Java Program ASAP Modify this program so it passes the test cases in Hypergrade. Also change the public class to FileSorting import java.io.*; import java.util.Scanner; public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program } else { String filePath = new File("").getAbsolutePath() + "/" + input; File file = new File(filePath); if (file.exists() && !file.isDirectory()) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { String st; StringBuilder formattedText = new StringBuilder(); while ((st = br.readLine()) != null) { // Split the input line into sentences using space as the delimiter String[] sentences = st.split(" "); for (int i = 0; i < sentences.length; i++) { StringBuilder sb = new StringBuilder(""); sb.append(Character.toUpperCase(sentences[i].charAt(0))); for (int j = 1; j < sentences[i].length(); j++) { char currentChar = sentences[i].charAt(j); if (currentChar == '!' || currentChar == '.') { sb.append(currentChar); if (j + 1 < sentences[i].length()) { sb.append(" "); sb.append(Character.toUpperCase(sentences[i].charAt(j + 1))); j++; } } else if (Character.isUpperCase(currentChar)) { sb.append(" "); sb.append(Character.toLowerCase(currentChar)); } else { sb.append(currentChar); } } formattedText.append(sb.toString() + ""); } formattedText.append("\n"); } System.out.print(formattedText); return; // Exit the program after printing } catch (IOException e) { e.printStackTrace(); } } else { System.out.println("File '" + input + "' is not found."); System.out.println("Please re-enter the file name or type QUIT to exit:"); } } } } } input2x2.csv -67,-11 -27,-70 input1.csv 10 input10x10.csv 56,-19,-21,-51,45,96,-46 -27,29,-58,85,8,71,34 50,51,40,50,100,-82,-87 -47,-24,-27,-32,-25,46,88 -47,95,-41,-75,85,-16,43 -78,0,94,-77,-69,78,-25 -80,-31,-95,82,-86,-32,-22 68,-52,-4,-68,10,-14,-89 26,33,-59,-51,-48,-34,-52 -47,-24,80,16,80,-66,-42 input0.csv Test Case 1 Please enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2x2.csvENTER -67,-11\n -70,-27\n Test Case 3 Please enter the file name or type QUIT to exit:\n input10x10.csvENTER -51,-46,-21,-19,45,56,96\n -58,-27,8,29,34,71,85\n -87,-82,40,50,50,51,100\n -47,-32,-27,-25,-24,46,88\n -75,-47,-41,-16,43,85,95\n -78,-77,-69,-25,0,78,94\n -95,-86,-80,-32,-31,-22,82\n -89,-68,-52,-14,-4,10,68\n -59,-52,-51,-48,-34,26,33\n -66,-47,-42,-24,16,80,80\n Test Case 4 Please enter the file name or type QUIT to exit:\n input0.csvENTER File input0.csv is empty.\n Test Case 5 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 6 Please enter the file name or type QUIT to exit:\n quitENTER Test Case 7 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n QUITENTER
Java Program ASAP
Modify this program so it passes the test cases in Hypergrade. Also change the public class to FileSorting
import java.io.*;
import java.util.Scanner;
public class ConvertText {
public static void main(String[] args) throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("Please enter the file name or type QUIT to exit:");
while (true) {
String input = sc.next();
if (input.equalsIgnoreCase("QUIT")) {
break; // Exit the program
} else {
String filePath = new File("").getAbsolutePath() + "/" + input;
File file = new File(filePath);
if (file.exists() && !file.isDirectory()) {
try (BufferedReader br = new BufferedReader(new FileReader(file))) {
String st;
StringBuilder formattedText = new StringBuilder();
while ((st = br.readLine()) != null) {
// Split the input line into sentences using space as the delimiter
String[] sentences = st.split(" ");
for (int i = 0; i < sentences.length; i++) {
StringBuilder sb = new StringBuilder("");
sb.append(Character.toUpperCase(sentences[i].charAt(0)));
for (int j = 1; j < sentences[i].length(); j++) {
char currentChar = sentences[i].charAt(j);
if (currentChar == '!' || currentChar == '.') {
sb.append(currentChar);
if (j + 1 < sentences[i].length()) {
sb.append(" ");
sb.append(Character.toUpperCase(sentences[i].charAt(j + 1)));
j++;
}
} else if (Character.isUpperCase(currentChar)) {
sb.append(" ");
sb.append(Character.toLowerCase(currentChar));
} else {
sb.append(currentChar);
}
}
formattedText.append(sb.toString() + "");
}
formattedText.append("\n");
}
System.out.print(formattedText);
return; // Exit the program after printing
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("File '" + input + "' is not found.");
System.out.println("Please re-enter the file name or type QUIT to exit:");
}
}
}
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER

Step by step
Solved in 4 steps with 3 images

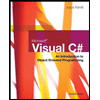
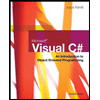