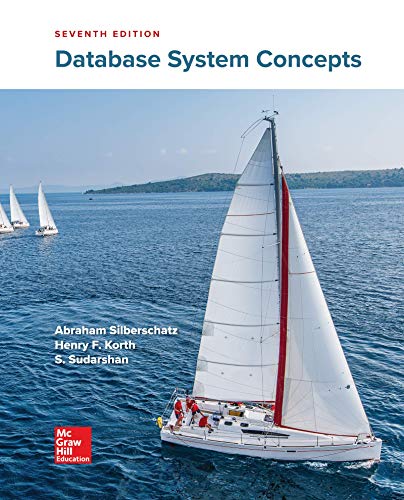
Concept explainers
I have this code so far
import java.util.Comparator; import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { super(); this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin; } public void setVin(String vin) { this.vin = vin; } } class CarComparator implements Comparator<Car> { @Override public int compare(Car car1, Car car2) { return car1.getManufacturer().compareTo(car2.getManufacturer()); } } public class OldestCarsByMake { public static void main(String[] args) throws FileNotFoundException { ArrayList<Car> cars = new ArrayList<Car>(); Scanner sc1 = new Scanner(System.in); System.out.println("Enter filename"); String carFile = sc1.next(); File file = new File(carFile); Scanner sc2 = new Scanner(file); String[] carDetails = new String[4]; sc2.nextLine(); //Skipping header while (sc2.hasNext()) { String carLine = sc2.nextLine(); carDetails = carLine.split("\\s\\s\\s"); String manufacturer = carDetails[1]; String model = carDetails[2]; int year = Integer.parseInt(carDetails[3]); String vin = carDetails[4]; boolean flag = true; for (Car c : cars) { if (c.manufacturer.equals(manufacturer)) { flag = false; if (c.year > year) { c.setModel(model); c.setYear(year); c.setVin(vin); } else if (c.year == year) { if ((c.vin).compareTo(vin) > 0) { c.setModel(model); c.setYear(year); c.setVin(vin); } } } } if (flag) cars.add(new Car(manufacturer, model, year, vin)); } Collections.sort(cars, new CarComparator()); int spaces = 25; for (Car c : cars) { for (int i = 0; i < 20 - (c.manufacturer.length()); i++) System.out.print(" "); System.out.print(c.manufacturer); for (int i = 0; i < 20 - (c.model.length()); i++) System.out.print(" "); System.out.print(c.model); System.out.println(" " + c.year + " " + c.vin); } System.out.println(cars.size() + " result(s)"); } }
however it produces an error
Enter filename\n
car-list-3.txtENTER
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1\n
\tat OldestCarsByMake.main(OldestCarsByMake.java:69)\n
it is supposed to display
Enter filename\n
car-list-3.txtENTER
Oldest cars by make\n
Acura Legend 1988 YV1672MK8A2784103\n
Aptera Typ-1 2009 19UUA56952A698282\n
Aston Martin DB9 2006 JTDJTUD36ED662608\n
Audi 5000S 1985 JN1CV6EK1CM209730\n
Austin Mini Cooper 1964 1G6KD57Y86U095255\n
Bentley Continental GT 2006 19UUA655X4A245718\n
BMW 7 Series 1993 1FM5K7B80FG198521\n
Buick LeSabre 1986 5FRYD4H82EB970786\n
Cadillac Fleetwood 1992 WAUSVAFA0AN244068\n
Chevrolet Corvette 1957 WBA3V7C57F5095471\n
Chrysler Town & Country 1994 WBANU53558B610150\n
Daewoo Lanos 1999 2HNYD18412H169358\n
Daihatsu Charade 1992 JH4CL95958C112754\n
Dodge D150 1993 19XFA1F37BE391950\n
Eagle Talon 1993 WUAAU34289N943973\n
Ferrari F430 Spider 2006 5N1AR2MM5EC533806\n
Fiat 500 2012 1G6DL67A880617705\n
Ford Thunderbird 1965 1HGCR2F32DA757676\n
Geo Metro 1992 1G6KE54Y34U777569\n
GMC Suburban 1500 1992 19XFB2F59CE612826\n
Holden VS Commodore 1995 WAUBGBFC7CN714505\n
Honda Civic 1980 JTDKN3DU8A0870976\n
Hummer H1 1999 5FNRL5H20BB474763\n
Hyundai Sonata 2002 JA4AS2AW2DU975492\n
Infiniti Q 1994 WAUDH78E97A027816\n
Isuzu Impulse 1992 WBALM5C5XAE656665\n
Jaguar XJ Series 1994 1FMJK1HT9FE090336\n
Jeep Wrangler 1992 WA1AY94L38D656200\n
Kia Sephia 2001 3GYFNCEY3BS991891\n
Lamborghini Countach 1986 SCBGU3ZA2EC356135\n ............
