Download shapes.h and renderer3.cpp from Canvas. (a) Complete the interative portion of the renderer for both rectangle and triangle.
Download shapes.h and renderer3.cpp from Canvas. (a) Complete the interative portion of the renderer for both rectangle and triangle.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++
shapes.h:
#include <iostream>
#include <cmath>
struct Point2d
{
double x;
double y;
void print()
{
// example: (2.5,3.64)
std::cout << "(" << x << "," << y << ")" << std::endl;
}
double length() const
{
return std::sqrt(x * x + y * y);
}
// const Point2D & ==> function can't modify 'other'
// the second const ==> function can't modify x, y
Point2d add(const Point2d &other) const
{
Point2d result;
result.x = x + other.x;
result.y = y + other.y;
return result;
}
};
// example of an interface
struct Shape
{
virtual bool contains(Point2d p) = 0;
};
struct Circle : Shape
{
Point2d center;
double radius;
bool contains(Point2d p)
{
Point2d diff;
diff.x = p.x - center.x;
diff.y = p.y - center.y;
double distToCenter = diff.length();
return distToCenter <= radius;
}
};
// TODO: replace this with your Rectangle implementation
struct Rectangle : Shape
{
// TODO: which member variables to model a rectangle?
bool contains(Point2d p)
{
return false; // TODO
#include <cmath>
struct Point2d
{
double x;
double y;
void print()
{
// example: (2.5,3.64)
std::cout << "(" << x << "," << y << ")" << std::endl;
}
double length() const
{
return std::sqrt(x * x + y * y);
}
// const Point2D & ==> function can't modify 'other'
// the second const ==> function can't modify x, y
Point2d add(const Point2d &other) const
{
Point2d result;
result.x = x + other.x;
result.y = y + other.y;
return result;
}
};
// example of an interface
struct Shape
{
virtual bool contains(Point2d p) = 0;
};
struct Circle : Shape
{
Point2d center;
double radius;
bool contains(Point2d p)
{
Point2d diff;
diff.x = p.x - center.x;
diff.y = p.y - center.y;
double distToCenter = diff.length();
return distToCenter <= radius;
}
};
// TODO: replace this with your Rectangle implementation
struct Rectangle : Shape
{
// TODO: which member variables to model a rectangle?
bool contains(Point2d p)
{
return false; // TODO
}
};
// https://stackoverflow.com/questions/2049582/how-to-determine-if-a-point-is-in-a-
2d-triangle?
double sign(Point2d p1, Point2d p2, Point2d p3)
{
return (p1.x - p3.x) * (p2.y - p3.y) - (p2.x - p3.x) * (p1.y - p3.y);
}
struct Triangle : Shape
{
Point2d v1;
Point2d v2;
Point2d v3;
bool contains(Point2d p)
{
double d1, d2, d3;
bool has_neg, has_pos;
d1 = sign(p, v1, v2);
d2 = sign(p, v2, v3);
d3 = sign(p, v3, v1);
has_neg = (d1 < 0) || (d2 < 0) || (d3 < 0);
has_pos = (d1 > 0) || (d2 > 0) || (d3 > 0);
return !(has_neg && has_pos);
}
};
// TODO: add another letter here and in the Sprite constructor
bool letter_A[5][3] = {{0, 1, 0}, {1, 0, 1}, {1, 1, 1}, {1, 0, 1}, {1, 0, 1}};
bool letter_B[5][3] = {{1, 1, 0}, {1, 0, 1}, {1, 1, 0}, {1, 0, 1}, {1, 1, 0}};
bool letter_C[5][3] = {{0, 1, 0}, {1, 0, 1}, {1, 0, 0}, {1, 0, 1}, {0, 1, 0}};
struct Sprite : Shape
{
bool image[5][3] = {};
Point2d topLeft;
Sprite(Point2d p, char ch)
{
topLeft = p;
// copy the appropriate array into this Sprite instance
// (this is kind of messy... we can't simply write "image = letter_A;",
unfortunately)
switch (ch)
{
case 'a':
case 'A':
std::copy(&letter_A[0][0], &letter_A[0][0]+15,&image[0][0]);
break;
case 'b':
case 'B':
std::copy(&letter_B[0][0], &letter_B[0][0]+15,&image[0][0]);
break;
};
// https://stackoverflow.com/questions/2049582/how-to-determine-if-a-point-is-in-a-
2d-triangle?
