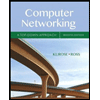
PLEASE HELP, PYTHON THANK YOU
def add_vertex(self, vertex):
if vertex not in self.__graph_dict:
self.__graph_dict[vertex] = []
def add_edge(self, edge):
edge = set(edge)
(vertex1, vertex2) = tuple(edge)
#################
# Problem 4:
# Check to see if vertex1 is in the current graph dictionary
##################
#DELETE AND PUT IN THE IF STATEMENTS
self.__graph_dict[vertex1].append(vertex2)
else:
self.__graph_dict[vertex1] = [vertex2]
def __generate_edges(self):
edges = []
#################
# Problem 5:
# Loop through all of the data in the graph dictionary and use the variable vertex for the loop'ed data
##################
#DELETE AND PUT IN THE LOOP STATEMENTS
for neighbour in self.__graph_dict[vertex]:
if {neighbour, vertex} not in edges:
edges.append({vertex, neighbour})
return edges
def find_path(self, start_vertex, end_vertex, path=None):
if path == None:
path = []
graph = self.__graph_dict
path = path + [start_vertex]
#################
# Problem 6:
# Check to see if the start_vertex equals the end_vertex
# if so, return the path
##################
#DELETE AND PUT IN THE IF STATEMENT
#################
# Problem 7:
# Check to see if the start_vertex is not in the graph
# if so, return None
##################
#DELETE AND PUT IN THE IF STATEMENT

Step by stepSolved in 3 steps with 1 images

- please help in c++arrow_forwardThis is a python programming question Python Code: class Node: def __init__(self, initial_data): self.data = initial_data self.next = None class LinkedList: def __init__(self): self.head = None self.tail = None def append(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: self.tail.next = new_node self.tail = new_node def prepend(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: new_node.next = self.head self.head = new_node def insert_after(self, current_node, new_node): if self.head == None: self.head = new_node self.tail = new_node elif current_node is self.tail: self.tail.next = new_node self.tail = new_node else: new_node.next = current_node.next…arrow_forwardimport edu.princeton.cs.algs4.StdOut; public class LinkedIntListR { // helper linked list node class private class Node { private int item; private Node next; public Node(int item, Node next) { this.item = item; this.next = next; } } private Node first; // first node of the list public LinkedIntListR() { first = null; } public LinkedIntListR(int[] data) { for (int i = data.length - 1; i >= 0; i--) first = new Node(data[i], first); } public void addFirst(int data) { first = new Node(data, first); } public void printList() { if (first == null) { StdOut.println("[]"); } else if (first.next == null) { StdOut.println("[" + first.item + "]"); } else { StdOut.print("[" + first.item); for(Node ptr = first.next; ptr != null; ptr = ptr.next) StdOut.print(", " + ptr.item); StdOut.println("]"); } } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; LinkedIntListR other =…arrow_forward
- Computer Science lab3.h ------------- #include<stdio.h> #include<stdlib.h> #ifndef LAB3_H #define LAB3_H // A linked list node struct Node { int data; //Data struct Node *next; // Address to the next node }; //initialize: create an empty head node (whose "data" is intentionally missing); This head node will not be used to store any data; struct Node *init () { //create head node struct Node *head = (struct Node*)malloc(sizeof(struct Node)); } //Create a new node to store data and insert it to the end of current linked list; the head node will still be empty and data in the array in "main.c" are not stored in head node void insert(struct node *head, int data) { struct Node *newNode = (struct Node*)malloc(sizeof(struct Node)); new_node->data = data; new_node->next= head; } //print data for all nodes in the linked list except the head node (which is empty) void display (struct Node *head) { struct Node *current_node = head; while ( current_node != NULL) { printf("%d ",…arrow_forwardHi i need an implementation on the LinkListDriver and the LinkListOrdered for this output : Enter your choice: 1 Enter element: Mary List Menu Selections 1. add element 2_remove element 3.head element 4. display 5.Exit Enter your choice: 3 Element @ head: Mary List Menu Selections 1-add element 2-remove element 3_head element 4.display 5-Exit Enter your choice: 4 1. Mary List Menu Selections 1. add element 2. remove element 3.head element 4. display 5. Exit Enter your choice: LinkedListDriver package jsjf; import java.util.LinkedList; import java.util.Scanner; public class LinkedListDriver { public static void main(String [] args) { Scanner input = new Scanner(System.in); LinkedList list = new LinkedList(); int menu = 0; do { System.out.println("\nList menu selection\n1.Add element\n2.Remove element\n3.Head\n4.Display\n5.Exit"); System.out.println();…arrow_forwardimport java.util.*;public class MyLinkedListQueue{ class Node { public Object data; public Node next; private Node first; public MyLinkedListQueue() { first = null; } public Object peek() { if(first==null) { throw new NoSuchElementException(); } return first.data; } public void enqueue(Object element) { Node newNode = new newNode(); newNode.data = element; newNode.next = first; first = newNode; } public boolean isEmpty() { return(first==null); } public int size() { int count = 0; Node p = first; while(p ==! null) { count++; p = p.next; } return count; } } } Hello, I am getting an error message when I execute this program. This is what I get: MyLinkedListQueue.java:11: error: invalid method declaration; return type required public MyLinkedListQueue() ^1 error I also need to have a dequeue method in my program. It is very similar to the enqueue method. I'll…arrow_forward
