Project 5 - Magic Squares Objectives The objective of this project is to have students practice with two-dimensional arrays and loops. Specifications n this project, you will write code to determine if a two-dimensional array of ints is a Magic Square. For a two- dimensional array of ints to be a Magic Square all of the following must be true: 1. The array must be square - in other words, the lengths of all rows and all columns must be the same. 2. The array must contain all integers from 1 to n*n, where n is the length of the rows and columns. 3. The sum of the numbers in each diagonal, each row, and each column must be the same. You have been given two classes: • MagicSquareTest.java - contains JUnit test cases for the MagicSquare class. Your code must pass all these tests. • MagicSquare.java - contains static methods to determine if a two-dimensional array of ints is a Magic Square: o isMagicSquare() - returns true if a two-dimensional array of ints meets all the criteria to be a Magic Square. This method is already completed for you. It uses all of the following methods: . issquare() - returns true if a two-dimensional array of ints is a square - in other words, the lengths of all rows and columns are the same. This method is already completed for you. • containsAllNumbers() - returns true if a square two-dimensional array of ints contains all integers from 1 to n*n, where n is the length of the rows and columns. This method is already completed for you. • diagonal1Sum() - returns the sum of the numbers in the upper-left to lower-right diagonal of a square two- dimensional array of ints . You must write the body for this method. • diagonal2Sum() - returns the sum of the numbers in the upper-right to lower-left diagonal of a square two- dimensional array of ints . You must write the body for this method. • rowSums () - if all the rows of a square two-dimensional array of ints have the same sum, returns that sum. Otherwise, returns -1. You must write the body for this method. It uses the following: . rowSum() - returns the sum of the numbers in a given row. You must write the body for this method. • colSums () - if all the columns of a square two-dimensional array of ints have the same sum, returns tha sum. Otherwise, returns -1. You must write the body for this method. Uses: • colSum() - returns the sum of the numbers in a given column. You must write the body for this method.
Project 5 - Magic Squares Objectives The objective of this project is to have students practice with two-dimensional arrays and loops. Specifications n this project, you will write code to determine if a two-dimensional array of ints is a Magic Square. For a two- dimensional array of ints to be a Magic Square all of the following must be true: 1. The array must be square - in other words, the lengths of all rows and all columns must be the same. 2. The array must contain all integers from 1 to n*n, where n is the length of the rows and columns. 3. The sum of the numbers in each diagonal, each row, and each column must be the same. You have been given two classes: • MagicSquareTest.java - contains JUnit test cases for the MagicSquare class. Your code must pass all these tests. • MagicSquare.java - contains static methods to determine if a two-dimensional array of ints is a Magic Square: o isMagicSquare() - returns true if a two-dimensional array of ints meets all the criteria to be a Magic Square. This method is already completed for you. It uses all of the following methods: . issquare() - returns true if a two-dimensional array of ints is a square - in other words, the lengths of all rows and columns are the same. This method is already completed for you. • containsAllNumbers() - returns true if a square two-dimensional array of ints contains all integers from 1 to n*n, where n is the length of the rows and columns. This method is already completed for you. • diagonal1Sum() - returns the sum of the numbers in the upper-left to lower-right diagonal of a square two- dimensional array of ints . You must write the body for this method. • diagonal2Sum() - returns the sum of the numbers in the upper-right to lower-left diagonal of a square two- dimensional array of ints . You must write the body for this method. • rowSums () - if all the rows of a square two-dimensional array of ints have the same sum, returns that sum. Otherwise, returns -1. You must write the body for this method. It uses the following: . rowSum() - returns the sum of the numbers in a given row. You must write the body for this method. • colSums () - if all the columns of a square two-dimensional array of ints have the same sum, returns tha sum. Otherwise, returns -1. You must write the body for this method. Uses: • colSum() - returns the sum of the numbers in a given column. You must write the body for this method.
Chapter8: Arrays
Section: Chapter Questions
Problem 9PE
Related questions
Question
100%

Transcribed Image Text:Project 5 - Magic Squares
Objectives
The objective of this project is to have students practice with two-dimensional arrays and loops.
Specifications
In this project, you will write code to determine if a two-dimensional array of ints is a Magic Square. For a two-
dimensional array of ints to be a Magic Square all of the following must be true:
1. The array must be square - in other words, the lengths of all rows and all columns must be the same.
2. The array must contain all integers from 1 to n*n, where n is the length of the rows and columns.
3. The sum of the numbers in each diagonal, each row, and each column must be the same.
You have been given two classes:
• MagicSquareTest.java - Contains JUnit test cases for the MagicSquare class. Your code must pass all these tests.
• MagicSquare.java - contains static methods to determine if a two-dimensional array of ints is a Magic Square:
o isMagicSquare() - returns true if a two-dimensional array of ints meets all the criteria to be a Magic Square.
This method is already completed for you. It uses all of the following methods:
· issquare() - returns true if a two-dimensional array of ints is a square - in other words, the lengths of
all rows and columns are the same. This method is already completed for you.
• containsAllNumbers() - returns true if a square two-dimensional array of ints contains all integers from
1 to n*n, where n is the length of the rows and columns. This method is already completed for you.
• diagonal1Sum() - returns the sum of the numbers in the upper-left to lower-right diagonal of a square two-
dimensional array of ints . You must write the body for this method.
1 diagonal2Sum() - returns the sum of the numbers in the upper-right to lower-left diagonal of a square two-
dimensional array of ints . You must write the body for this method.
I rowSums () - if all the rows of a square two-dimensional array of ints have the same sum, returns that
sum. Otherwise, returns -1. You must write the body for this method. It uses the following:
• rowSum() - returns the sum of the numbers in a given row. You must write the body for this
method.
. colSums () - if all the columns of a square two-dimensional array of ints have the same sum, returns that
sum. Otherwise, returns -1. You must write the body for this method. Uses:
- colSum() - returns the sum of the numbers in a given column. You must write the body for this
method.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
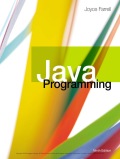
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
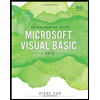
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
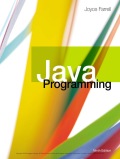
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
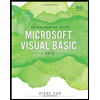
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
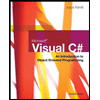
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,