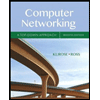
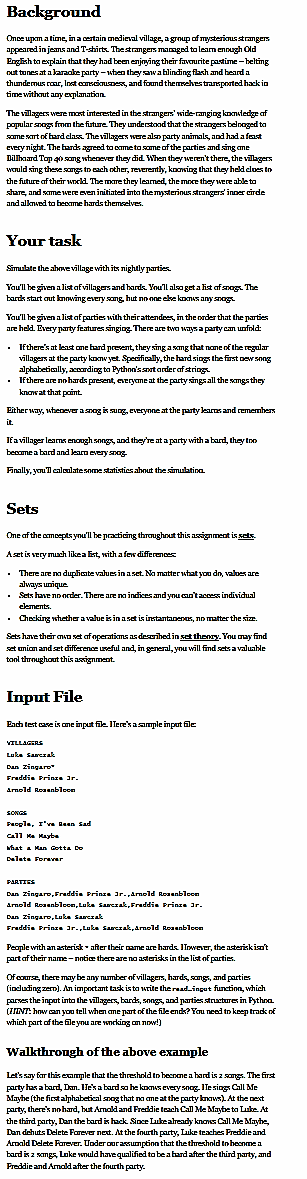
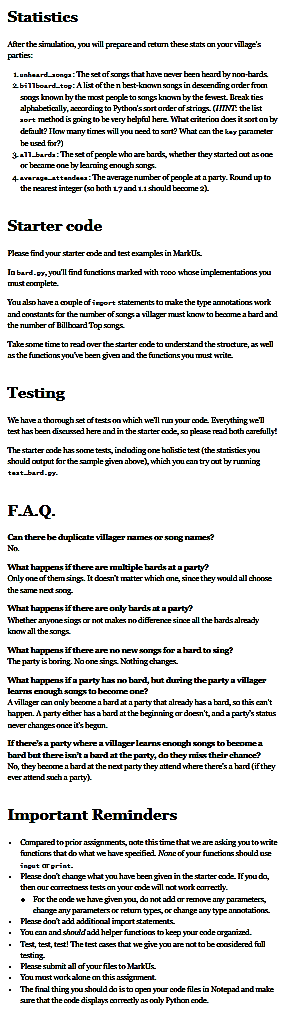

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

A BARD DAY'S NIGHT
a.k.a. BARD ROCK CAFE
a.k.a. THE SCHOOL OF BARD KNOCKS
a.k.a. A BARD RAIN'S A-GONNA FALL
"""
# Imports
from typing import Optional, TextIO # Specific annotations
from math import ceil # For stats
# Constants
# Minimum number of songs for a villager to be promoted to a bard
BARD_THRESHOLD = 10
# Number of songs for the billboard_top statistic
BILLBOARD_N = 10
# DO NOT use these as variables in your code;
# they are only for type contracts.
# Maps from {name: songs that this villager knows}
villagers_type = dict[str, set[str]]
bards_type = set[str]
# Maps from {song name: names of people, including bards, that know this song}
songs_type = dict[str, set[str]]
# A list of parties; each party is a set of the attendee names
parties_type = list[set[str]]
def read_input(
f: TextIO,
) -> tuple[villagers_type, bards_type, songs_type, parties_type]:
"""
Read the given file and return the villagers, bards, songs, and parties.
f is an open file containing VILLAGERS and bards, SONGS, and PARTIES,
in that order. One villager or bard per line;
one song per line; one party per line, consisting of attendees
separated by commas. The parties are given in the order they're held.
"""
# TODO
pass
# Party functions
# We highly recommend adding helper functions here!
def sing_at_party(
villagers: villagers_type, bards: bards_type, songs: songs_type, party: set[str]
) -> None:
"""
A bard sings if present, otherwise the villagers sing.
"""
# TODO
pass
def update_bards_after_party(
villagers: villagers_type, bards: bards_type, songs: songs_type, party: set[str]
) -> None:
"""
Promote attendees who have learned enough songs to bards,
iff there is another bard present at the party.
"""
# TODO
pass
# Stats functions
def unheard_songs(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> set[str]:
"""
Return a set of songs that have never been heard by non-bards.
(This means that only the bards know it.)
"""
# TODO
pass
def billboard_top(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> list[str]:
"""
Return a list of the BILLBOARD_N most popular songs by number of people
who know them, in descending order. Break ties alphabetically.
"""
# TODO
pass
def all_bards(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> set[str]:
"""Return the set of the village's bards."""
# TODO
pass
def average_attendees(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> int:
"""
Return the average number of attendees at parties in the village.
Round up to the nearest integer.
"""
# TODO
pass
# Main process
def run(filename: str) -> dict[str, object]:
"""
Run the program: read the input, host the parties,
and return a dictionary of resulting statistics keyed by name:
unheard_songs, billboard_top, all_bards, average_attendees
filename is the name of an input file.
"""
# TODO
pass
# Run program
if __name__ == "__main__":
# Sample input from the handout -- you can tweak this if you like
stats_handout = run("handout_example.txt")
print("Results of handout sample input")
for key, value in stats_handout.items():
print(f"{key}: {value}")
print()
# Sample bigger input -- you can tweak this if you like
stats_bigger = run("bigger_example.txt")
print("Results of bigger sample input")
for key, value in stats_bigger.items():
print(f"{key}: {value}")
A BARD DAY'S NIGHT
a.k.a. BARD ROCK CAFE
a.k.a. THE SCHOOL OF BARD KNOCKS
a.k.a. A BARD RAIN'S A-GONNA FALL
"""
# Imports
from typing import Optional, TextIO # Specific annotations
from math import ceil # For stats
# Constants
# Minimum number of songs for a villager to be promoted to a bard
BARD_THRESHOLD = 10
# Number of songs for the billboard_top statistic
BILLBOARD_N = 10
# DO NOT use these as variables in your code;
# they are only for type contracts.
# Maps from {name: songs that this villager knows}
villagers_type = dict[str, set[str]]
bards_type = set[str]
# Maps from {song name: names of people, including bards, that know this song}
songs_type = dict[str, set[str]]
# A list of parties; each party is a set of the attendee names
parties_type = list[set[str]]
def read_input(
f: TextIO,
) -> tuple[villagers_type, bards_type, songs_type, parties_type]:
"""
Read the given file and return the villagers, bards, songs, and parties.
f is an open file containing VILLAGERS and bards, SONGS, and PARTIES,
in that order. One villager or bard per line;
one song per line; one party per line, consisting of attendees
separated by commas. The parties are given in the order they're held.