- PYTHON class BSTnode: def __init__(self, key = None, left = None, right = None, parent = None): # Initialize the a node. self.__key = key # value for the node self.__left = left self.__right = right self.__parent = parent def setkey(self, newkey): # Sets the key value of the node to newkey ####################################################################### # Remove the pass and write your code ####################################################################### pass ####################################################################### # End code ####################################################################### def getkey(self): # returns the key value of the node ####################################################################### # Remove the pass and write your code #######################################################################…arrow_forwardPLEASE HELP, PYTHON THANK YOU class Graph(object): def __init__(self, graph_dict=None): ################# # Problem 1: # Check to see if the graph_dict is none # if so, create an empty dictionary and store it in graph_dict ################## #DELETE AND PUT IN THE IF AND ASSIGNMENT STATEMENTS self.__graph_dict = graph_dict ################# # Problem 2: # Create a method called vertices and pass in self as the parameter ################## #DELETE AND PUT IN THE METHOD DEFINITION """ returns the vertices of a graph """ return list(self.__graph_dict.keys()) def edges(self): """ returns the edges of a graph """ ################# # Problem 3: # Return the results of the __generate_edges ################## #DELETE AND PUT IN THE RETURN STATEMENTSarrow_forwardPlease help in Python 3. Given code for BST.py ## Node classclass Node():def __init__(self, val):self.__value = valself.__right = Noneself.__left = Nonedef setLeft(self, n):self.__left = ndef setRight(self, n):self.__right = ndef getLeft(self):return self.__leftdef getRight(self):return self.__rightdef getValue(self):return self.__value # BST classclass BST():def __init__(self):self.__root = Nonedef append(self, val):node = Node(val)if self.__root == None:self.__root = nodereturncurrent = self.__rootwhile True:if val <= current.getValue():if current.getLeft() == None:current.setLeft(node)returnelse:current = current.getLeft()else:if current.getRight() == None:current.setRight(node)returnelse:current = current.getRight()def searchLength(self, val):if self.__root == None:return -1current = self.__rootlength = 0while current != None:if current.getValue() == val:return length + 1elif val < current.getValue():length += 1current = current.getLeft()else:length += 1current =…arrow_forward
- This data structure provides a variety of benefits over others, such as a linked list or tree.arrow_forwardPython code: ##############################class Node: def __init__(self,value): self.left = None self.right = None self.val = value ###############################class BinarySearchTree: def __init__(self): self.root = None def print_tree(node): if node == None: return print_tree(node.left) print_tree(node.right) print(node.val) ################################################## Task 1: get_nodes_in_range function################################################# def get_nodes_in_range(node,min,max): # # your code goes here # pass # fix this if __name__ == '__main__': BST = BinarySearchTree() BST.root = Node(10) BST.root.left = Node(5) BST.root.right = Node(15) BST.root.left.left = Node(2) BST.root.left.right = Node(8) BST.root.right.left = Node(12) BST.root.right.right = Node(20) BST.root.right.right.right = Node(25) print(get_nodes_in_range(BST.root, 6, 20))arrow_forwardBelow you're given a Node class and a LinkedList class. You will implement a method for the LinkedList class named "delete48in148". That is, whenever there is a sequence of nodes with values 1, 4, 8, we delete the 4 and 8. For exCample, Before: 1 -> 4 -> 8 LAfter: 1 Before: 7 -> 1 -> 4 -> 8 -> 9 -> 4 -> 8 After: 7 -> 1 -> 9 -> 4 -> 8 Before: 7 -> 1 -> 4 -> 8 -> 4 -> 8 -> 4 -> 8 -> 9 After: 7 -> 1 -> 9 Note from the above example that, after deleting one instance of 48, there may be new instances of 148 formed. You must delete ALL of them. Requirement: Your implementation must be ITERATIVE (i.e., using a loop). You must NOT use recursion. Recursive solutions will NOT be given marks. import ... # No other import is allowedarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
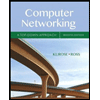
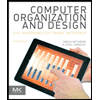
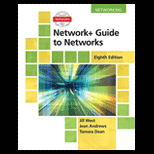
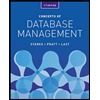
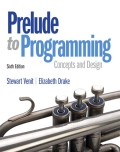
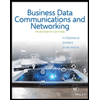