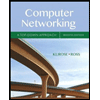
Please create a class named BST based on the information in the second screenshot. You don't have to modify the class Node. After that, please use the BST class and Node class to create a class BSTApp. Please make sure to use the same class name so that I don't get confused. Also, please make sure to use the following code as a starting point.
import java.util.*;
public class BSTTest{
public static void main(String[] args) {
// perform at least one test on each operation of your BST
}
private int[] randomArray(int size) {
// remove the two lines
int[] arr = new int[1];
return arr;
}
// the parameters and return are up to you to define; this method needs to be uncommented
// private test() {
//
// }
}
Base of class Node
public class Node {
int key;
Node left, right, parent;
public Node() {}
public Node(int num) {
key = num;
left = null;
right = null;
parent = null;
}
}
Base of class BST
import java.util.*;
class BST {
// do not change this
private Node root;
private ArrayList<Integer> data;
// DO NOT MODIFY THIS METHOD
public BST() {
root = null;
data = new ArrayList<Integer>(0);
}
// DO NOT MODIFY THIS METHOD
public ArrayList<Integer> getData() {
return data;
}
// DO NOT MODIFY THIS METHOD
public void inOrderTraversal() {
inOrderTraversal(root);
}
// DO NOT MODIFY THIS METHOD
private void inOrderTraversal(Node node) {
if (node != null) {
inOrderTraversal(node.left);
data.add(node.key);
inOrderTraversal(node.right);
}
}
// search
public boolean search(int target) {
// remove this line
return false;
}
// insert
public Node insert(int target) {
// remove this line
return root;
}
// note: you may need to implement several supporting methods for delete
public Node delete(int target) {
// remove this line
return root;
}
// you are welcome to add any supporting methods
}
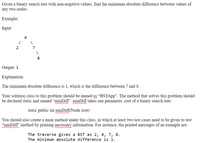
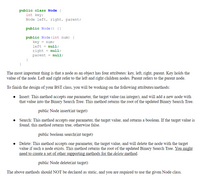

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 8 images

- Java Given main(), complete the SongNode class to include the printSongInfo() method. Then write the Playlist class' printPlaylist() method to print all songs in the playlist. DO NOT print the dummy head node.arrow_forwardSave the Word document and close them. Go to D2L and submit it by attaching the document file.You mayarrow_forwardMay you help with this code!!!! PLEASE, ASAP!arrow_forward
- Please help me with this questionarrow_forwardRo-Sham-Bo. Believe it or not, the classic game of Rock-PaperScissors has many other names. One of them is Ro-Sham-Bo. For this assignment, “Ro” will represent “Rock”, “Sham” will represent Paper, and “Bo” will represent Scissors. You will create a RoshamboPlayer class. It will have three attributes: PlayerName: String RoLimit: int ShamBoLimit: int It will also have an overloaded constructor that sets those three values. It will have two functions: playRound that takes in a string and returns a boolean value, and getName that takes in nothing and returns the PlayerName string. In your driver class for this assignment, you will create two RoshamboPlayer objects with the following values: p1: RoLimit == 30, ShamBoLimit == 60 p2: RoLimit = 40, ShamBoLimit = 85 You may name them whatever you like. You will prompt the user to choose one of these two to play against. Then you will create a loop that prompt the user to either play a round of Roshambo, or quit the game. If the user chooses…arrow_forwardPLEASE ANSWER ALL QUESTIONS ON THE NEXT PAGE: class bookType { public: void setBookTitle(string s); //sets the bookTitle to s void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter void setBookPrice(double cost); //sets the private member bookPrice to cost void setCopiesInStock(int noOfCopies); //sets the private member copiesInStock to noOfCopies void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the copiesInStock string getBookISBN() const; //returns the bookISBN double getBookPrice() const; //returns the bookPrice int showQuantityInStock() const; //returns the quantity in stock void updateQuantity(int addBooks); //adds addBooks to the quantityInStock, so that the quantity in stock now has it original // value plus the parameter sent to this function private: string…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
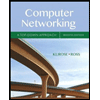
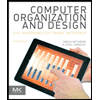
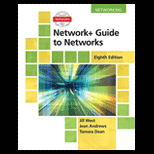
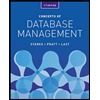
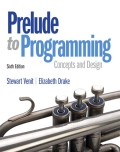
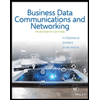