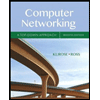
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
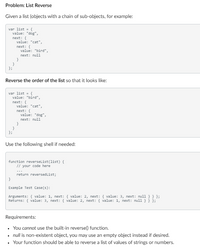
Transcribed Image Text:Problem: List Reverse
Given a list (objects with a chain of sub-objects, for example:
var list = {
value: "dog",
next: {
value: "cat",
next: {
value: "bird",
next: null
}
}
};
Reverse the order of the list so that it looks like:
var list =
value: "bird",
next: {
value: "cat",
next: {
value: "dog" ,
next: null
}
}
};
Use the following shell if needed:
function reverselist(list) {
// your code here
...
return reversedList;
Example Test Case(s):
Arguments: { value: 1, next: { value: 2, next: { value: 3, next: null } } };
Returns: { value: 3, next: { value: 2, next: { value: 1, next: null }}};
Requirements:
• You cannot use the built-in reverse) function.
• null is non-existent object, you may use an empty object instead if desired.
• Your function should be able to reverse a list of values of strings or numbers.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Similar questions
- OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. The code must have a function that takes a pair of strings (tuple) and returns a unit. You must avoid this error that is attached as an image. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction -Command mul refers to integer multiplication -Command div refers to integer division -Command rem refers to the remainder of integer…arrow_forwardRecursive Multiplication Given an JavaScript object list of books that each have a pages attribute to define the number of pages in the book, find the product of all the pages in the object list of books using recursion (you must use recursion to solve this problem). Keep in mind: The input list object may be completely empty (ex. {}) The next attribute may not be defined function getPageCount(list) {// your code here// returns an integer}Example test case:Input: {"book":"A","pages":1,"next":{"book":"B","pages":2,"next":{"book":"C","pages":3,"next": null}}}Output: 6Reasoning: 1 * 2 * 3 pagesarrow_forwardimport random; #importing random module aGrades=[]; #list to store grades of all tests for i in range(0,7):aGrades.append(random.randrange(0,100)); #generating 7 random values for testsexamCount=len(aGrades)-1; #examCount is a variable to store count of all exams except last exam totalPoints=sum(aGrades)-aGrades[len(aGrades)-1]; #totalPoints is a variable to store sum of all scores excluding last exam testAverage=(totalPoints)/examCount; #testAverage is a variable used to store the average of scores except last exam finalAverage= (testAverage*0.6)+(aGrades[len(aGrades)-1]*0.4); #finalAverage is a variable to store weighted average of the tests print(aGrades); #printing list of gradesprint(testAverage); #printing test averageprint(finalAverage); #printing final average How do i rewrite the Grades List programs above using a loop instead of referencing the elements of the lists by index.arrow_forward
- The program requires me to remove all item for a list, but when doing the test case for it, it only removes one. What would be the problem in this code?Given code: #include "MovieList.h"#include <iostream> using namespace std;// Constructor functionMovieList::MovieList() { top = nullptr; } // Destructor functionMovieList::~MovieList() { MovieNode *p = nullptr; while (top->next != nullptr) { p = top; top = top->next; delete p; } delete top; p = nullptr; top = nullptr; } void MovieList::display(ostream &out) { MovieNode *t = top; int pos = 0; // Function to display the movie list while (t != nullptr) { out << pos << ": " << t->title << endl; pos++; t = t->next; } } int MovieList::count() { MovieNode *t = top; int pos = 0; // Counting based off the movie list while (t != nullptr) { pos++; t = t->next; } return pos; } void…arrow_forwardWrite a python hangman program Modify your program so that the following functionality is added:1. Instead of selecting the word from a hard coded list, incorporate problem2 so that thelist is read from a file, then a word is randomly selected from this list.2. when the game ends, the following information should be outputted to a new file:● the word● the state of the hangman● if the user won or lostExample outputs:quokkaO\||You won!kangarooO\|/|/ \You lostarrow_forwarden "MyDoubleLinkedList" class: te a method called "PrintListInReverse" to print the list backward. example, Givenarrow_forward
- struct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardstarter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardlet buckets (f:'a->'a->bool) (lst:'a list): 'a list list = let rec find (find_f: 'a->'a->bool)(find_ht: 'a) (find_acc:'a list list):'a list list = match find_acc with []-> [[find_ht]] | head::tail -> match head with h::_-> if f h find_ht then (find_ht::head)::tail else head::(find f find_ht tail) in let rec parse (parse_f: 'a->'a->bool) (parse_lst:'a list) (parse_acc: 'a list list): 'a list list = match parse_lst with [] -> parse_acc | ht::tl -> parse f tl (find f ht parse_acc) in match lst with []->[] | ht::tl -> parse f tl [[ht]];; let buckets (f:'a->'a->bool) (lst:'a list): 'a list list = let rec find (find_f: 'a->'a->bool)(find_ht: 'a) (find_acc:'a list list):'a list list = match find_acc with []-> [[find_ht]] | head::tail -> match head with h::_-> if f h find_ht then (find_ht::head)::tail else head::(find f find_ht tail) in let rec parse (parse_f: 'a->'a->bool)…arrow_forward
- Pythong help!arrow_forwardA-1: Let l = [−1, −2, . . . , −10] be an existing list. Construct a new list from l by dividing each evennumber element in l by 2. Print the new list. Do not use NUMPY library.A-2: Create a new list containing the following elements using list comprehension:[1, −1, 2, −2, 3, −3, ..., 9, −9]A-3: Consider the following coded string. Create a list of all contiguous (connected without space) lettersin the order of their appearance."Vjg dguv rtqitcou ctg ytkvvgp uq vjcv eqorwvkpi ocejkpgu ecp rgthqto vjgoswkemn{0 Cnuq. vjg dguv rtqitcou ctg ytkvvgp uq vjcv jwocp dgkpiu ecp wpfgtuvcpfvjgo engctn{0 C iqqf guuc{kuv cpf c iqqf rtqitcoogt jcxg c nqv kp eqooqp0"A-4: In the list obtained after executing task(s) from Part(A-3), remove newline characters (if any).A-5: For the list obtained after executing task(s) from Part(A-4), for each word in the list do thefollowing: Breakdown the word into list of letters. Convert each of the above letters into integer, using ord() function. Update the…arrow_forwardPYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
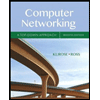
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
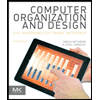
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
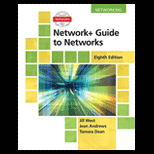
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
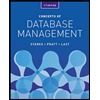
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
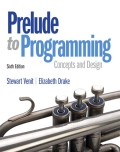
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
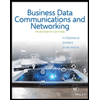
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY