Modify the unsortedArrayAccess class by adding a method that find the smallest element and average in the array. import java.util.*; public class unsortedArrayAccess { public static double[] arr; public int arraySize; public unsortedArrayAccess(int scale) { arr = new double[scale]; arraySize = 0; } public int search(double Key) { int i = 0; while ((i < arraySize) && (arr[i] != Key) ) { i = i + 1; } if (i < arraySize) { return i; } else { System.out.println("There is no such item!"); return -1; } } public void append(double Item) { arr[arraySize] = Item; arraySize = arraySize + 1; } public double remove() { if (arraySize == 0) { System.out.println("There is no item in the array!"); return -1; } double x = arr[arraySize - 1]; arraySize = arraySize - 1; return x; } public void deletion(double Key) { int k = search(Key); if (k != -1) { for (int i = k; i < arraySize; i++) { arr[i] = arr[i + 1]; } arraySize = arraySize - 1; }; } public void display() { if (arraySize == 0) { System.out.println("Array is empty!"); } else { for (int i = 0; i < arraySize; i++) { System.out.println(arr[i]); } }; System.out.println("array size is " + arraySize); } public double smallest() { double small = arr[0]; for(int i =1; i
Modify the unsortedArrayAccess class by adding a method that find the smallest element and average in the array.
import java.util.*;
public class unsortedArrayAccess
{
public static double[] arr;
public int arraySize;
public unsortedArrayAccess(int scale)
{
arr = new double[scale];
arraySize = 0;
}
public int search(double Key)
{
int i = 0;
while ((i < arraySize) && (arr[i] != Key) )
{
i = i + 1;
}
if (i < arraySize)
{
return i;
}
else
{
System.out.println("There is no such item!");
return -1;
}
}
public void append(double Item)
{
arr[arraySize] = Item;
arraySize = arraySize + 1;
}
public double remove()
{
if (arraySize == 0)
{
System.out.println("There is no item in the array!");
return -1;
}
double x = arr[arraySize - 1];
arraySize = arraySize - 1;
return x;
}
public void deletion(double Key)
{
int k = search(Key);
if (k != -1)
{
for (int i = k; i < arraySize; i++)
{
arr[i] = arr[i + 1];
}
arraySize = arraySize - 1;
};
}
public void display()
{
if (arraySize == 0)
{
System.out.println("Array is empty!");
}
else
{
for (int i = 0; i < arraySize; i++)
{
System.out.println(arr[i]);
}
};
System.out.println("array size is " + arraySize);
}
public double smallest()
{
double small = arr[0];
for(int i =1; i<arraySize; i++)
{
if(arr[i]<small)
small=arr[i];
}
return small;
}
public double average()
{
double sum=0.0;
for(int i=0; i<arraySize; i++)
sum = sum +arr[i];
if( arraySize>0)
return sum/arraySize;
else
return -999999.0;
}
public static void main (String[] args) {
Main a=new Main(50);
a.append(150);
a.display();
}
}

Program modification:
- Define the smallest() function that is called by the called by class object
- Initialize a variable small that holds the first element of the array
- Iterate over the array to compare the array value with the variable small and swap the current element if it is smaller than the variable small
- Return the value of the variable small
- Define the average() function that is called by the called by class object
- Initialize a variable sum that holds the sum of array elements
- Iterate over the array and add the elements to variable sum
- Evaluate the average using the below-mentioned expression
- print the average of the array elements
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

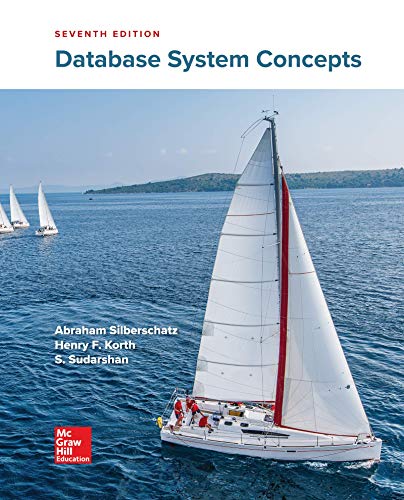
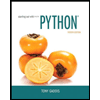
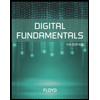
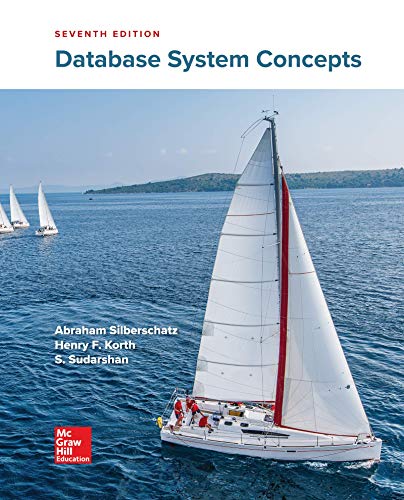
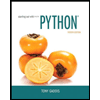
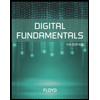
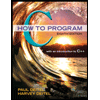
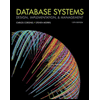
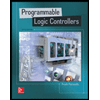