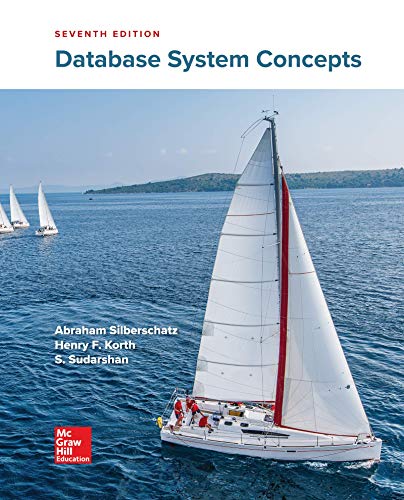
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
public class Course
{
String name;
String dept;
int number;
public Course (String n, String d, int n)
{
name = n;
dept = d;
number = n;
}
public String toString()
{
return "Course " + name + " in " + dept + " #: " + number;
}
public static void main (String [] args)
{
Scanner in = new Scanner(System.in);
add 10 lines using the scanner and saving them as strings The input for each line will be entered a String, a space, another String, and an int. Parse the input string into those three items and make an instance of Course. Add the new Course instance to a generic ArrayList of Course. print out each Course in the ArrayList.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Fix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forward1 public class Main { 1234567 7 8 9 10 11 ENERGET 12 13 14 15 16 17 18} public static void main(String[] args) { String present = ""; double r = Math.random(); // between 0 and 1 if (r > >>arrow_forwardpublic class Qla { Run | Debug public static void main (String [] args) { int n = 1000; int mySum 0; %3D for (int i = n; i >= 0; i--) { %3D if (i % 2 == 0) { mySum += i; } } Stdout.println(mySum);arrow_forward
- Question 37 public static void main(String[] args) { Dog[] dogs = { new Dog(), new Dog()}; for(int i = 0; i >>"+decision()); } class Counter { private static int count; public static void inc() { count++;} public static int getCount() {return count;} } class Dog extends Counter{ public Dog(){} public void wo(){inc();} } class Cat extends Counter{ public Cat(){} public void me(){inc();} } The Correct answer: Nothing is output O 2 woofs and 5 mews O 2 woofs and 3 mews O 5 woofs and 5 mews Oarrow_forwardclass Lab4Q1P3 { public static void main(String args[]) { int max = 10;while (max < 10) {System.out.println("count down: " + max);max--;}}} Do you know what is wrong with the program? I know that there is error on the 7th line but I don't know what to fix.arrow_forwardFix the errors and send the code please // Application allows user to enter a series of words // and displays them in reverse order import java.util.*; public class DebugEight4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); int x = 0, y; String array[] = new String[100]; String entry; final String STOP = XXX; StringBuffer message = new StringBuffer("The words in reverse order are\n"); System.out.println("Enter any word\n" + "Enter + STOP + when you want to stop"); entry = input.next(); while(!(entry.equals(STOP))) { array[x] = entry; ++x; System.out.println("Enter another word\n" + "Enter " + STOP + " when you want to stop"); entry = input.next(); } for(y = x - 1; y > 0; ++y) { message.append(array[y]); message.append("\n"); } System.out.println(message) }arrow_forward
- class TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forwardpublic class main { public static void main(String [] args) { Dog dog1=new Dog(“Spark”,2),dog2=new Dog(“Sammy”,3); swap(dog1, dog2); System.out.println(“dog1 is ”+ dog1); System.out.println(“dog2 is ”+ dog2); } public static void swap(Dog a, Dog b) { String nameA = a.getName(); String nameB = b.getName(); a.setName(nameB); b.setName(nameA); } What is the output of the main()?arrow_forwardpublic class Test { } public static void main(String[] args){ int a = 10; System.out.println(a*a--); }arrow_forward
- using System; class main { publicstaticvoid Main(string[] args) { Random r = new Random(); string[] ROYGBIV; ROYGBIV = new string[7] {"Red", "Orange", "Yellow", "Green", "Blue", "Indigo", "Violet"}; int randNo= r.Next(0,6); Console.Write("Hi, my name is Stevie. I am thinking of Rainbow colors.\nGuess which color I am thinking of? "); string guessedColor = Console.ReadLine(); do { if(guessedColor != ROYGBIV[randNo]) { Console.WriteLine("Your guess is NOT correct.Please enter another color"); guessedColor = Console.ReadLine(); } if(guessedColor == ROYGBIV[randNo]) { Console.WriteLine("You guessed correctly!"); } }while(guessedColor != ROYGBIV[randNo]); } } Hello! This program is written in C# to play a guessing game with the user but instead of numbers its with the colors of the rainbow. How can it be changed to the letters of the entire alphabet instead?arrow_forwardpublic class Test { } public static void main(String[] args) { String str = "Salom"; System.out.println(str.indexOf('t)); }arrow_forwardusing System; class main { publicstaticvoid Main(string[] args) { Random r = new Random(); int randNo= r.Next(1,11); Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? "); int guess = Convert.ToInt32(Console.ReadLine()); if (guess == randNo) { Console.WriteLine("You guessed correctly!"); } else { Console.WriteLine("I was thinking of the number "+randNo+"\nSorry! Play again."); } } } this is a guessing game program for a random number between 1-10 in C#. how can code be added to tell you if your guess is too high or too low from the chosen random number?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
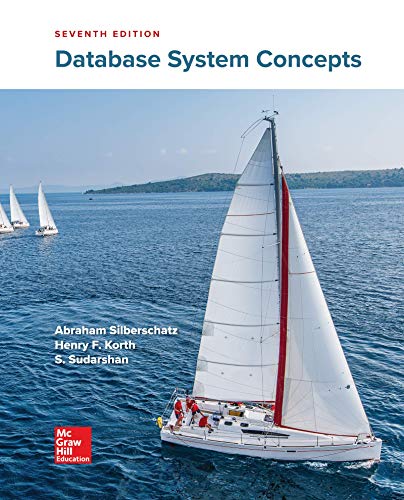
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
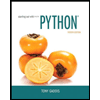
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
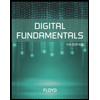
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
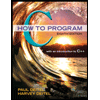
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
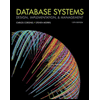
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
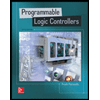
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education