1. Quit (exit the program) 2. Add (to the ArrayList) all the marks information about a coursework or research student by reading it from another text file and determine the student’s overall mark and grade. You will need to consider how to deal with the different assessment components for the coursework and research students. You may use two different text files, one for coursework students and another for research students. The program should read the correct text file for this purpose. 3. Given student number (ID), remove the specified student and relevant information from the ArrayList. It is always good to ask the user to confirm again before removing the record. For confirmation, output the student number (ID) and the name to the user. 4. Output from the ArrayList the details (all information including the overall mark and the grade) of all students currently held in the ArrayList. 5. Compute and output the overall mark and grade for coursework or research students 6. Determine and display how many coursework or research students obtained an overall mark equal to or above the average overall mark and how many obtained an overall mark below the average overall mark 7. Given a coursework or research student number (ID), view all details of the student with that number. If the student is not found in the ArrayList, an appropriate error message is to be displayed 8. Given a coursework or research student’s name (both surname and given name – ignoring case), view all details of that student. If the student is not found in the ArrayList, an appropriate error message is to be displayed. If more than one student having the same name, display all of them. 9. Sort the ArrayList of the student objects into ascending order of the students’ numbers (IDs), and output the sorted array - implement an appropriate sorting algorithm for this, and explain why such algorithm is selected (in internal and external documentation). 10. Output the sorted ArrayList from (9) to a CSV file. If the ArrayList is not sorted, this option cannot be selected I am having problem trying to implement the 9th and 10th menu option into my program. the rest of the menu is working fine, can you help me find a way to implement this two options?
1. Quit (exit the program)
2. Add (to the ArrayList) all the marks information about a coursework or research student by
reading it from another text file and determine the student’s overall mark and grade.
You will need to consider how to deal with the different assessment components for the
coursework and research students. You may use two different text files, one for coursework
students and another for research students. The program should read the correct text file for
this purpose.
3. Given student number (ID), remove the specified student and relevant information from the
ArrayList. It is always good to ask the user to confirm again before removing the record. For
confirmation, output the student number (ID) and the name to the user.
4. Output from the ArrayList the details (all information including the overall mark and the
grade) of all students currently held in the ArrayList.
5. Compute and output the overall mark and grade for coursework or research students
6. Determine and display how many coursework or research students obtained an overall mark
equal to or above the average overall mark and how many obtained an overall mark below the
average overall mark
7. Given a coursework or research student number (ID), view all details of the student with that
number. If the student is not found in the ArrayList, an appropriate error message is to be
displayed
8. Given a coursework or research student’s name (both surname and given name – ignoring
case), view all details of that student. If the student is not found in the ArrayList, an
appropriate error message is to be displayed. If more than one student having the same name,
display all of them.
9. Sort the ArrayList of the student objects into ascending order of the students’ numbers (IDs),
and output the sorted array - implement an appropriate sorting
why such algorithm is selected (in internal and external documentation).
10. Output the sorted ArrayList from (9) to a CSV file. If the ArrayList is not sorted, this option
cannot be selected
I am having problem trying to implement the 9th and 10th menu option into my program. the rest of the menu is working fine, can you help me find a way to implement this two options?

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

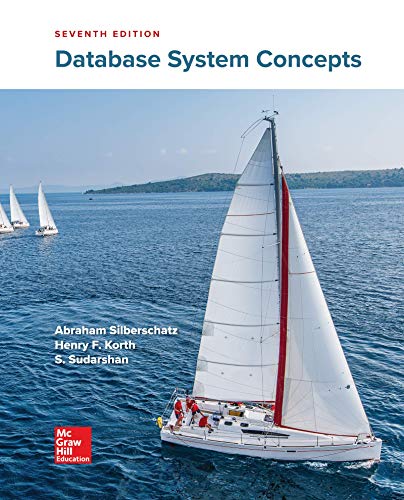
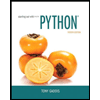
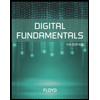
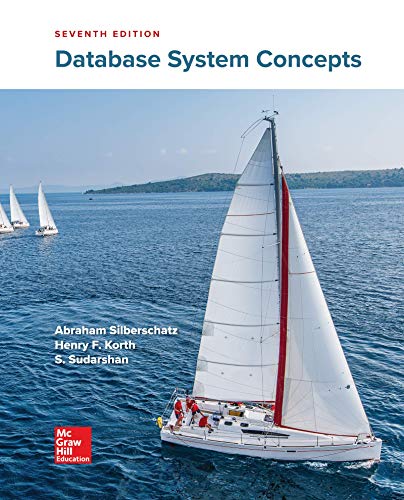
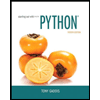
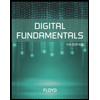
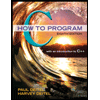
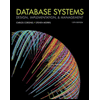
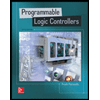