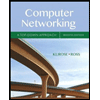
Implement Two Way Linked List
The MyLinkedList class is a one-way directional linked list that enables one-way traversal of the list. MyLinkedList.java is attached below. Modify the Node class to add the new field named previous to refer to the previous node in the list, as follows:
public class Node <E> { E element;
Node<E> next; Node<E> previous;
public Node(E e) { element = e;
} }
Implement a new class named MyTwoWayLinkedList that uses a double linked list to store elements. The MyLinkedList class in the text extends MyList. Define MyTwoWayLinkedList to extend the java.util.AbstractSequentialList class. You can find methods of AbstractSequentialList from this article: https://www.geeksforgeeks.org/abstractsequentiallist-in-java-with-examples/. Study MyLinkedList.java carefully to understand how it is structured, and where and what you need to modify/implement for MyTwoWayLinkedList.
Since MyTwoWayLinkedList extends java.util.AbstractSequentialList which implements Iterable<E>, it includes an iterator () method that returns an object of the iterator interface automatically. Read the article https://www.softwaretestinghelp.com/java/learn-to-use-java-iterator-with-examples/ for basics to use iterator. In order to iterate through MyTwoWayLinkedList, we need to implement the methods listIterator() and listIterator(int index). Both return an instance of java.util.ListIterator<E>. The former sets the cursor to head of the list and the latter to the element at the specific index.
public ListIterator<E> listIterator() { return new TwoWayLinkedListIterator();
}
public ListIterator<E> listIterator(int index) { return new TwoWayLinkedListIterator(index);
}
Here, TwoWayLinkedListIterator is a private class similar to LinkedListIterator in MyLinkedList.java. It defines a custom iterator for TwoWayLinkedList. Make sure to implement methods previous(), previousIndex(), and hasPrevious() similar to next(), nextIndex(), and hasNext() methods in MyLinkedList class.
All the methods dealing with add/remove may need to be modified to handle both next and previous fields.
Implement a new class named TestMyTwoWayLinkedList to test your two-way linked list with Double as the concert type for the elements in the linked list.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- A business that sells dog food keeps information about its dog food products in a linked list. The list is named dogFoodList. (This means dogFoodList points to the first node in the list.) A node in the list contains the name of the dog food (a String), a dog food ID (also a String) and the price (a double.) a.) Create a class for a node in the list. b.) Use this class to write pseudocode or Java for a public method that prints the name of all dog foods in the list where the price is more than $20.00.arrow_forwardpackage hw5; public class LinkedIntSet {private static class Node {private int data;private Node next; public Node(int data, Node next) {this.data = data;this.next = next;}} private Node first; // Always points to the first node of the list.// THE LIST IS ALWAYS IN SORTED ORDER!private int size; // Always equal to the number of elements in the set. /*** Construts an empty set.*/public LinkedIntSet() {throw new RuntimeException("Not implemented");} /*** Returns the number of elements in the set.* * @return the number of elements in the set.*/public int size() {throw new RuntimeException("Not implemented");} /*** Tests if the set contains a number* * @param i the number to check* @return <code>true</code> if the number is in the set and <code>false</code>* otherwise.*/public boolean contains(int i) {throw new RuntimeException("Not implemented");} /*** Adds <code>element</code> to this set if it is not already present and* returns…arrow_forwardStart this lab with the code listed below. The LinkedList class defines the rudiments of the code needed to build a linked list of Node objects. You will first complete the code for its addFirst method. This method is passed an object that is to be added to the beginning of the list. Write code that links the passed object to the list by completing the following tasks in order:1. Create a new Node object.2. Make the data variable in the new Node object reference the object that was passed to addFirst.3. Make the next variable in the new Node object reference the object that is currently referenced in variable first.4. Make variable first reference the new Node.Test your code by running the main method in the LinkedListRunner class below. Explain, step by step, why each of the above operations is necessary. Why are the string objects in the reverse order from the way they were added? public class LinkedList{ private Node first; public LinkedList() { first = null; } public Object…arrow_forward
- Could you help me the following question? The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list. For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This…arrow_forwardLab 19 Building a linked list Start this lab with the code listed below. The LinkedList class defines the rudiments of the code needed to build a linked list of Node objects. You will first complete the code for its addFirst method. This method is passed an object that is to be added to the beginning of the list. Write code that links the passed object to the list by completing the following tasks in order: 1. Create a new Node object. 2. Make the data variable in the new Node object reference the object that was passed to addFirst. 3. Make the next variable in the new Node object reference the object that is currently referenced in variable first. 4. Make variable first reference the new Node. Test your code by running the main method in the LinkedListRunner class below. Explain, step by step, why each of the above operations is necessary. Why are the string objects in the reverse order from the way they were added? public class LinkedList { private Node first; public LinkedList () {…arrow_forwardLinked List, create your own code. (Do not use the build in function or classes of Java or from the textbook). Create a LinkedList class: Call the class MyLinkedList, (hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should haveo Variables you may need for a Node,o (optional) Constructor Your linked list is of an int type. (you may do it as General type as <E>) For this Linked List you need to have the following methods: add, addAfter, remove, size, contain, toString, compare, addInOrder. This is just a suggestion, if you use Generic type, you must modify this Write a main function or Main class to test all the methods,o Create a 2 linked list and test all your methods. (Including the compare)arrow_forward
- Tree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forward/** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forwardGiven main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.arrow_forward
- Implement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forwardAssume you have a class SLNode representing a node in a singly-linked list and a variable called list referencing the first element on a list of integers, as shown below: public class SLNode { private E data; private SLNode next; public SLNode( E e){ data = e; next = null; } public SLNode getNext() { return next; } public void setNext( SLNoden){ next = n; } } SLNode list; Write a fragment of Java code that would append a new node with data value 21 at the end of the list. Assume that you don't know if the list has any elements in it or not (i.e., it may be empty). Do not write a complete method, but just show a necessary fragment of code.arrow_forwardJava Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Second image is ItemNodearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
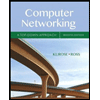
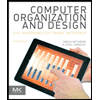
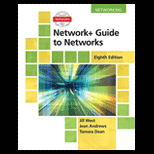
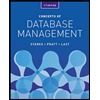
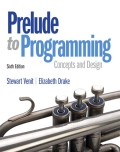
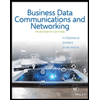