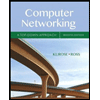
no magic number
public class GolfClubMember
{
// Constants for driving range basket costs
public static double SMALL_BASKET_COST = 7.0;
public static double MIDIUM_BASKET_COST = 11.0;
public static double LARGE_BASKET_COST = 15.0;
// Constants for game costs
public static double TWILIGHT_GAME_COST = 15.0;
public static double REGULAR_GAME_COST = 17.0;
// Step 2: Declare two instance variables
// name: String
// balance: double
// Step 3: Write the following constructor
// according to the description
/**
* The constructor has two parameters to initialize
* the instance variables.
*
* @param nameParam the name of this golf club member
* @param balanceParam the balance of this golf club member
*/
// Step 4: Override method toString() according to the description
/**
* Gets a string representaton for this golf club member in the
* following format:
* GolfClubMember[Name:Mike,Balance:390.0]
*
* @return a string containing the name and balance
* of this golf club member
*/
@Override
// Step 5: Write method drivingRange() according to the description
/**
* When you go to a golf driving range, you need to pay to get
* a basket of golf balls.
*
* The method has one parameter for the size of the basket and
* it reduces the balance of this golf club member based on
* the basket size.
* There are three different sizes, "small", "medium", and
* "large", but anything other than "small" or "medium" will
* be considered "large".
* The cost is SMALL_BASKET_COST, MIDIUM_BASKET_COST
* and LARGE_BASKET_COST, respectively.
*
* @param size the size of the basket.
*/
// Step 6: Write method playingGame() according to the description
/**
* When you play a golf game, you pay for the game to play.
*
* The method has one parameter for the type of game to play
* and it reduces the balance of this golf club member based
* on the game type.
* The type is either "regular" or "twilight", but anything
* not "regular" will be considered as "twilight".
* The cost is REGULAR_GAME_COST and TWILIGHT_GAME_COST,
* respectively.
*
*
*/
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 4 images

- // This class discounts prices by 10% public class DebugFour4 { public static void main(String args[]) { final double DISCOUNT_RATE = 0.90; int price = 100; double price2 = 100.00; tenPercentOff(price DISCOUNT_RATE); tenPercentOff(price2 DISCOUNT_RATE); } public static void tenPercentOff(int p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println("Ten percent off " + p); System.out.println(" New price is " + newPrice); } public static void tenPercentOff(double p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println"Ten percent off " + p); System.out.println(" New price is " + newprice); } }arrow_forwardCode writing challenge activity demo 462500.3140334.qx3zqy7 1 temperatures 2 3 4 5 { 'Seattle': 56.5, Run = 'New York': float(input()), 'Kansas City': 81.9, 'Los Angeles': 76.5 6} 7 8 if 'New York' in temperatures: 9 10 11 12 13 else: 14 15 if temperatures ['New York'] > 90: print('The city is melting!') print('The temperature in New York is', temperatures ['New York']) print('The temperature in New York is unknown.' else: X Not all tests passed ✓ Testing with input: 105 Your output The city is melting! X Testing with input: 50 Output differs. See highlights below. Your output The temperature in New York is 50.0 Expected output The temperature in New York is 50.0. A Рarrow_forwardDigital communication classarrow_forward
- class Bug { private final int orgPosition = 0; private int currentPosition; private boolean direction; public Bug() { direction = true; currentPosition = orgPosition; } public void move() { if (direction) { ++currentPosition; } else { --currentPosition; } } public void turn() { direction = !direction; } public int getPosition() { return currentPosition; } public boolean getDirection() { return direction; } public String toString() { StringBuffer sb = new StringBuffer(); sb.append("Position = " + currentPosition + ", Direction = "); sb.append(direction ? "RIGHT" : "LEFT"); return sb.toString(); } } -------------------------------------TestDriver.java------------------------------------- import java.util.Scanner; class TestDriver { public static void main(String args[]) { Scanner input = new Scanner(System.in); Bug b…arrow_forwardHelp, I making a elevator simulator. Can someone please help me improve this code I have. The remaining code is in the pictures. Any help is appreciated. Thank You! The simulation should have 4 types of Passengers: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. The simulation should also have 4 types of Elevators: StandardElevator: This is the most common type of elevator…arrow_forwardWrite the JAVA code for the following UML Diagram Account -ownerName : String -balance : double -accountNumber : String +inputDetails(): void +displayDetails(0: void +depositAmount(amount: double) +withdrawAmount(amount: double) SavingAccount CurrentAccount -rateOflnterest: double |-overDraftLimit: double +inputDetails(): void +showDetails(: void +getinputDetails(): void +displayDetails(): voidarrow_forward
- Help me Complete the class definition and implementation for class task.h and task.cpp: Example: class Task { private: string name; DateTime startDate; DateTime endDate; int status; // value =1 means DONE! and value = 0 is pending }arrow_forward1 import java.util.Scanner; 2 3 public class RedBalloon { public static void main (String 0 args) { Scanner scnr = new Scanner(System.in); boolean isRed; boolean isBalloon; 4 5 6 7 8 isRed = scnr.nextBoolean(); isBalloon = scnr.nextBoolean(); 9 10 11 12 13 } 15 } 14arrow_forwardPythonarrow_forward
- Computational thinking for a software developer/computer programmer is a critical skill that is consistently applied. This lab requires you to develop a solution using Java object-oriented programming that simulates creating and managing a collection of songs. The system manages information about a collection of songs. The system stores the following information (for each attribute, choose any type that you think is appropriate--you must be able to justify the decisions you make): Collection Name: the name for the collections of songs Collection list of songs: the system allows you to create a collection with unlimited songs. You can add the same song multiple times to any given collection. Hence, the collection allows songs to be duplicated Note: other system functionalities can be inferred from the given JUnit test. o NoSystem.out.printlnstatementsshouldappearinit.o NoScanneroperations(e.g.,input.nextInt())shouldappearinit. Instead, declare the method's input parameters as indicated…arrow_forwardPLEASE ANSWER ALL QUESTIONS ON THE NEXT PAGE: class bookType { public: void setBookTitle(string s); //sets the bookTitle to s void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter void setBookPrice(double cost); //sets the private member bookPrice to cost void setCopiesInStock(int noOfCopies); //sets the private member copiesInStock to noOfCopies void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the copiesInStock string getBookISBN() const; //returns the bookISBN double getBookPrice() const; //returns the bookPrice int showQuantityInStock() const; //returns the quantity in stock void updateQuantity(int addBooks); //adds addBooks to the quantityInStock, so that the quantity in stock now has it original // value plus the parameter sent to this function private: string…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
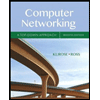
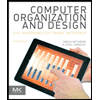
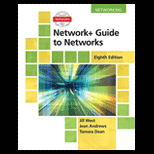
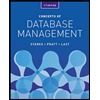
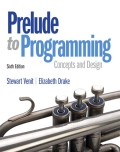
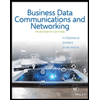