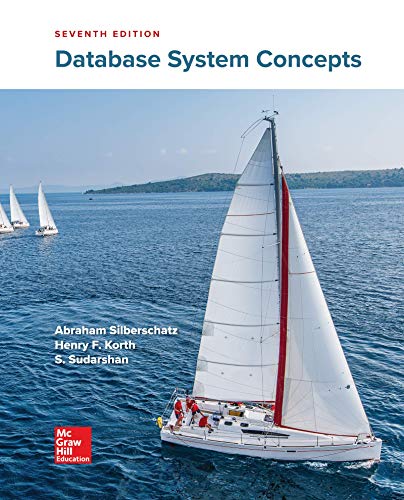
Concept explainers
Hello, I submitted this question earlier but I left out critical details. Please work problem again. Please give the final code in detail.
Implement a class KitKat in Python that represents a KitKat candy bar.. Each KitKat object will keep track of the flavor of the candy bar as well as the number of fingers (sections) remaining in the bar.
Implementation details:
- the constructor ( __init__ ) accepts two optional arguments
flavor, a str that defaults to 'Milk Chocolate'
number of fingers remaining, an int that defaults to 4. (Assume that any argument
given is non-negative, no data validation is required.)
- __repr__ returns a str version of the KitKat as formatted below, note that single quotes appear around the brand
Example of output:
>>> candy = KitKat() # using both defaults
>>> candy
KitKat('Milk Chocolate', 4)
>>> candy = KitKat('Green Tea',3) # flavor and fingers both specified
>>> candy
KitKat('Green Tea', 3)
>>> candy = KitKat('Mint') # flavor specified, fingers default to 4
>>> candy
KitKat('Mint', 4)
The eat method allows one to eat one or more fingers/sections of a KitKat . Details:
- accepts one optional argument, the number of fingers to eat
defaults to 1 if no argument is supplied
otherwise, you may assume this is a positive integer - reduces the current number of fingers by the number eaten
Any attempt to eat more fingers than the KitKat currently has, will consume only those that are there. (i.e., fingers cannot go negative) - returns a list containing copies of the flavor, one per finger that was actually eaten.
Example of output:
>>> candy = KitKat('Green Tea',4)
>>> candy.eat() # defaults to 1
['Green Tea']
>>> candy
KitKat('Green Tea', 3)
>>> candy.eat(4) # try to eat 4, but only 3 there, so eat 3
['Green Tea', 'Green Tea', 'Green Tea']
>>> candy
KitKat('Green Tea', 0)
>>> candy.eat() # nothing left to eat
[]
>>> candy
KitKat('Green Tea', 0)
Two KitKat objects are compared by comparing the number of fingers available, for these
comparisons the flavor is ignored. There are two comparisons supported:
- == - this method must be named __eq__ , it accepts two KitKat objects and returns True if and only if they currently have the same number of fingers.
- > - this method must be named __gt__ , it accepts two KitKat objects and returns True if the first KitKat has strictly more fingers than the second KitKat .
Hints/Notes:
You may not have written __gt__ before. Structurally the code is identical to __eq__ , the only difference is that the Boolean expression inside the method is different, as described above.
Example of final code output:
>>> KitKat('Green Tea', 3) == KitKat('Mint', 3)
True
>>> KitKat('Green Tea', 3) == KitKat('Mint', 2)
False
>>> KitKat('Green Tea', 3) > KitKat('Mint', 3)
False
>>> KitKat('Green Tea', 3) > KitKat('Mint', 2)
True
>>> KitKat('Green Tea', 2) > KitKat('Mint', 4)
False

Step by stepSolved in 2 steps

- solve in python Street ClassStep 1:• Create a class to store information about a street:• Instance variables should include:• the street name• the length of the street (in km)• the number of cars that travel the street per day• the condition of the street (“poor”, “fair”, or “good”).• Write a constructor (__init__), that takes four arguments corresponding tothe instance variables and creates one Street instance. Street ClassStep 2:• Add additional methods:• __str__• Should return a string with the Street information neatly-formatted, as in:Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.• compare:• This method should compare one Street to another, with the Street the method is calledon being compared to a second Street passed to a parameter.• Return True or False, indicating whether the first street needs repairs more urgently thanthe second.• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, whichneed repairs more…arrow_forwardA class object can encapsulate more than one [answer].arrow_forward-int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates +intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false +resize(double scale):void// * multiply the…arrow_forward
- Summary In this lab, you create a derived class from a base class, and then use the derived class in a Python program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Open the file named Motorcycle.py. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class __init()__ method inside the Motorcycle class's __init()__ method. In theMotorcycle class, create an attribute named sidecar. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the message in the accelerate method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named MyMotorcycleClassProgram.py. In the…arrow_forwardint x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // *** returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // *** move the center of the circle to the new coordinates +intersects(Circle other): bool //*** returns true if the center of the other circle lies inside this circle else returns false +resize(double…arrow_forwarda) Implement a class Point with three attributes, x, y, and z.b) Implement an init method with an optional parameter type: 1) Set the default value of x, y, and z to 0.c) Implement a display method to print the values of x, y, and z as the example output below.d) Instantiate two objects of type Point, one with arguments,1, 2, and 3, and the other one without any arguments.e) Call display() to print x, y, and z. Example Output(x, y, z): (1, 2, 3)(x, y, z) : (0, 0, 0)arrow_forward
- O Challenge task Write the code of the Constructors and Distructor for the following class 1-Write the code for the empty constructor, that sets X, Y to 0, and prints "Constructor 1 2-Write the code for the parameterized constructor, that takes two integers AB and assigns X A Y=B. and prints the Sentance "Constructor 2 3-Write the code for the Distructor that prints the Sentance "Distruct" followed by the value of X, Y Constraints 5 Input Format the input is two integers E. Output Format as shown in Test case Sample #1 Input 57 Output Constructor 1 Constructor 2 Constructor 1 Destruct 0,0 Destruct 5,7 Destruct 0,0arrow_forwardWhat happens if you write a class with no constructor whatsoever?arrow_forwardAg 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forward
- True or False Constructors can accept arguments in the same way as other methods.arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
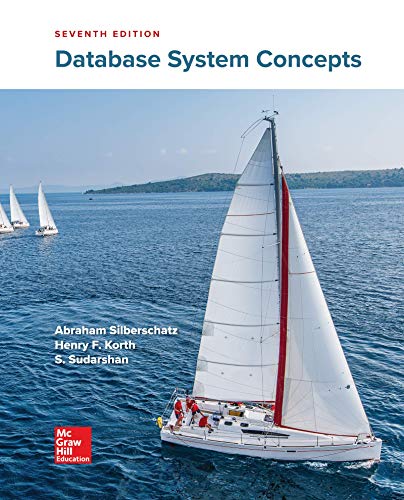
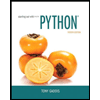
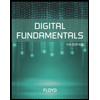
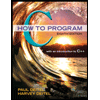
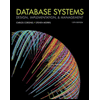
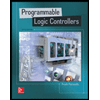