modify Exercise 6.57 in a way that every time the user give the right answer , the response varies within the following : Possible responses to a correct answer: Very good! Excellent! Nice work! Keep up the good work! while if the user give the incorrect answer the response varies within the following : Possible responses to an incorrect answer: No. Please try again. Wrong. Try once more. Don't give up! No. Keep trying. Use random-number generation to choose a number from 1 to 4 that will be used to select one of the four appropriate responses to each correct or incorrect answer. Use a switch statement to issue the responses. here is the code that need to be modified : and make sure you add line comments for each new line of code EXERCISE 6.57 CODE: ----------------------------- //Name: IhabAtouf //Date:02/23/2023 // exercise 6.57 on page 281 //program description: create computer-assisted instruction (CAI) program that help students master thier math skills in multiplications, // multiplication involving two numbers each is a single digit positive, with encouraging message for succedding and another for failing to answer // using random fucntion (rand) to generate a random number every time #include// to be able to use input output stream #include//h is a header file in the standard library of the C programming language designed for basic mathematical operations #include//Converts a string into a long integer, also carry the library for definition of memory allocation and random processe #include//converts the given time since epoch to a calendar local time and then to a character representation. using namespace std;//using the standard library int answer;//global varibale that can be used by any function in this code void questionGenerator() {//the function that generates the multiplication question srand(time(NULL));// using the random function that changes every time per second //here is the initial variable as zero by default int initial = 0; // here is two vraibales number1 and number2 that are required to multiply two numbers int number1; int number2; number1 = (initial + (rand()) % 10);//the value of number1 using the follwing math : zero + any random number that has remainder of 10 using the remainder modulus number2 = (initial + (rand()) % 10);//the value of number2 using the follwing math : zero + any random number that has remainder of 10 using the remainder modulus answer = number1 * number2;// the asnwer variable that equals the multiplication of those two numbers (number1 and 2) cout << "How much is " << number1 << " times " << number2 << " ?" << endl;//print how much is number 1 multiplied by number 2 ? } int main() {// the main function int studentResponse = 1;// declaring local variable called student response questionGenerator();// calling the question generator function while (studentResponse != -1) {// as long as the student response not equal to -1 then: cout << "Enter the correct answer" << endl;// print enter the correct answer statement cin >> studentResponse;// allow user to input the student response variables value if (answer == studentResponse) {//if the studenrt response entered by user equal the answer variable of multiplying those two numbers1 and number2 variables cout << "Very Good!" << endl;// then print very good statement questionGenerator();// then recall the function again for the net set of random numebrs multiplication continue;// continue till user enter incorrect answer } else {// otherwise when answer not correct then print please try again statement cout << "Please try again!" << endl; while (studentResponse != answer) {// while loop if student response not equal to the correct answer then: cout << "Enter the correct answer" << endl;//print message enter correct answer cin >> studentResponse;//allow user to reneter hoping to get the correct answer, this will keep repeats as long as the student answer and the corredct answer doesnt match if (answer == studentResponse) {// if the answer match with student answer then: cout << "Very Good!" << endl;// then print very good statement questionGenerator();// then recall the function again for the net set of random numebrs multiplication question continue;// continue till user input incorrect answer } } } } return 0; } ------------------------------ END OF CODE
modify Exercise 6.57 in a way that every time the user give the right answer , the response varies within the following :
Possible responses to a correct answer:
Very good!
Excellent!
Nice work!
Keep up the good work!
while if the user give the incorrect answer the response varies within the following :
Possible responses to an incorrect answer:
No. Please try again.
Wrong. Try once more.
Don't give up!
No. Keep trying.
Use random-number generation to choose a number from 1 to 4 that will be used to select one of the four appropriate responses to each correct or incorrect answer. Use a switch statement to issue the responses.
here is the code that need to be modified :
and make sure you add line comments for each new line of code
EXERCISE 6.57 CODE:
-----------------------------
//Name: IhabAtouf
//Date:02/23/2023
// exercise 6.57 on page 281
//program description: create computer-assisted instruction (CAI) program that help students master thier math skills in multiplications,
// multiplication involving two numbers each is a single digit positive, with encouraging message for succedding and another for failing to answer
// using random fucntion (rand) to generate a random number every time
#include<iostream>// to be able to use input output stream
#include<math.h>//h is a header file in the standard library of the C
#include<cstdlib>//Converts a string into a long integer, also carry the library for definition of memory allocation and random processe
#include<ctime>//converts the given time since epoch to a calendar local time and then to a character representation.
