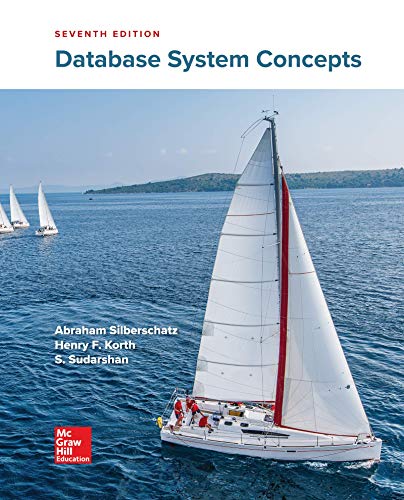
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
The function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths.
The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned.
For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"].
HINT: Python has a built-in function min() which is helpful here.
- Initialize accumulator variable newlist to be an empty list
- Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths
- Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration).
- AFTER the loop finishes, append the remaining items (if any) to newlist; use a decision statement to determine which list has remaining items -- then it just takes one line of code to append those items to newlist (using a slice of L1 or L2)
- return newlist
For example:
Test | Result |
---|---|
L1 = ["hop", "skip", "jump"] L2 = ["A", "B", "C", "D", "E"] print(interleave_lists(L1, L2)) | ['hop', 'A', 'skip', 'B', 'jump', 'C', 'D', 'E'] |
L1 = ["dive"] L2 = ["hop", "skip", "jump"] print(interleave_lists(L1, L2)) | ['dive', 'hop', 'skip', 'jump'] |
cats = ["Ziggy", "Troll Boy"] dogs = ["Ruthie", "Ame"] print(interleave_lists(cats, dogs)) | ['Ziggy', 'Ruthie', 'Troll Boy', 'Ame'] |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In Python programming languagearrow_forwardPython Qu Write a Python program (not a function!) that asks the user for nonnegative integers,one integer per line.When the user is finished entering their integers, they enter a negative integerto tell the program to stop asking them for more integers.Once the input is complete, the program prints "No integers to average"if the user provided no nonnegative integers,and the average of the nonnegative integers otherwise.You must NOT use lists, tuples, or dictionaries in your code.Here is a sample program run where the user enters 4 and then 8 and then -1.The program outputs Average: 6.048-1Average: 6.0Here is another run where the user types -3 to the first prompt.The program outputs No integers to average-3No integers to averagearrow_forwardIn python pleasearrow_forward
- The function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forwardThe function makeRandomList creates and returns a list of numbers of a given size(its argument). The numbers in the list are unique and range from 1 through thesize. They are placed in random order. Here is the code for the function:def makeRandomList(size):lyst = []for count in range(size):while True:number = random.randint(1, size)if not number in lyst:lyst.append(number)breakreturn lystYou can assume that range, randint, and append are constant time functions. Youcan also assume that random.randint more rarely returns duplicate numbers as therange between its arguments increases. State the computational complexity of thisfunction using big-O notation, and justify your answer. Only analytical work is requiredarrow_forwardWrite a function that accepts two arguments, a list and a number n. The list contains numbers. The function return a list of all the numbers from the input list that are greater than number n. greater_than_n(list, n)arrow_forward
- python LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardTo determine if two lists of integers are equivalent, you must write a function that accepts the lists as input and returns true or false (i.e. contain the same values). The greatest value of each list must be shown if the lists are dissimilar and the procedure is effective.arrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forward
- The mapped list pattern Our second pattern is the mapped list pattern, described in video 4 3 mapped list pattern. Often we need to write a function that takes a list as a parameter and returns a new list in which each item in the original list is "mapped" to a new item in the result list. For example, the following function takes a list of numbers as a parameter and returns a list of all the numbers squared, e.g. squares ( [1, 3, 7]) returns [1, 9, 49]. def squares (nums): "Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result Although this is just a special case of the accumulator pattern, it is so common that we give it its own name: the mapped list pattern. Consider the following function: def squares(nums): ""Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result If the main program calls print(squares ( [5, -3, 2, 7]) what is the state table for the function…arrow_forwardPlease answer quickly In pythonarrow_forwardUsing c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
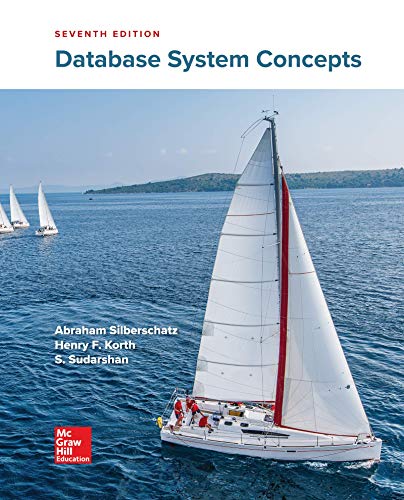
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
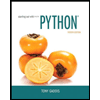
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
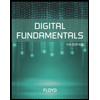
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
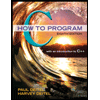
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
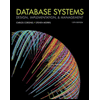
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
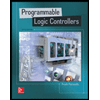
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education