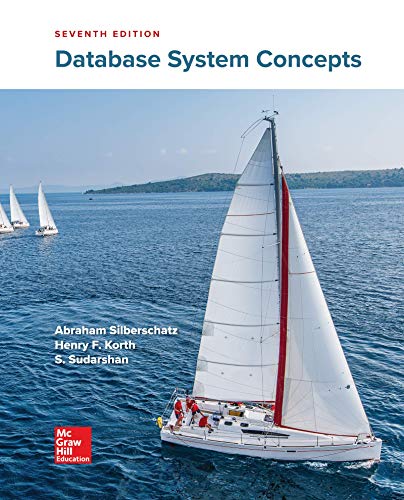
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.util.Scanner;
public class romeoJuliet {
public static void main(String[]args) throws Exception{
Scanner keyboard=new Scanner(System.in);
System.out.println("Enter a filename");
String fN=keyboard.nextLine();
int wLC=0, lC=0;
String sW= keyboard.nextLine();
File file=new File(fN);
String [] wordSent=null;
FileReader fO=new FileReader(fN);
BufferedReader buff= new BufferedReader(fO);
String sent;
while((sent=buff.readLine())!=null){
if(sent.contains(sW)){
wLC++;
}
lC++;
}
System.out.println(fN+" has "+lC+" lines");
System.out.println("Enter some text");
System.out.println(wLC+" line(s) contain \""+sW+"\"");
fO.close();
}
}
Need to adjust code so that it reads all of the words that it was tasked to find. for some reason it isn't finding all of the word locations in a text.
for example the first question is to find "the" in romeo and juliet which should have 1137, but it's only picking up 1032?

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- you must create the data files with a text tool, such as Notepad exampleThree In the while loop, this program reads fileLoop.txt until all items are read package hasnext; import java.util.Scanner; import java.io.*; // public class Hasnext { public static void main(String[] args) throws IOException { File loopfile = new File("fileLoop.txt"); Scanner getAll = new Scanner( loopfile ); // connect a Scanner to the file int num = 0, square = 0; while(getAll.hasNextInt()) { num = getAll.nextInt(); square = num * num ; System.out.println("The square of " + num + " is " + square); } getAll.close(); } }arrow_forwardPlease help me fix my errors in Python. def read_data(filename): try: with open(filename, "r") as file: return file.read() except Exception as exception: print(exception) return None def extract_data(tags, strings): data = [] start_tag = f"<{tags}>" end_tag = f"</{tags}>" while start_tag in strings: try: start_index = strings.find(start_tag) + len(start_tag) end_index = strings.find(end_tag) value = strings[start_index:end_index] if value: data.append(value) strings = strings[end_index + len(end_tag):] except Exception as exception: print(exception) return data def get_names(string): names = extract_data("name", string) return names def get_descriptions(string): descriptions = extract_data("description", string) return descriptions def get_calories(string): calories = extract_data("calories", string) return…arrow_forwardWhat is wrong with this code it creates a file Exercise17_1 but no input into the file? package randomintegers; import java.io.*;import java.util.*; public class RandomIntegers { public static void main(String[] args)throws Exception{ PrintWriter out = new PrintWriter(new FileOutputStream("Exercise17_1.txt",(true)); { for(int j=0;j<100; j++) out.print((int)(Math.random()*100000)+""); } }}arrow_forward
- FilelO 01: Write a Message Write a FileI001 class with a method named writeMessage() to takes message as a String and a filename (as a String) (in that order). Have the method, write the message to the filename. Your code will either need to 1) catch all checked exceptions or 2) use the throws keyword. public class FileI001{ public void writeMessage( String message, String filename ){arrow_forwardSelect all true statements import java.io.File; import java.util.Scanner; public class DirectorySize { public static void main(String[] args) { // Prompt the user to enter a directory or a file System.out.print("Enter a directory or a file: "); Scanner input = new Scanner(System.in); String directory = input.nextLine(); // Display the size System.out.println(getSize(new File(directory)) + " bytes"); } public static long getSize(File file) { long size = 0; // Store the total size of all files if (file.isDirectory()) { File[] files = file.listFiles(); for (int i = 0; files != null && i < files.length; i++) { size += getSize(files[i]); // Recursive call } } else { // Base case size += file.length(); } return size; } } a. The length() method returns the size of a file. b. The listFiles() method returns an array of File objects and excludes each directory.…arrow_forward-Add a method to the tractor class to write all of atractors attributes to a text file. Name it saveData(Stringfilename) It should throw Exceptions.-Add a method to the tractor class to read all of atractors attributes from a text file. Name itloadData(String filename) It should throw Exceptions.-Test these methods by calling them from your testdrivers main() method public class Tractor { private String ID; private double rentalRate; private int rentalDays; Tractor() { this.ID = "00"; this.rentalDays = 0; this.rentalRate = 0; } } Tractor(String ID, double rentalRate, int rentalDays) { this.setID(ID); this.setRentalDays(rentalDays); this.setRentalRate(rentalRate); } /** * @return the iD */ public String getID() { return ID; } /** * @return the rentalDays */ public int getRentalDays() { return rentalDays; } /** * @return the rentalRate */…arrow_forward
