Java Program ASAP Here is a FileSorting program to read a CSV file, sorts a list of integers on each row, and prints a comma separated list of sorted integers on the console. Modify ir by removing or changing the main method os it passes all the test cases. import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; public class FileSorting { private static final String FILENAME = "somefile.txt"; public static void main(String[] args) { // read integers from input file Scanner fileReader; ArrayList numbers = new ArrayList<>(); try { fileReader = new Scanner(new File(FILENAME)); while(fileReader.hasNextLine()) { numbers.add(Integer.parseInt(fileReader.nextLine().trim())); } fileReader.close(); }catch(FileNotFoundException fnfe){ System.out.println("File " + FILENAME + " is not found."); } // check whether the file is empty or not if(numbers.isEmpty()) { System.out.println("File " + FILENAME + " is empty."); return; } System.out.println("ORIGINAL LIST: " + numbers); // sort the arraylist sort(numbers); // print the arraylist System.out.println("SORTED LIST: " + numbers); } // this method takes an arraylist of integers as parameter and sorts the list in ascending order private static void sort(ArrayList nums) { int i, j, temp; for(i = 0; i < (nums.size() - 1); i++) { for(j = 0; j < (nums.size() - i - 1); j++) { if(nums.get(j) > nums.get(j + 1)) { temp = nums.get(j); nums.set(j, nums.get(j + 1)); nums.set(j + 1, temp); } } } } } input2x2.csv -67,-11 -27,-70 input1.csv 10 input10x10.csv 56,-19,-21,-51,45,96,-46 -27,29,-58,85,8,71,34 50,51,40,50,100,-82,-87 -47,-24,-27,-32,-25,46,88 -47,95,-41,-75,85,-16,43 -78,0,94,-77,-69,78,-25 -80,-31,-95,82,-86,-32,-22 68,-52,-4,-68,10,-14,-89 26,33,-59,-51,-48,-34,-52 -47,-24,80,16,80,-66,-42 input0.csv Test Case 1 Please enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2x2.csvENTER -67,-11\n -70,-27\n Test Case 3 Please enter the file name or type QUIT to exit:\n input10x10.csvENTER -51,-46,-21,-19,45,56,96\n -58,-27,8,29,34,71,85\n -87,-82,40,50,50,51,100\n -47,-32,-27,-25,-24,46,88\n -75,-47,-41,-16,43,85,95\n -78,-77,-69,-25,0,78,94\n -95,-86,-80,-32,-31,-22,82\n -89,-68,-52,-14,-4,10,68\n -59,-52,-51,-48,-34,26,33\n -66,-47,-42,-24,16,80,80\n Test Case 4 Please enter the file name or type QUIT to exit:\n input0.csvENTER File input0.csv is empty.\n Test Case 5 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 6 Please enter the file name or type QUIT to exit:\n quitENTER Test Case 7 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n QUITENTER
Java Program ASAP
Here is a FileSorting program to read a CSV file, sorts a list of integers on each row, and prints a comma separated list of sorted integers on the console. Modify ir by removing or changing the main method os it passes all the test cases.
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
public class FileSorting {
private static final String FILENAME = "somefile.txt";
public static void main(String[] args) {
// read integers from input file
Scanner fileReader;
ArrayList<Integer> numbers = new ArrayList<>();
try
{
fileReader = new Scanner(new File(FILENAME));
while(fileReader.hasNextLine())
{
numbers.add(Integer.parseInt(fileReader.nextLine().trim()));
}
fileReader.close();
}catch(FileNotFoundException fnfe){
System.out.println("File " + FILENAME + " is not found.");
}
// check whether the file is empty or not
if(numbers.isEmpty())
{
System.out.println("File " + FILENAME + " is empty.");
return;
}
System.out.println("ORIGINAL LIST: " + numbers);
// sort the arraylist
sort(numbers);
// print the arraylist
System.out.println("SORTED LIST: " + numbers);
}
// this method takes an arraylist of integers as parameter and sorts the list in ascending order
private static void sort(ArrayList<Integer> nums)
{
int i, j, temp;
for(i = 0; i < (nums.size() - 1); i++)
{
for(j = 0; j < (nums.size() - i - 1); j++)
{
if(nums.get(j) > nums.get(j + 1))
{
temp = nums.get(j);
nums.set(j, nums.get(j + 1));
nums.set(j + 1, temp);
}
}
}
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER

Step by step
Solved in 4 steps with 3 images

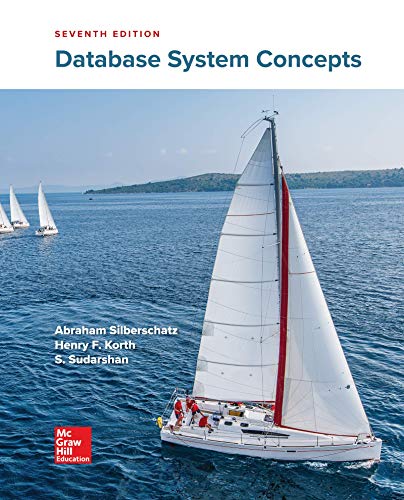
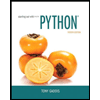
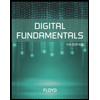
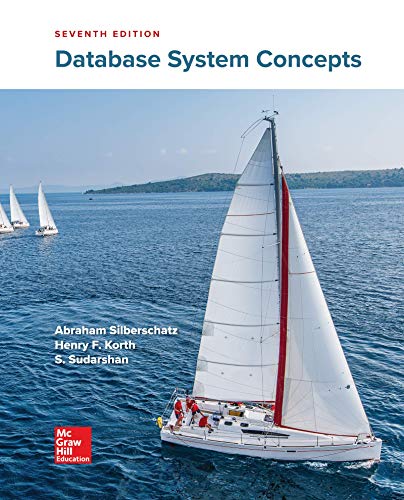
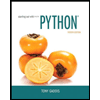
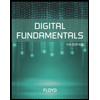
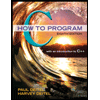
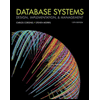
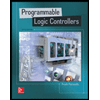