using namespace std;//using the standard library
int answer;//global varibale that can be used by any function in this code
void questionGenerator() {//the function that generates the multiplication question
srand(time(NULL));// using the random function that changes every time per second
//here is the initial variable as zero by default
int initial = 0;
// here is two vraibales number1 and number2 that are required to multiply two numbers
int number1;
int number2;
number1 = (initial + (rand()) % 10);//the value of number1 using the follwing math : zero + any random number that has remainder of 10 using the remainder modulus
number2 = (initial + (rand()) % 10);//the value of number2 using the follwing math : zero + any random number that has remainder of 10 using the remainder modulus
answer = number1 * number2;// the asnwer variable that equals the multiplication of those two numbers (number1 and 2)
cout << "How much is " << number1 << " times " << number2 << " ?" << endl;//print how much is number 1 multiplied by number 2 ?
}
int main() {// the main function
int studentResponse = 1;// declaring local variable called student response
questionGenerator();// calling the question generator function
while (studentResponse != -1) {// as long as the student response not equal to -1 then:
cout << "Enter the correct answer" << endl;// print enter the correct answer statement
cin >> studentResponse;// allow user to input the student response variables value
if (answer == studentResponse) {//if the studenrt response entered by user equal the answer variable of multiplying those two numbers1 and number2 variables
cout << "Very Good!" << endl;// then print very good statement
questionGenerator();// then recall the function again for the net set of random numebrs multiplication
continue;// continue till user enter incorrect answer
}
else {// otherwise when answer not correct then print please try again statement
cout << "Please try again!" << endl;
while (studentResponse != answer) {// while loop if student response not equal to the correct answer then:
cout << "Enter the correct answer" << endl;//print message enter correct answer
cin >> studentResponse;//allow user to reneter hoping to get the correct answer, this will keep repeats as long as the student answer and the corredct answer doesnt match
if (answer == studentResponse) {// if the answer match with student answer then:
cout << "Very Good!" << endl;// then print very good statement
questionGenerator();// then recall the function again for the net set of random numebrs multiplication question
continue;// continue till user input incorrect answer
}
}
}
}
return 0;
}
------------------------------
END OF CODE

Here is the approach :
- To generate the random number in c++ is very easy task .
- We have to use the random generator . Hence get the random generator .
- We want to generate the random number within the range of 1 to 4 because we have total 4 messages in both the cases success and failure .
- Hence to generate the random number within the range of 1 to 4 ---> 1+ (rand() % 4)
- Here we are using the modulo operator to generate the random number in the range .
- Next we need the switch case .
- In the switch case switch the randomly generated number .
- In the code store the randomly generated message in some string variable .
- Check the code and output in the second step . Everything is mentioned in the comments .
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

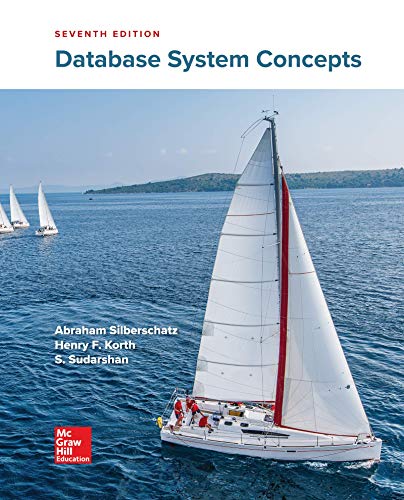
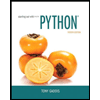
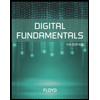
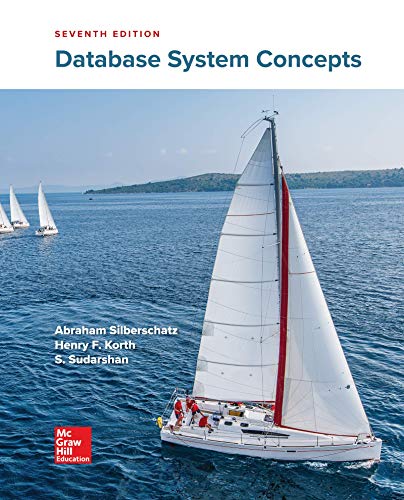
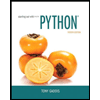
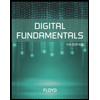
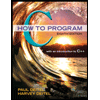
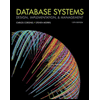
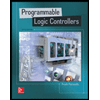