- I need help with this code !! import java.util.Arrays;import java.util.Scanner; public class MaxElement {public static void main(String[] args) { //create an object for Scanner class Scanner x = new Scanner (System.in);System.out.print ("Enter 10 integers: ");// create an arrayInteger[] arr = new Integer[10]; // Execute for loopfor (int i = 0; i < arr.length; i++) {//get the 10 integersarr[i] = x.nextInt(); } // Print the maximum numberSystem.out.print("The max number is = " + max(arr));System.out.print("\n"); } //max method public static <E extends Comparable<E>> E max(E[] arr) {E max = arr[0]; // Execute for loop for (int i = 1; i < arr.length; i++) { E element = arr[i];if (element.compareTo(max) > 0) {max = element;}}return max; }}arrow_forwardhelp me rewrite the code without using bufferReader pleasearrow_forwardpublic static void connectToInputFile() {Scanner inputStream = null;String inputFileName = getFileName("Enter name of input file: ");try{inputStream = new Scanner(new File("SortedA.txt"));}catch(FileNotFoundException e){System.out.println("File " + inputFileName + " not found. ");}catch(IOException e){System.out.println("Error opening input file: " + inputFileName);}}private static String getFileName(String prompt){String fileName = null;System.out.println(prompt);Scanner keyboard = new Scanner (System.in);fileName = keyboard.next();return fileName;}public static void connectToOutputFile(){String outputFileName = getFileName("Enter name of output file: ");try{outputStream = new ObjectOutputStream(new FileOutputStream("SortedArray.csv"));}catch(IOException e){System.out.println("Error opening output file" + outputFileName);System.out.println(e.getMessage());}}public static void closeFiles(){try{inputStream.close();outputStream.close();}catch(IOException e){System.out.println("Error…arrow_forward
- How do I match the output? Code: import java.io.File; import java.util.ArrayList; import java.util.Scanner; public class Assignment08 { public static void main(String[] args) throws Exception { File inputData = new File("input05.txt"); Scanner input = new Scanner(inputData); int totalNumber = input.nextInt(); Friend.friends = new Friend[totalNumber]; input.nextLine(); for (int i = 0; i < totalNumber; i++) { String name = input.nextLine(); String phone = input.nextLine(); String age = input.nextLine(); int ageNum = Integer.parseInt(age); Friend.friends[i] = new Friend(name, phone, ageNum); } printContacts(Friend.friends); Friend.showTotalFriendAge(); ManageDuplicate(Friend.friends); printContacts(Friend.friends); Friend.showTotalFriendAge(); } public static void printContacts(Friend[] contacts) {…arrow_forwardJava Program ASAP Modify this program so it passes the test cases in Hypergrade. Also change the public class to FileSorting import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program } else { String filePath = new File("").getAbsolutePath() + "/" + input; File file = new File(filePath); if (file.exists() && !file.isDirectory()) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { String st; StringBuilder formattedText = new StringBuilder(); while ((st = br.readLine()) != null)…arrow_forwardpackage lab06;;public class gradereport { public static void main(String[] args) { Scanner in = new Scanner(System.in);double[] Scores = new double[10]; for(int i=0;i<10;i++){ System.out.println("Enter score " + (i+1));scores[i]=in.nextdouble(); } for(int i=0;i<10;i++){ if (scores[i] >=80) System.out.println("Score " + (i+1) + " receives a grade of HD"); else if (scores[i]>=70) System.out.println("Score " + (i+1) + " receives a grade of D"); else if (scores[i] >=60) System.out.println("Score "+ (i+1) + " receives a grade of C"); else if (scores[i] >=50) System.out.println("Score " + (i+1) + " receives a grade of P"); else if (scores[i] >=40) System.out.println("Score " + (i+1) + " receives a grade of MF"); else if (scores[i] >=0) System.out.println("Score " +…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
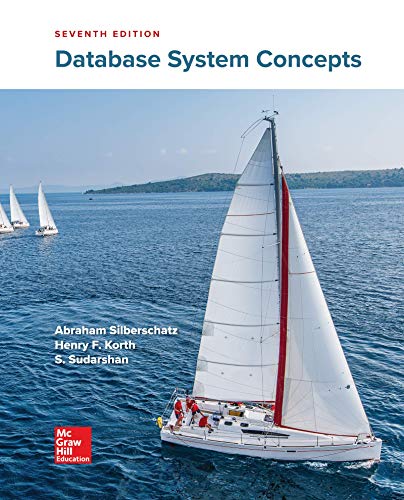
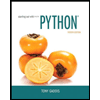
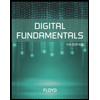
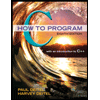
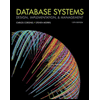
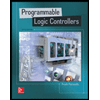