56 result(s)\n
can an expert help explain what's causing this error and fix my code to produce the correct output that is pulled from a user entered .txt file

Step by stepSolved in 3 steps

- java program For this question, the server contains the id, name and cgpa of some Students in a file. The client will request the server for specific data, which the server will provide to the client. Server: The server contains the data of some Students. For each student, the server contains id, name and cgpa data in a file. Here's an example of the file: data.txt101 Saif 3.52201 Hasan 3.81.... Create a similar file in you server side. The file should have at least 8 students. The client will request the server to provide the details (id, name, cgpa) of the Nth highest cgpa student in the file. If N's value is 1, the server will return the details of the student with the highest cgpa. if N's value is 3, the server will return the details of the student with the third highest cgpa. If N's value is incorrect, the server returns to the client: "Invalid request". Client: The client sends the server the value of N, which is an integer number. The client takes input the value of N…arrow_forwardwrite in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardLoad the airline JSON file into a python dictionary, save it to a text file and print the airlines that operated in Boston and SanFrancisco airports during the month of June.arrow_forward
- Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. I am having trouble with my code here is my code import java.util.Comparator;import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Collections;import java.util.Scanner;class Car {String manufacturer;String model;int year;String vin;public Car(String manufacturer, String model, int year, String vin) {super();this.manufacturer = manufacturer;this.model = model;this.year = year;this.vin = vin;}public String getManufacturer() {return manufacturer;}public void setManufacturer(String manufacturer) {this.manufacturer = manufacturer;}public String getModel() {return model;}public void setModel(String model) {this.model = model;}public int getYear() {return year;}public void setYear(int year) {this.year = year;}public String getVin() {return vin;}public void setVin(String vin) {this.vin = vin;}}class…arrow_forwardA WriteOnly attribute may be implemented automatically. True vs. Falsearrow_forwardFocus on string operations and methods You work for a small company that keeps the following information about its clients: • first name • last name • a 5-digit user code assigned by your company. The information is stored in a file clients.txt with the information for each client on one line (last name first), with commas between the parts. For example Jones, Sally,00345 Lin ,Nenya,00548 Fule,A,00000 Smythe , Mary Ann , 00012 Your job is to create a program assign usernames for a login system. First: write a function named get_parts(string) that will that will receive as its arguments a string with the client data for one client, for example “Lin ,Nenya,00548”, and return the separate first name, last name, and client code. You should remove any extra whitespace from the beginning and newlines from the end of the parts. You’ll need to use some of the string methods that we covered in this lesson You can test your function by with a main() that is just the function call with the…arrow_forward
- Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. I am having trouble with a specific line of my code here is my code import java.util.Comparator; import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; class Car { String manufacturer; String model; int year; String vin; public Car(String manufacturer, String model, int year, String vin) { super(); this.manufacturer = manufacturer; this.model = model; this.year = year; this.vin = vin; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getVin() { return vin;…arrow_forwardcreate a class Person to represent a person and another one to represent the database. A dictionary should be a data member of the database class, with Person objects as search keys for this dictionary. Write a program that tests and demonstrates your database.arrow_forwardCreate a datafile that contains the first name, last name, gender, age, height, smoking preference, eye color and phone number. Add a variety of records to the file. A sample file looks like:  Write a program that opens the file and reads the records one by one. The program will skip any records where the gender preference is not a match. Of those records that match the gender preference, check to see if the age and height are between the maximum and minum preferences. Then check to see if the smoking preference and eye color are also a match. If at least 3 of the remaining fields match, consider the record a partial match, and print it in the report. If all 4 of the remaining fields match, the record is a perfect match and print it in the report with an asterisk next to it. At the end of the program, close the file and report how many total records there were of the specified gender, how many were a partial match, and how many were a perfect match.arrow_forward
- Ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. i am having trouble getting my code to work this is my code: import java.util.*;import java.io.*;class Car {String manufacturer;String model;int year;String vin;public Car(String manufacturer, String model, int year, String vin) {super();this.manufacturer = manufacturer;this.model = model;this.year = year;this.vin = vin;}public String getManufacturer() {return manufacturer;}public void setManufacturer(String manufacturer) {this.manufacturer = manufacturer;}public String getModel() {return model;}public void setModel(String model) {this.model = model;}public int getYear() {return year;}public void setYear(int year) {this.year = year;}public String getVin() {return vin;}public void setVin(String vin) {this.vin = vin;}}class CarComparator implements Comparator < Car > {@Overridepublic int compare(Car car1, Car car2) {int yearCompare =…arrow_forwardUsing Go, return a Movie with the given title and genre. The Views, Likes, and Dislikes should all be left in their zero-vale states. type Movie struct { Title string Genre string Likes int Dislikes int Views int } func NewMovie(title, genre string) *Movie { return &Movie { } }arrow_forwardThe following sample file called grades.txt contains grades for several students in an imaginary class. Each line of this file stores a student's name followed by their exam scores. The number of scores might be different for each student. abdul 10 15 20 30 40erica 23 16 19 22haroon 8 22 17 14 32 17 24 21 2 9 11 17omar 12 28 21 45 26 10chengjie 14 32 25 16 89alex 15 22 11 35 36 7 9bryan 34 56 11 29 6laxman 24 23 9 45 27 Now, consider the following code. f = open("grades.txt", "r") for aline in f: items = aline.split() # choose your option for this part f.close() Which option prints out only the names of each student in stored in grades.txt? Question 11 options: print(items[0:]) print(items[0]) print(items[0][0])arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
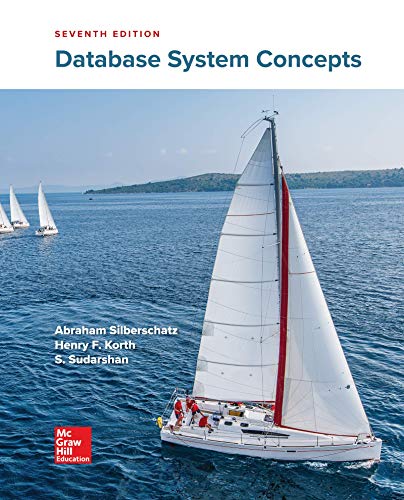
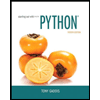
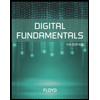
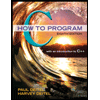
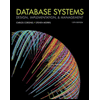
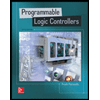