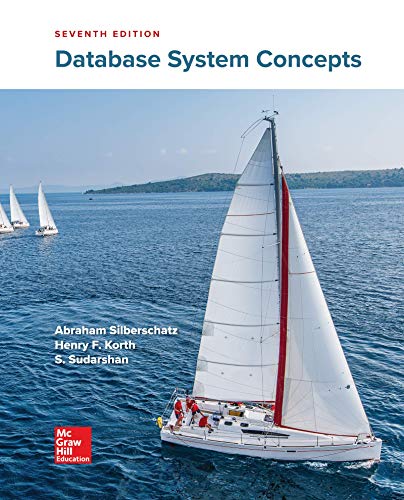
[Python Language]
Using loops of any kind, lists, or Sets is not allowed.
Sloan’s Book Collection
Sloan loves reading books. She recently started reading an
Sloan is collecting books from the series at her nearest bookstore. Since the series is AI generated, the publishers have produced an infinite collection of the books where each book is identified by a unique integer. The bookstore has exactly one copy of each book.
Sloan wants to buy the books in the range [l,r], where l ≤ r. As an example, the range [−3,3] means that Sloan wants to buy the books − 3, − 2, − 1, 0, 1, 2, and 3.
Pam also loves the series (or maybe annoying Sloan– who knows, really), and he manages to sneak into the bookstore very early to buy all of the books in the range [d,u], where d ≤ u. When Sloan later visits, sadly she will not find those books there anymore.
For example, if Sloan tries to buy books [−2,3] and Pam has bought books [0,2], Sloan would only receive books − 2, − 1, and 3.
The reading recommendation score of the books is the product of the book numbers received. So if Sloan gets books − 2, − 1, and 3, for instance, the reading recommendation score will be − 2 × − 1 × 3 = 6.
Your task is to write a
Filename
Your filename for this question must be q2.py.
Input
There are four lines of input.
- The first line of input will contain the integer l, the lower bound of the collection of books that Sloan would like to buy.
- The second line of input will contain the integer r, the upper bound of the collection of books that Sloan would like to buy.
- The third line of input will contain the integer d, the lower bound of the collection of books that Pam buys.
- The fourth line of input will contain the integer u, the upper bound of the collection of books that Pam buys.
Output
Let s be the reading recommendation score of the book collection Sloan receives.
- If s is less than 0, print NEGATIVE.
- If s is exactly 0, print ZERO.
- If s is greater than 0, print POSITIVE.
- If s does not exist, print EMPTY.
Sample Input 1
1 10 3 7
Sample Output 1
POSITIVE
Sample Input 2
1 3 1 3
Sample Output 2
EMPTY
Sample Input 3
-5 5 -2 3
Sample Output 3
NEGATIVE
Sample Input 4
-1 3 -2 -1
Sample Output 4
ZERO

Step by stepSolved in 3 steps with 1 images

- Virus DNA files As a future doctor, Jojo works in a laboratory that analyzes viral DNA. Due to the pandemic During the virus outbreak, Jojo received many requests to analyze whether there was any viral DNA in the patient. This number of requests made Jojo's job even more difficult. Therefore, Jojo ask Lili who is a programmer for help to make a program that can read the file containing the patient's DNA data and viral DNA and then match them. If on the patient's DNA found the exact same string pattern, then write to the index screen the found DNA. Data contained in the file testdata.in Input Format The first line of input is the number of test cases TThe second row and so on as many as T rows are the S1 string of patient DNA and the S2 string of viral DNA separated by spaces Output Format The array index found the same string pattern. Constraints 1 ≤ T ≤ 1003 ≤ |S2| ≤ |S1| ≤ 100|S| is the length of the string.S will only consist of lowercase letters [a-z] Sample Input (testdata.in)…arrow_forwardUse Java programming language Write a program that asks the user to enter 5 test grades (use an array to store them). Output the grades entered, the lowest and highest grade, the average grade, how many grades are above the average and how many are below and the letter grade for the average grade. Create a method that returns the lowest grade. Create a method that returns the highest grade. Create a method that returns the average grade. Create a method that returns how many grades were above the average. Create a method that returns how many grades were below the average. Create a method that returns the letter grade of the average (90-100 – A, 80-89 – B, 70-79 – C, < 70 – F)arrow_forwardJava Programming: Write a command line game that plays a simple version of blackjack. The program should generate random numbers between 1 and 10 each time the player gets a card. It should keep a running total of the players cards, and ask the player whether or not it should deal another card. Sample output for the game is written below. Your program should produce the same output. The players get two cards to start with. Then they are asked if they want more cards. Players can continue to take as many cards as they like. The goal is to get close to 21 without going over. If the total is greater than 21 we say the player "busted".arrow_forward