"""
# TODO
pass
# Party functions
# We highly recommend adding helper functions here!
def sing_at_party(
villagers: villagers_type, bards: bards_type, songs: songs_type, party: set[str]
) -> None:
"""
A bard sings if present, otherwise the villagers sing.
"""
# TODO
pass
def update_bards_after_party(
villagers: villagers_type, bards: bards_type, songs: songs_type, party: set[str]
) -> None:
"""
Promote attendees who have learned enough songs to bards,
iff there is another bard present at the party.
"""
# TODO
pass
# Stats functions
def unheard_songs(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> set[str]:
"""
Return a set of songs that have never been heard by non-bards.
(This means that only the bards know it.)
"""
# TODO
pass
def billboard_top(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> list[str]:
"""
Return a list of the BILLBOARD_N most popular songs by number of people
who know them, in descending order. Break ties alphabetically.
"""
# TODO
pass
def all_bards(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> set[str]:
"""Return the set of the village's bards."""
# TODO
pass
def average_attendees(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> int:
"""
Return the average number of attendees at parties in the village.
Round up to the nearest integer.
"""
# TODO
pass
# Main process
def run(filename: str) -> dict[str, object]:
"""
Run the program: read the input, host the parties,
and return a dictionary of resulting statistics keyed by name:
unheard_songs, billboard_top, all_bards, average_attendees
filename is the name of an input file.
"""
# TODO
pass
# Run program
if __name__ == "__main__":
# Sample input from the handout -- you can tweak this if you like
stats_handout = run("handout_example.txt")
print("Results of handout sample input")
for key, value in stats_handout.items():
print(f"{key}: {value}")
print()
# Sample bigger input -- you can tweak this if you like
stats_bigger = run("bigger_example.txt")
print("Results of bigger sample input")
for key, value in stats_bigger.items():
print(f"{key}: {value}")
- Hello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. public static double listAdder(ArrayList<? extends Number> list) { ???} Examine the listAdder method fragment above. Complete the method so that it displays the list elements all on one line and returns the sum of the elements. Demonstrate the method by calling it in main with ArrayLists of three different classes that extend class Number. The operator instanceof can test for object type which would be necessary to have in a foreach loop for the data types types supported ( Integer , Byte and Double ) SAMPLE OUTPUT 12 21 7 16 8 13 Integer list totals 77.0 1.1 2.2 3.3 4.4 5.5 6.6 Double list…arrow_forwardComplete the searching and sorting algorithms in ModuleOne and ModuleTwo of the modules package. Test all sorting algorithms in AlgorithmsTest. You must build everything from scratch and cannot use any methods in other Java packages to make your algorithm. Restrictions : All classes/methods must be made by you. You are ONLY allowed to use java.util.Arrays.toString method You are NOT allowed to use any other the Java API Library classes/methods.arrow_forwardGiven a long string use recursion to traverse the string and replace every vowel (A,E,I,O,U) with an @ sign. You cannot change the method header. public String encodeVowels(String s){ // Your code here }arrow_forward
- JAVA you guys returned the question and said that it is a writing assignment but it is not. It is a java question, multiple-choice no coding. Please help me with this.arrow_forwardSubject: Java Programmingarrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forward
- Note: The code needs to be in Java and the starter code needs to be used as well.arrow_forwardPlease help me this using recursion and java. Create a sierpenski triangle. And please comment the code. Do not create the game using hashtaarrow_forwardThis question is in java The Sorts.java file is the sorting program we looked at . You can use either method listed in the example when coding. You want to add to the grades.java and calculations.java files so you put the list in numerical order. Write methods to find the median and the range. Have a toString method that prints the ordered list, the number of items in the list, the mean, median and range. Ex List: 20 , 30 , 40 , 75 , 93Number of elements: 5Mean: 51.60Median: 40Range: 73 Grades.java is the main file import java.util.Scanner;public class grades { public static void main(String[] args) { int n; calculation c = new calculation(); int array[] = new int[20]; System.out.print("Enter number of grades that are to be entered: "); Scanner scan = new Scanner(System.in); n = scan.nextInt(); System.out.println("Enter the grades: "); for(int i=0; i<n; i++) { array[i] = scan.nextInt(); if(array[i] < 0) {…arrow_forward
- Sorting an array of batting averages would be useful only if you sort another array in the same sequence in Java. Explain.arrow_forward%matplotlib inlineimport numpy as npfrom matplotlib import pyplot as pltfrom math import sin, cos, exp, pi, sqrtimport matharrow_forwardputty program for unix can can not get the screen to show the mountain list. how do i do this?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
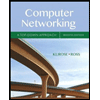
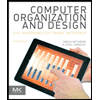
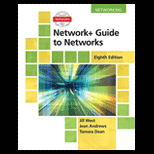
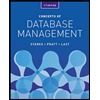
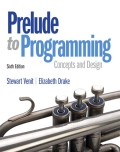
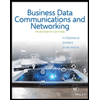