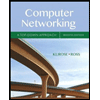
Use c++
Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions:
.getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array.
.getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row.
.getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column.
.getHighestInRow: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the highest value in the specified row of the array.
.getLowestInRow: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the lowest value in the specified row of the array.

Required: Write a program that creates a two-dimensional array initialized with test data.
all the code and screenshot with output has been provided in next step.
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- FYI: Please write the code in Pseudocode (no programming language please) 1. Write pseudocode to load a single array with data. Then search that array for a match. Here are the specifics. You do NOT have to write the entire program. Load the array with data from a file named customerNumbers Ask the user to enter their customer number Search the array for the customer number If you find a match output FOUND If there is no match output NOT FOUNDarrow_forwardProgramming Language: C++ Please use the resources included and provide notes for understanding. Thanks in advance. Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return…arrow_forwardIn C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forward
- When traversing an array, which of the following statements does not need to keep track of the individual array subscripts: Do...Loop, For...Next, or For Each...Next?arrow_forwardC++ Programming Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return 0;} // Add function definitions here WorldSeriesChampions.txt…arrow_forwardC code which returns the largest of the 6 integer number array according to integer array sent into it and the array length. Also include minimum, sum and average functions.arrow_forward
- use c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forwardTRUE OR FALSE, C++ When passing an array to a function, you must include & in front of the array name. When passing an array to a function, you must include & in front of the array name. It is possible to have a 2-dimensional array where each row has a different number of columns. The * is called the address of operator.arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
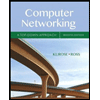
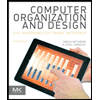
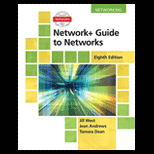
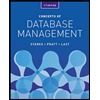
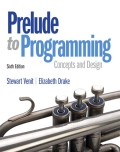
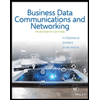