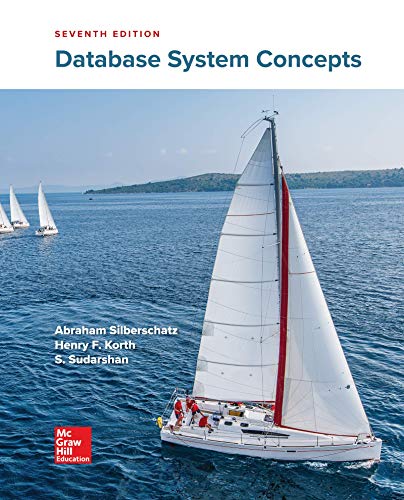
JAVA
Your teacher has created two arrays, each holding the results of tests, say Test 1 and Test 2. You need to create a new array which holds the averages of these two tests. You may assume that the first element of the first test array refers to the student who also has the grade in the first element of the second test, and the last element in each array are the grades the last student earned for each test. All students received grades for both tests, meaning both test arrays are of the same length.
Complete the method, named makeAverage, in the class named Grades.java. There are two parameters to this method: the first is the integer array representing the grades of the first test, and the second is the array containing the grades of the second test. The new average array should be returned by the method. The grades should be treated as double variables.
For example, consider the test grades for the five students in the following arrays:
[ 87 ] [ 91 ]
[ 76 ] [ 76 ]
[ 94 ] [ 95 ]
[ 82 ] [ 86 ]
[ 72 ] [ 67 ]
The first student has an exam average of 89.0 (= (87+91)/2 = 178/2), the second student has an exam average of 76.0 (= (76+76)/2 = 152/2), the third student has an exam average of 94.5 (= (94+95)/2 = 189/2), etc. Hence, the new average array should match the following:
[ 89.0 ]
[ 76.0 ]
[ 94.5 ]
[ 84.0 ]
[ 69.5 ]

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Please type and execute this java program and also need an output for this java program as soon as possible.arrow_forwardJumping in the Mega Millions fever, your lab is to write a program that simulates the Mega Millions lottery draw. Your program must have an array of five integers named winningNumbers, with a randomly generated number in the range of 0 through 9 for each element in the array. Your program should ask the user to enter five numbers and should store them in a second integer array named player. The program must compare the corresponding elements in the two arrays and count how many numbers match. For example, the following shows the winningNumbers array and the Player array with sample numbers stored in each. There are two matching elements, elements 2 and 4. Once the user has entered a set of numbers, the program should display the winning numbers and the player's numbers and tell how many numbers matched. Here is an example of the possible output from your code: /* SAMPLE RESULTS RUN 1 Submit the five numbers of your lottery number, separated by blanks: 93441 Winning number: 30766 Your…arrow_forwardJAVAWrite a program that searches person's name and display all matched name. For example, if a user inputs the letter "Davis", the program searches all name which includes "Davis" in an array. Then, display all matched names on the screen. You must declare a single dimensional array which has all people’s name. Use methods of string classarrow_forward
- Javaarrow_forwardWrite a program that simulates a lottery. The program should have an array of five integers named winningDigits, with a randomly generated number in the range of 0 through 9 for each element in the array. The program should ask the user to enter five digits and should store them in a second integer array named player. The program must compare the corresponding elements in the two arrays and count how many digits match. For example, the following shows the winningDigits array and the Player array with sample numbers stored in each. There are two matching digits, elements 2 and 4. The grand prize winner is determined by all 5 matching digits. The program should use functions for the following processes (3):Random number generation for lotteryPrompt for user data entryMatch Processing and Winner Determinationarrow_forwardan int variable k, an int array currentMembers that has been declared and initialized, an int variable nMembers that contains the number of elements in the array, an int variable memberID that has been initialized, and a bool variable isAMember, Write code that assigns true to isAMember if the value of memberID can be found in currentMembers, and that assigns false to isAMemberotherwise. Use only k, currentMembers, nMembers, and isAMember.arrow_forward
- This is need in Java Declare an array named temperatures that can hold 10 doubles.arrow_forwardYou are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and the course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade. A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each…arrow_forward1. Array splitting in Java Create an integer array with 10 integer numbers, initialize the array arbitrarily but make sure there are both positive and negative integers. Then generate two arrays out of the original array, one array with all natural numbers (positive numbers or zeros) and another one with all negative numbers. Print out the number of elements and the detailed elements in each array.arrow_forward
- 10 Build the Sudoku Reviewer ( the testing program). The specification is below. Please note: you DO NOT have to build a Sudoku solver. To test you just need to build a class that generates a 9x9 2D array with the values you want to test. You can assume the value types are valid. (integers from 1-9). 1-The main purpose is to make sure the Sudoku solver actually solved it correctly! 2- You are also confirming the solver solves puzzle in no longer than a minute 3- Solver (not the reviewer) requires 7 INDICES (ELEMENTS) filled in the input array at least. 4- The reviewer receives both the original puzzle and the solution. The Reviewer needs to confirm the solution is not only a valid SudoKu solution but also the solution to the original puzzle. 5- Solver will return a 2d array filled with -1s if input array has an error/bad input (exception). Reviewer will check for this case.arrow_forwardIn this project you will generate a poker hand containing five cards randomly selected from a deck of cards. The names of the cards are stored in a text string will be converted into an array. The array will be randomly sorted to "shuffle" the deck. Each time the user clicks a Deal button, the last five cards of the array will be removed, reducing the size of the deck size. When the size of the deck drops to zero, a new randomly sorted deck will be generated. A preview of the completed project with a randomly generated hand is shown in Figure 7-50.arrow_forwardCreate an array of objects of the Person class, of size 4. Create three objects of the Person class, with values, and assign the objects to the array. Loop through the array and print the name, job, and email of all Personobjects.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
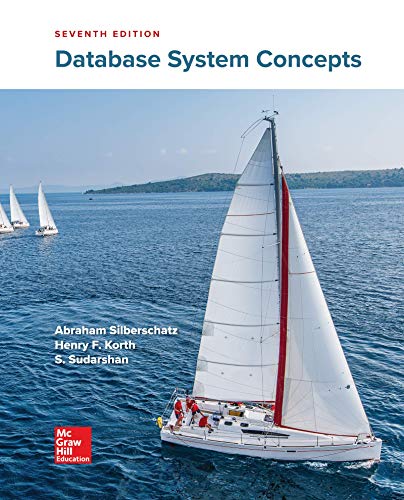
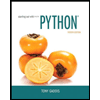
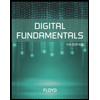
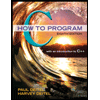
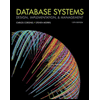
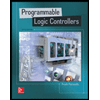