double sign(Point2d p1, Point2d p2, Point2d p3)
{
return (p1.x - p3.x) * (p2.y - p3.y) - (p2.x - p3.x) * (p1.y - p3.y);
}
struct Triangle : Shape
{
Point2d v1;
Point2d v2;
Point2d v3;
bool contains(Point2d p)
{
double d1, d2, d3;
bool has_neg, has_pos;
d1 = sign(p, v1, v2);
d2 = sign(p, v2, v3);
d3 = sign(p, v3, v1);
has_neg = (d1 < 0) || (d2 < 0) || (d3 < 0);
has_pos = (d1 > 0) || (d2 > 0) || (d3 > 0);
return !(has_neg && has_pos);
}
};
// TODO: add another letter here and in the Sprite constructor
bool letter_A[5][3] = {{0, 1, 0}, {1, 0, 1}, {1, 1, 1}, {1, 0, 1}, {1, 0, 1}};
bool letter_B[5][3] = {{1, 1, 0}, {1, 0, 1}, {1, 1, 0}, {1, 0, 1}, {1, 1, 0}};
bool letter_C[5][3] = {{0, 1, 0}, {1, 0, 1}, {1, 0, 0}, {1, 0, 1}, {0, 1, 0}};
struct Sprite : Shape
{
bool image[5][3] = {};
Point2d topLeft;
Sprite(Point2d p, char ch)
{
topLeft = p;
// copy the appropriate array into this Sprite instance
// (this is kind of messy... we can't simply write "image = letter_A;",
unfortunately)
switch (ch)
{
case 'a':
case 'A':
std::copy(&letter_A[0][0], &letter_A[0][0]+15,&image[0][0]);
break;
case 'b':
case 'B':
std::copy(&letter_B[0][0], &letter_B[0][0]+15,&image[0][0]);
break;
case 'c':
case 'C':
std::copy(&letter_C[0][0], &letter_C[0][0]+15,&image[0][0]);
break;
default:
break; // image = {}, i.e. blank image
}
}
bool contains(Point2d p)
{
Point2d diff = {p.x - topLeft.x, p.y - topLeft.y};
if(0 <= diff.x && diff.x < 3)
{
if(0 <= diff.y && diff.y < 5)
{
// p is in Sprite's rectangle...
// so we check... does the Sprite have 0 or 1 here?
return image[(int)diff.y][(int)diff.x];
}
}
// p is not in Sprite's rectangle
return false;
}
};
case 'C':
std::copy(&letter_C[0][0], &letter_C[0][0]+15,&image[0][0]);
break;
default:
break; // image = {}, i.e. blank image
}
}
bool contains(Point2d p)
{
Point2d diff = {p.x - topLeft.x, p.y - topLeft.y};
if(0 <= diff.x && diff.x < 3)
{
if(0 <= diff.y && diff.y < 5)
{
// p is in Sprite's rectangle...
// so we check... does the Sprite have 0 or 1 here?
return image[(int)diff.y][(int)diff.x];
}
}
// p is not in Sprite's rectangle
return false;
}
};

Transcribed Image Text:Download shapes.h and renderer3.cpp from Canvas.
(a) Complete the interative portion of the renderer for both rectangle and triangle.
(b) The renderer can currently draw letters 'A', 'B' and 'C'. Extend this to one addi-
tional letter.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

Recommended textbooks for you
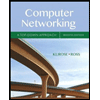
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
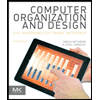
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
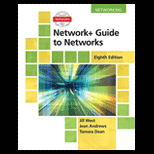
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
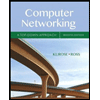
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
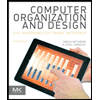
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
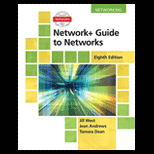
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
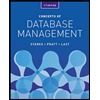
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
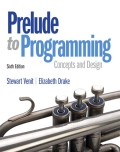
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
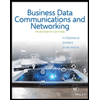
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY