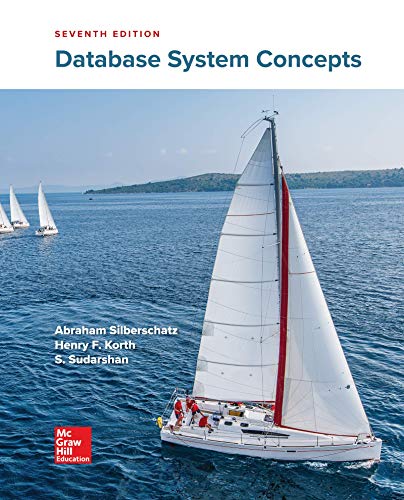
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
May I please have your assistance with this lab:
2.15 LAB: Artwork label ( modules)
Part of the code is correct. I am only needing the code to be correct for the following errors:
This is the current code & errors at bottom of code:
# Artist.py
class Artist:
# below is the comstructor to initializez artist infomration
# constructor initialize the artist's name to "None" and the years of birth and death to 0
def __init__(self, name='None', birth_year=0, death_year=0):
self.name = name
self.birth_year = birth_year
self.death_year = death_year
def print_info(self):
if self.birth_year >= 0 and self.death_year >= 0:
print(f'Artist: {self.name} ({self.birth_year} to {self.death_year})')
elif self.birth_year >= 0:
print(f'Artist: {self.name} ({self.birth_year} - present)')
else:
print(f'Artist: {self.name} (unkown)')
# Artwork.py
class Artwork:
def __init__(self, title= "None", year_created=0, artist=Artist()):
self.title = title
self.year_created = year_created
self.artist = artist
def print_info(self):
self.artist.print_info()
print(f'Title: {self.title}, {self.year_created}')
# Main.py
if __name__ == "__main__":
user_artist_name = input()
user_birth_year = int(input())
user_death_year = int(input())
user_title = input()
user_year_created = int(input())
user_artist = Artist(user_artist_name, user_birth_year, user_death_year)
new_artwork = Artwork(user_title, user_year_created, user_artist)
new_artwork.print_info()
Current Errors:
4:Unit testkeyboard_arrow_up
0 / 2
Tests Artist constructor with default param values
AttributeError: 'Artist' object has no attribute 'name'
5:Unit testkeyboard_arrow_up
0 / 2
Tests that Artist('Pablo Picasso', 1881, 1973) correctly initializes artist
TypeError: Artist() takes no arguments
6:Unit testkeyboard_arrow_up
0 / 2
Tests that Artist('Brice Marden', 1938, -1) correctly initializes artist
TypeError: Artist() takes no arguments
7:Unit testkeyboard_arrow_up
0 / 1
Tests Artwork constructor with default param values
AttributeError: 'Artwork' object has no attribute 'title'
Please see attached lab and error messages.
Thank you.
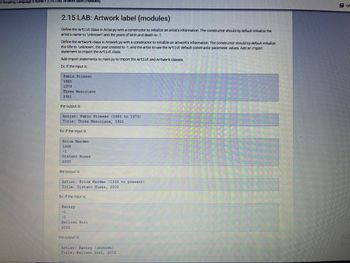
Transcribed Image Text:2.15 LAB: Artwork label (modules)
Define the Artist class in Artist.py with a constructor to initialize an artist's information. The constructor should by default initialize the artist's name to "unknown" and the years of birth and death to -1.
Define the Artwork class in Artwork.py with a constructor to initialize an artwork's information. The constructor should by default initialize the title to "unknown", the year created to -1, and the artist to use the Artist class's default constructor parameter values. Add an import statement to import the Artist class.
Add import statements to main.py to import the Artist and Artwork classes.
Ex: If the input is:
```
Pablo Picasso
1881
1973
Three Musicians
1921
```
the output is:
```
Artist: Pablo Picasso (1881 to 1973)
Title: Three Musicians, 1921
```
Ex: If the input is:
```
Brice Marden
1938
-1
Distant Muses
2000
```
the output is:
```
Artist: Brice Marden (1938 to present)
Title: Distant Muses, 2000
```
Ex: If the input is:
```
Banksy
-1
-1
Balloon Girl
2002
```
the output is:
```
Artist: Banksy (unknown)
Title: Balloon Girl, 2002
```
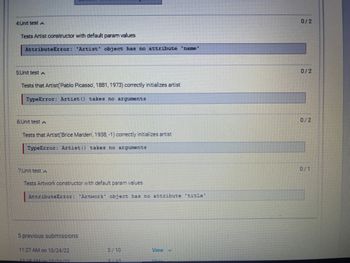
Transcribed Image Text:The image shows the results of a series of unit tests related to the construction of `Artist` and `Artwork` objects.
### Detailed Explanation:
1. **4th Unit Test (0/2):**
- **Test Description:** Tests the `Artist` constructor with default parameter values.
- **Error Message:** `AttributeError: 'Artist' object has no attribute 'name'`
- **Explanation:** This error indicates that there is an attempt to access an attribute `name` that does not exist in the `Artist` class when using default parameters.
2. **5th Unit Test (0/2):**
- **Test Description:** Tests that `Artist(Pablo Picasso, 1881, 1973)` correctly initializes the artist.
- **Error Message:** `TypeError: Artist() takes no arguments`
- **Explanation:** The error suggests that the `Artist` class constructor is not designed to accept arguments, but arguments were provided during initialization.
3. **6th Unit Test (0/2):**
- **Test Description:** Tests that `Artist(Brice Marden, 1938, -1)` correctly initializes the artist.
- **Error Message:** `TypeError: Artist() takes no arguments`
- **Explanation:** Similar to the 5th test, the constructor is not accepting arguments as expected, leading to a `TypeError`.
4. **7th Unit Test (0/1):**
- **Test Description:** Tests the `Artwork` constructor with default parameter values.
- **Error Message:** `AttributeError: 'Artwork' object has no attribute 'title'`
- **Explanation:** This error points out that the `Artwork` class is missing a `title` attribute when default parameters are used.
### Additional Information:
- Below the unit tests, there is a section labeled "5 previous submissions" with two time stamps: 11:27 AM on 10/24/22 and 11:18 AM on 10/24/22. The submissions scored 3/10 and 0/10 respectively, with an option to view more information.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- // Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forwardDesign a class named Pet, which should have the following fields: • name. The name field holds the name of a pet. • animal. The animal field holds the type of animal that a pet is. Example values are “Dog”, “Cat”, and “Bird”. • age. The age field holds the pet’s age. The Pet class should also have the following methods: • setName. The setName method stores a value in the name field. • setAnimal. The setAnimal method stores a value in the animal field. • setAge. The setAge method stores a value in the age field. • getName. The getName method returns the value of the name field. • getAnimal. The getAnimal method returns the value of the animal field. • getAge. The getAge method returns the value of the age field. Write the Java code for the Pet class and demonstrate it.arrow_forward
- The xxx_Student class:– Name - the name consists of the First and Last name separated by a space.– Student Id – a whole number automatically assigned in the student class– Student id numbers start at 100. The numbers are assigned using a static variable in the Student class• Include all instance variables• Getters and setters for instance variables• A static variable used to assign the student id starting at 100• A toString method which returns a String containing the student name and id in the format below:Student: John Jones ID: 101 The xxx_Course classA Course has the following information (modify your Course class):– A name– An Array of Students which contains an entry for each Student enrolled in the course (allow for up to 10 students)– An integer variable which indicates the number of students currently enrolled in the course. Write the constructor below which does the following:Course (String name)Sets courseName to nameCreates the students array of size 10Sets number of…arrow_forwardPet ClassDesign a class named Pet, which should have the following fields: name: The name field holds the name of a pet. type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird". age: The age field holds the pet’s age. The Pet class should also have the following methods: setName: The setName method stores a value in the name setType: The setType method stores a value in the type setAge: The setAge method stores a value in the age getName: The getName method returns the value of the name getType: The getType method returns the value of the type getAge: The getAge method returns the value of the age Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. The output Should be…arrow_forward#pyhton programing topic: Introduction to Method and Designing class Method overloading & Constructor overloading ------------------ please find the attached imagearrow_forward
- INVENTORY CLASS You need to create an Inventory class containing the private data fields, as well as the methods for the Inventory class (object). Be sure your Inventory class defines the private data fields, at least one constructor, accessor and mutator methods, method overloading (to handle the data coming into the Inventory class as either a String and/or int/float), as well as all of the methods (methods to calculate) to manipulate the Inventory class (object). The data fields in the Inventory class include the inventory product number (int), inventory product description (String), inventory product price (float), inventory quantity on hand (int), inventory quantity on order (int), and inventory quantity sold (int). The Inventory class (java file) should also contain all of the static data fields, as well as static methods to handle the logic in counting all of the objects processed (counter), as well as totals (accumulators) for the total product inventory and the total product…arrow_forwardclass Book: book_belongs_to = 'Schulich School of Engineering' def _init_(self, pages = 0, title = 'Unknown', author = 'Unknown', isbn = self.pages = pages self.title = title e): self.author = author self.isbn = isbn book1 = Book(255, 'Black Beauty', 'Anna Sewell', 9780001840423) book2 = Book(208, 'The Chrysalids', 'John Wyndham', 9780140013085) Book.book_belongs_to = 'Emily Marasco' book3 = Book()arrow_forwardIN C++ Lab #6: Shapes Create a class named Point. private attributes x and y of integer type. Create a class named Shape. private attributes: Point points[6] int howManyPoints; Create a Main Menu: Add a Triangle shape Add a Rectangle shape Add a Pentagon shape Add a Hexagon shape Exit All class functions should be well defined in the scope of this lab. Use operator overloading for the array in Shape class. Once you ask the points of any shape it will display in the terminal the points added.arrow_forward
- 6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forwardDice Rolling Class In this problem, you will need to create a program that simulates rolling dice. To start this project, you will first need to define the properties and behaviors of a single die that can be reused multiple times in your future code. This will be done by creating a Dice class. Create a Dice class that contains the following members: Two private integer variables to store the minimum and maximum roll possible. Two constructors that initialize the data members that store the min/max possible values of rolls. a constructor with default min/max values. a constructor that takes 2 input arguments corresponding to the min and max roll values Create a roll() function that returns a random number that is uniformly distributed between the minimum and maximum possible roll values. Create a small test program that asks the user to give a minValue and maxValue for a die, construct a single object of the Dice class with the constructor that initializes the min and max…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
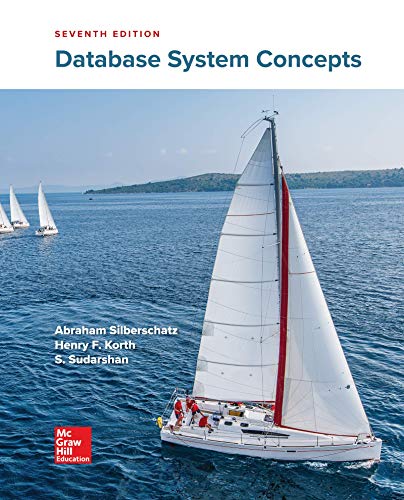
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
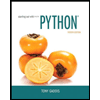
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
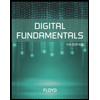
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
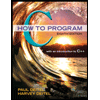
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
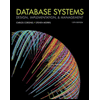
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
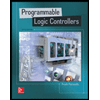
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education