public class Main { public static void main(String args[]) { Main m = new Main(); m.go(); } public void go() { Customer c = new Customer("Tom", "Smith"); Order o = new Order(c); o.addItem(new Item("Greeting Card", 1.50, 1)); o.addItem(new Item("Baseball Glove", 54.00, 1)); o.addItem(new Item("Notebook", 2.50, 3)); System.out.println(o); } }
public class Main { public static void main(String args[]) { Main m = new Main(); m.go(); } public void go() { Customer c = new Customer("Tom", "Smith"); Order o = new Order(c); o.addItem(new Item("Greeting Card", 1.50, 1)); o.addItem(new Item("Baseball Glove", 54.00, 1)); o.addItem(new Item("Notebook", 2.50, 3)); System.out.println(o); } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
public class Main
{
public static void main(String args[])
{
Main m = new Main();
m.go();
}
public void go()
{
Customer c = new Customer("Tom", "Smith");
Order o = new Order(c);
o.addItem(new Item("Greeting Card", 1.50, 1));
o.addItem(new Item("Baseball Glove", 54.00, 1));
o.addItem(new Item("Notebook", 2.50, 3));
System.out.println(o);
}
}

Transcribed Image Text:Main.java - Notepad
A learn.zybooks.com/zybook/MCLACSC1328CohenSpring2022/chapter/5/section/2
Main.java - Notepad
O Main.java - Notepad
= zyBooks My librar
Order, Item, Customer
File Edit Format View Help
File Edit Format View Help
The class diagram below shows an Order class that "has a" Customer and zero or more Items.
Order
Item
0.*
private Customer m_cust;
private ArrayList<Item> m_items;
private String m_desc
private int m_qty
private double m_price
public Order(Customer cust)
public void additem(Item i)
public String toString()
public Item(String desc, double price, int qty)
public String toString)
1.1
Customer
private String m_first;
private String m_last;
public Customer(String first, String last)
public String toString)
Please create the Order, Customer, and Item classes shown above.
I have provided you with a read only Main class that you can use to test your code (you can download it below). You can use this to test
your code, but there is no need to submit it to zyBooks. I will be using my own main to grade your program.
When you have completed the Order, Customer, and Item classes, running the Main program should produce the following output:
Order for Smith, Tom
Order Items:
Greeting Card: 1 at 1.5
Baseball Glove: 1 at 54.0
Notebook: 3 at 2.5
364732.2186014. gx3zgy7
1:05 PM
梦 メ
O Type here to search
a
25°F
2/25/2022
Expert Solution

Explaination
Here is the step by step instructions
- Make sure that all the files are in the same folder and mentioned the statment package folderName; at start of every java file as there are 4 java files one for customer, one for item ,one for order, one for main java class
- We just had to create the two classes customer and item which have the constructor to initilize the object data members of the class and to string method to give the string representation of the class
- In the order class we have addItem method in which we have to add the item in the list of item . Hence use the method add to add the item in the list of items.
- Then we have to create the to string method
- Apply the for loop on the arraylist m_items to call the to string method on every item object in the list
- Call the to string method of the customers class to get the name of the person
- Check the code comments as everything is mentioned into that clearly .
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

Recommended textbooks for you
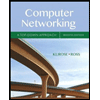
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
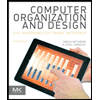
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
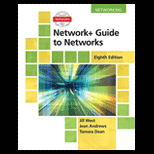
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
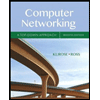
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
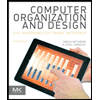
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
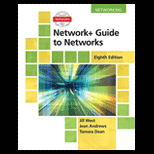
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
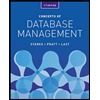
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
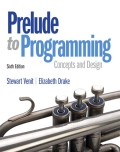
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
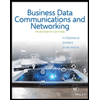
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY