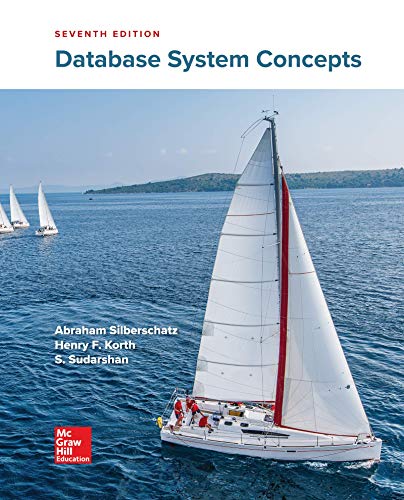
For the following Pseudocode help me to Design a UML Class Diagram. Thanks!
from tkinter import Tk,Button,Label
from tkinter import Canvas
from random import randint
root = Tk()
root.title("Catch the ball Game")
root.resizable(False,False)
canvas = Canvas(root, width=600, height=600)
canvas.pack()
limit = 0
dist = 5
score = 0
class Ball:
def __init__(self, canvas, x1, y1, x2, y2):
self.x1 = x1
self.y1 = y1
self.x2 = x2
self.y2 = y2
self.canvas = canvas
self.ball = canvas.create_oval(self.x1, self.y1, self.x2, self.y2,
fill = "red",tags = 'dot1')
def move_ball(self):
offset = 10
global limit
if limit >= 510:
global dist,score,next
if(dist - offset <= self.x1 and
dist + 40 + offset >= self.x2):
score += 10
canvas.delete('dot1')
ball_set()
else:
canvas.delete('dot1')
bar.delete_bar(self)
score_board()
return
limit += 1
self.canvas.move(self.ball,0,1)
self.canvas.after(10,self.move_ball)
class bar:
def __init__(self,canvas,x1,y1,x2,y2):
self.x1 = x1
self.y1 = y1
self.x2 = x2
self.y2 = y2
self.canvas = canvas
self.rod=canvas.create_rectangle(self.x1, self.y1, self.x2, self.y2,
fill="yellow",tags='dot2')
def move_bar(self,num):
global dist
if(num == 1):
self.canvas.move(self.rod,20,0)
dist += 20
else:
self.canvas.move(self.rod,-20,0)
dist-=20
def delete_bar(self):
canvas.delete('dot2')
def ball_set():
global limit
limit=0
value = randint(0,570)
ball1 = Ball(canvas,value,20,value+30,50)
ball1.move_ball()
def score_board():
root2 = Tk()
root2.title("Catch the ball Game")
root2.resizable(False,False)
canvas2 = Canvas(root2,width=300,height=300)
canvas2.pack()
w = Label(canvas2,text="\nOOPS...GAME IS OVER\n\nYOUR SCORE = "
+ str(score) + "\n\n")
w.pack()
button3 = Button(canvas2, text="PLAY AGAIN", bg="green",
command=lambda:play_again(root2))
button3.pack()
button4 = Button(canvas2,text="EXIT",bg="green",
command=lambda:exit_handler(root2))
button4.pack()
def play_again(root2):
root2.destroy()
main()
def exit_handler(root2):
root2.destroy()
root.destroy()
def main():
global score,dist
score = 0
dist = 0
bar1=bar(canvas,5,560,45,575)
button = Button(canvas,text="==>", bg="green",
command=lambda:bar1.move_bar(1))
button.place(x=300,y=580)
button2 = Button(canvas,text="<==",bg="green",
command=lambda:bar1.move_bar(0))
button2.place(x=260,y=580)
ball_set()
root.mainloop()
if(__name__=="__main__"):
main()

Step by stepSolved in 2 steps with 2 images

- After drawing the hierarchy, implement the classes by writing C# code foreach class. Then create objects of each class and call ALL methods onthese objects.arrow_forwardhow to do this in java that functions with the main class in the first image and can be compared with the container class in the second imagearrow_forwardcombines attributes and methods into one unit and hides its internal structure of objects. Select one: a.Information hiding b.Inheritance c.Polymorphism d.Encapsulationarrow_forward
- Create a UML class diagram of the application illustrating class hierarchy, collaboration, and the content of each class. There is only one class. There is a main method that calls four methods. I am not sure if I made the UML Class diagram correct. import java.util.Random; // import Random packageimport java.util.Scanner; // import Scanner Package public class SortArray{ // class name public static void main(String[] args) { //main method Scanner scanner = new Scanner(System.in); // creates object of the Scanner classint[] array = initializeArray(scanner); System.out.print("Array of randomly generated values: ");displayArray(array); // Prints unsorted array sortDescending(array); // sort the array in descending orderSystem.out.print("Values in desending order: ");displayArray(array); // prints the sorted array, should be in descending ordersortAscending(array); // sort the array in ascending orderSystem.out.print("Values in asending order: ");displayArray(array); // prints the…arrow_forwardHow do you distinguish between a domain and a workgroup?arrow_forwardHello! Need help with my Java Homework Please use Eclipse and Add a comments in each program to explain what your code is doing so I can understand it and learn. I really appreciate the help! Examine carefully the UML class diagram Attached: NOTE: Class Reptile was missing a toString() method. It has now been added. class Pet has an attribute of type java.util.Date. no specific dates are required for this attribute. compareTo(Dog) compares Dogs by weight. Create executable class TestPet as follows: create at least one Reptile pet and display it create an array of at least four Dog pets sort the array of Dogs by weight use a foreach loop to fully display all data for all dogs sorted by weight (see sample output) Sample Output Reptile name = Slinky, rock python, M Must be caged, crawls or slithers Not much sound, maybe a hiss, acquired Fri Feb 03 17:06:54 EST 2017 All dogs sorted by weight Dog name = Pedro, chihuahua, M Walks on a leash, weight 14 Barks or howls, acquired Fri…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
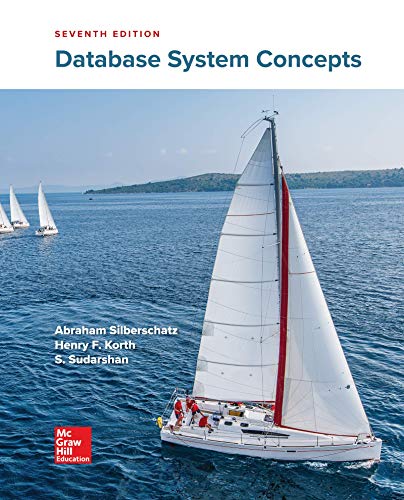
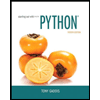
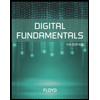
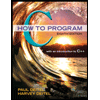
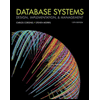
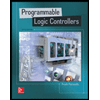