- cerat javaFX 9-Rock, Paper, Scissors GameCreate a JavaFX application that lets the user play the game of rock, paper, scissors againstthe computer. The program should work as follows.1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, thenthe computer has chosen paper. If the number is 3, then the computer has chosenscissors. (Do not display the computer’s choice yet.)2. The user selects his or her choice of rock, paper, or scissors by clicking a Button. Animage of the user’s choice should be displayed in an ImageView component. (Youwill find rock, paper, and scissors image files in the book’s Student Sample Files.)3. An image of the computer’s choice is displayed.4. A winner is selected according to the following rules:l If one player chooses rock and the other player chooses scissors, then rock wins.(Rock smashes scissors.)l If one player chooses scissors and the other player…arrow_forwardJava Programming: Write a program that reads five integer values from the user, then analyzes them as if they were a hand of cards. When your program runs it might look like this (user input is in orange for clarity): Enter five numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 9 Pair! (This is a pair, since there are two eights). You are only required to find the hands Pair and Straight.arrow_forwardBlue-Eyed Island: A group of individuals live on an island until a visitor arrives with an unusual order: all blue-eyed people must leave the island as quickly as possible. Every evening at 8:00 p.m., a flight will depart. Everyone can see everyone else's eye colour, but no one knows their own (nor is anyone allowed to tell them). Furthermore, they have no idea how many people have blue eyes, but they do know that at least one person does. How long will it take for the blue-eyed individuals to leave?arrow_forward
- Please written by computer source Please solve with C - NIM Gamearrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a number and recursively determine how many of its digits are even. Return the count of the even digits in each number. % might prove useful to take the number apart digit by digit Sample Data : 453211145322224532714246813579 Sample Output : 23540arrow_forwardRandom Numbers: Most computer programs do the same thing every time they run; programs like that are called deterministic. Usually, determinism is a good thing, since we expect the same calculation to yield the same result. But for some applications, we want the computer to be unpredictable. Games are an obvious example, but there are many others, like scientific simulations. We have already seen java.util.Random, which generates pseudorandom numbers. The method nextInt takes an integer argument, n, and returns a random integer between 0 and n - 1. Assignment: For this assignment you will write a program in java to simulate a guessing game. Your program will generate a random number in a certain range (let the user define the range) and ask the user to guess what number it is. Give the user a certain number of tries while you narrow down the possibilities. For example, let's say the number generated is 67 and the user has 5 tries, this could be your program's output: This is a guessing…arrow_forward
- Random Numbers: Most computer programs do the same thing every time they run; programs like that are called deterministic. Usually, determinism is a good thing, since we expect the same calculation to yield the same result. But for some applications, we want the computer to be unpredictable. Games are an obvious example, but there are many others, like scientific simulations. We have already seen java.util.Random, which generates pseudorandom numbers. The method nextint takes an integer argument, n, and returns a random integer between 0 and n - 1. Assignment For this assignment you will write a program in java to simulate a guessing game. Your program will generate a random number in a certain range (let the user define the range) and ask the user to guess what number it is. Give the user a certain number of tries while you narrow down the possibilities. For example, let's say the number generated is 67 and the user has 5 tries, this could be your program's output: This is a guessing…arrow_forwardShowCurrentTime.java an example program in Chapter, gives a program that displays the current time in GMT. Common old news shows would show clocks across many time zones, write a program that modifies ShowCurrent Time and will display the time in prominent business centers. London, Paris, New York was common. Write a program that displays the time in: Berlin, London, New York, Chicago, San Francisco, Tokyo and Hong Kong. ↓ HONG KONG ↓ LONDON L PARIS TOKYO MOSCOW 101 BERLIN DUBAI iStock Credit: Tetlana Muslyaka NEW YORKarrow_forwardPlease solve this java problem and including the GUI to run it.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
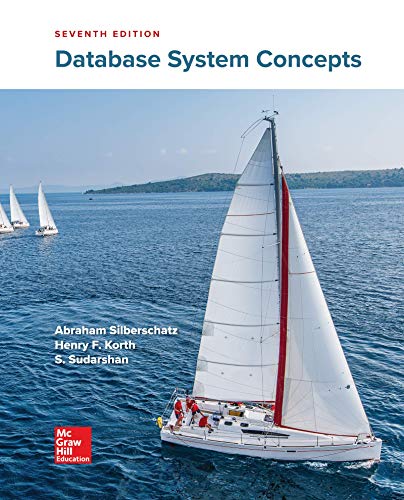
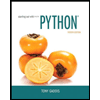
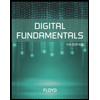
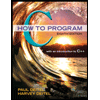
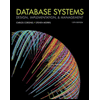
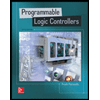