Please add the following to the code!!! - Add destructor - Add copy constructor - Overload the assignment operator - Overload the << operator to display set - Add elements in sorted way - Make search (logn)
Please add the following to the code!!! - Add destructor - Add copy constructor - Overload the assignment operator - Overload the << operator to display set - Add elements in sorted way - Make search (logn)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please add the following to the code!!!
- Add destructor
- Add copy constructor
- Overload the assignment operator
- Overload the << operator to display set
- Add elements in sorted way
- Make search (logn)
#include <iostream>
#include<set>
using namespace std;
#include<set>
using namespace std;
class Set{
private:
int* arr;
int count=0;
int capacity=10;
public:
Set(){
arr = new int[capacity];
};
void add(int);
bool search(int);
Set union_set(const Set& set2);
void display();
};
int main(){
Set set1;
Set set2;
set1.add(5); set1.add(3); set1.add(2); set1.add(4);
set2.add(7); set2.add(3); set2.add(8); set2.add(9);
Set set3 = set1+ set2;
cout << set3;
Set set1;
Set set2;
set1.add(5); set1.add(3); set1.add(2); set1.add(4);
set2.add(7); set2.add(3); set2.add(8); set2.add(9);
Set set3 = set1+ set2;
cout << set3;
//example of the set use from the std library. Set implemented using BST
set<int> myset;
set<int>::iterator it; // pointer to a node
set<int>::iterator it; // pointer to a node
// set some initial values:
myset.insert(30);
myset.insert(70);
myset.insert(90);
myset.insert(20);
myset.insert(10);
//it = myset.find(20);
//myset.erase(it);
//myset.erase(myset.find(40));
myset.insert(30);
myset.insert(70);
myset.insert(90);
myset.insert(20);
myset.insert(10);
//it = myset.find(20);
//myset.erase(it);
//myset.erase(myset.find(40));
std::cout << "myset contains:";
for (it = myset.begin(); it != myset.end(); ++it)
std::cout << ' ' << *it;
std::cout << '\n';
for (it = myset.begin(); it != myset.end(); ++it)
std::cout << ' ' << *it;
std::cout << '\n';
return 0;
}
void Set::add(int data){
if (count >= capacity){
//expend set
}
//expend set
}
if (!search(data)){
//Futute improvement: insert sorted - lots of shifting, so BST is more efficient
arr[count++] = data;
}
//Futute improvement: insert sorted - lots of shifting, so BST is more efficient
arr[count++] = data;
}
}
bool Set::search(int data){
bool Set::search(int data){
//Futute improvement: binary search but has to be sorted
for (int i = 0; i < count; i++){
if (arr[i] == data)
return true;
}
return false;
}
Set Set::union_set(const Set& set2){
Set newSet;
for (int i = 0; i < count; i++)
newSet.add(arr[i]);
for (int i = 0; i < set2.count; i++){
newSet.add(set2.arr[i]);
}
return newSet;
}
for (int i = 0; i < count; i++){
if (arr[i] == data)
return true;
}
return false;
}
Set Set::union_set(const Set& set2){
Set newSet;
for (int i = 0; i < count; i++)
newSet.add(arr[i]);
for (int i = 0; i < set2.count; i++){
newSet.add(set2.arr[i]);
}
return newSet;
}
void Set::display(){
for (int i = 0; i < count; i++)
{
cout << arr[i] << ' ';
}
cout << endl;
}
for (int i = 0; i < count; i++)
{
cout << arr[i] << ' ';
}
cout << endl;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
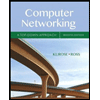
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
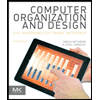
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
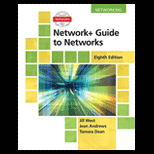
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
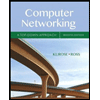
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
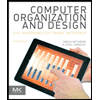
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
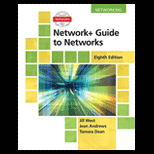
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
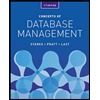
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
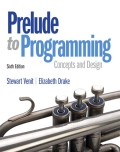
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
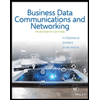
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY