 -1
Sample Input 0
Sample Output 0

ANSWER:-
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Write in JAVA When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This adjustment can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain fewer than 20 floating-point values. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:System.out.printf("%.2f", yourValue);arrow_forwardI really. need help with this problem please. A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes in word pairs that consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should output the phone number associated with that name. Assume the search name is always in the list. Ex: If the input is: Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank the output is: 867-5309arrow_forwardIn python, Problem Description:You are hosting a party and do not have room to invite all of your friends. You use the following unemotional mathematical method to determine which friends to invite. Number your friends 1, 2, . . . , K and place them in a list in this order. Then perform m rounds. In each round, use a number to determine which friends to remove from the ordered list. The rounds will use numbers r1, r2, . . . , rm. In round i remove all the remaining people in positions that are multiples of ri (that is, ri, 2ri, 3ri, . . .) The beginning of the list is position 1. Output the numbers of the friends that remain after this removal process. Input Specification:The first line of input contains the integer K (1 ≤ K ≤ 100). The second line of input contains the integer m (1 ≤ m ≤ 10), which is the number of rounds of removal. The next m lines each contain one integer. The ith of these lines (1 ≤ i ≤ m) contains ri ( 2 ≤ ri ≤ 100) indicating that every person at a position…arrow_forward
- kindly provide me solution to the python program.arrow_forwardin python Integer num_samples is read from input, representing the number of data samples to be read from input. List data_list contains the data samples read from the remaining input. For each element in data_list: If the element is greater than 25, output the element followed by ' at index ', the element's index in the list, and ' is flagged'. Otherwise, output the element followed by ' at index ', the element's index in the list, and ' is normal'.arrow_forwardIn pythonWrite a function called compter_les_votes that accepts a list of ballots as input (of arbitrary length) and produces a report as a dictionary. The ballots have the form of a couple (valid, condidat) where candidate is the name of one of the candidates in the election, and valid is a boolean that indicates whether or not the ballot is valid. For example, for the following list of bulletins: [(True, 'Pierre'), (False, 'Jean'), (True, 'Pierre'), (True, 'Jacques')]Your function must return the following dictionary: { "number of ballots": 4, "invalid ballots": 1, "results": { "Stone": 2, "Jacques": 1 }}Note that you do not have to display the dictionary, only return it.arrow_forward
- The function sum_evens in python takes a list of integers and returns the sum of all the even integers in the list. For example: Test Result print(sum_evens([1, 5, 2, 5, 3, 5, 4])) 6 print(sum_evens([5, 5, -5, -5])) 0 print(sum_evens([16, 24, 30])) 70arrow_forwardUsing c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forwardThe function should be written in python language. Write a function called "zero_triples" to return all triples in a list of integers that sum to zero. You can assume the list won't contain any duplicates, and a triple should not use the same number more than once. [In]: zero_triples([1, 2, 4]) [Out]: [] [In]: zero_triples([-3, 1, 4, 2]) [Out]: [[1, 2, -3]] [In]: zero_triples([-9, 1, -3, 2, 4, 5, -4, -1]) [Out]: [[-9, 4, 5], [1, -3, 2], [-3, 4, -1], [5, -4, -1]]arrow_forward
- Solve in C++ using the given code and avoid using vectorsarrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forwardrite a function numPairs that accepts two arguments, a target number and a list of numbers. The function then returns the count of pairs of numbers from the list that sum to the target number. In the first example the answer is 2 because the pairs (0,3) and (1,2) both sum to 3. The pair can be two of the same number, e.g. (2,2) but only if the two 2’s are separate twos in the list.In the last example below, there are three 2’s, so there are three different pairs (2,2) so there are 5 pairs total that sum to 4. Sample usage: >> numPairs( 3, [0,1,2,3] )2arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
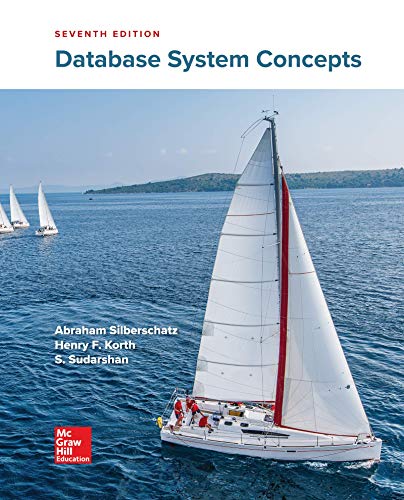
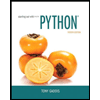
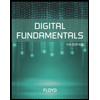
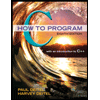
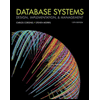
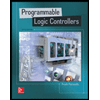