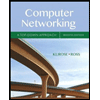
Please do P1 by redefining and using the student.py code. Strictly use the student.py code, you can change and add some code but you have to use it to create P1. Thanks a lot!
P1: Add three methods to the Student class that compare two Student objects. One method should test for equality. A second method should test for less than. The third method should test for greater than or equal to. In each case, the method returns the result of the comparison of the two students’ names. Include a main function that tests all of the comparison operators.
***student.py***
"""
File: student.py
Resources to manage a student's name and test scores.
"""
class Student(object):
"""Represents a student."""
def __init__(self, name, number):
"""All scores are initially 0."""
self.name = name
self.scores = []
for count in range(number):
self.scores.append(0)
def getName(self):
"""Returns the student's name."""
return self.name
def setScore(self, i, score):
"""Resets the ith score, counting from 1."""
self.scores[i - 1] = score
def getScore(self, i):
"""Returns the ith score, counting from 1."""
return self.scores[i - 1]
def getAverage(self):
"""Returns the average score."""
return sum(self.scores) / len(self._scores)
def getHighScore(self):
"""Returns the highest score."""
return max(self.scores)
def __str__(self):
"""Returns the string representation of the student."""
return "Name: " + self.name + "\nScores: " + \
" ".join(map(str, self.scores))
def main():
"""A simple test."""
student = Student("Ken", 5)
print(student)
for i in range(1, 6):
student.setScore(i, 100)
print(student)
if __name__ == "__main__":
main()

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 5 images

- Write a class called Alien. The Alien class must have one field of type String called name, one of type String called planet and one field of type boolean called humanoid. Write one constructor that takes three parameters to initialise all of these fields. The parameters must be passed in the order name, planet and humanoid status. IntelliJ hint (this won't be in the real test): put your cursor after the last field; right-click, choose Generate, choose Constructor, select the fields that are set via parameters and click Ok. Check that the constructor is correct. Write a getter method for each field. IntelliJ hint (this won't be in the real test): put your cursor after the closing curly bracket of the constructor; right click, choose Generate, choose Getter, select all of the fields; click Ok. Check that the you have three getters. Do not write setters methods. Write a method called getDetails that will return the values of the fields in one of the following two formats. For example,…arrow_forwardPlease help me with this JAVA exercise. Thank youarrow_forwardThe next sections will provide you with the definitions of the classes MonthCalender and DateTimerPicker respectively.arrow_forward
- Need help with the following: Adding comments to help explain the purpose of methods, classes, constructors, etc. to help improve understanding. Implementing enhanced for loop (for each loop) to iterate over the numbers array in the calculateProduct method. Creating a separate class for the UI and a separate class for the calculator logic. Lastly, creating a constant instead of hard coding the number "5" in multiple places. Thank you for any input/knowldge you can transfer to me, I appreciate it. Source Code: package implementingRecursion; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class RecursiveProductCalculator extends JFrame { private JTextField[] numberFields; private JButton calculateButton; private JLabel resultLabel; public RecursiveProductCalculator() { setTitle("Recursive Product Calculator"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridLayout(7, 1)); numberFields = new…arrow_forwardThe goal of this coding exercise is to create two classes BookstoreBook and LibraryBook. Both classes have these attributes: author: Stringtiltle: Stringisbn : String- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like 20% off...etc). For a LibraryBook, we add the call number (that tells you where the book is in the library) as a string. The call number is automatically generated by the following procedure:The call number is a string with the format xx.yyy.c, where xx is the floor number that is randomly assigned (our library has 99 floors), yyy are the first three letters of the author’s name (we assume that all names are at least three letters long), and c is the last character of the isbn.- In each of the classes, add the setters, the getters, at least three constructors (of your choosing) and override the toString method (see samplerun…arrow_forwardCreate a new Teller Class Build a new Teller class(“Teller.java”). This class will be a sub-class of Employee. The Teller class should have all the same properties as an Employee as well as two new properties: hoursWorked and shift. HoursWorked is the total hours that this teller worked this week, and shift is either “day” or “evening” shift. Make sure to add 2 constructors and a display() method. Do not include a main() method in the Teller class. Test out this Teller class, by building a separate Tester class called TellerTester stored in a new file called ( “TellerTester.java”. This TellerTester class will only have a main() method, that will instantiate a Teller object, pass all the data to the Teller constructor, and lastly call the Teller display() method; just these 3 lines.arrow_forward
- Override the testOverriding() method in the Triangle class. Make it print “This is theoverridden testOverriding() method”. (add it to triangle class don't make a new code based on testOverriding in SimpleGeometricObject class.) (this was the step before this one) Add a void testOverriding() method to the SimpleGeometricObject class. Make it print“This is the testOverriding() method of the SimpleGeometricObject class”arrow_forwardFor this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forwardA getPaycheck method that implements the same method in the Employee class. Itcalculates the paycheck amount (m_hours × m_hourlyWage) and returns the studentworker’s paycheck as a string in the following format (Note that the three fields areseparated by a hyphen, represented by the minus character. Do NOT add spaces beforeor after each hyphen. There are no commas in the number.):arrow_forward
- Please help me with this using java. Explain each line of code with comments. Please make sure that the code works.arrow_forwardNumber 11arrow_forwardBased on the information in the screenshots, please answer the following question below. The language used is Java and please provide the code for it with the explanation. Create a testing class named “PlanTest”. Under this class, there needs to be another static method besides the main method: You will need to create a static method named “prerequisiteGenerator” under “PlanTest”, which return a random 2D array containing prerequisite pairs (e.g., {{1, 3}, {2, 3}, {4, 2}}). The value range of a random integer is [0, 10] (inclusive). This method will accept an integer parameter, which specifies the length of the returned array: int[][] pre = prerequisiteGenerator(3); // possibly {{1, 3}, {2, 3}, {4, 2}} Under the main method, you will need to use prerequisiteGenerator to generate random prerequisite pair lists, and perform tests on plan method at least three examples by printing proper messages. Given 4 courses and prerequisites as [[1,0], [2,0], [3,1]] It is possible to take all…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
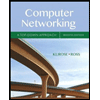
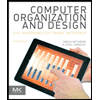
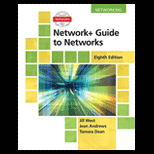
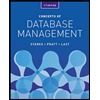
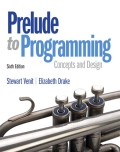
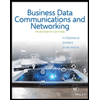