I need a step by step explanation on how to create this project. Project: Flight Time Problem Description: Design two classes: Flight and Itinerary. The Flight class stores the information about a flight with the following members: A data field named flightNo of the String type with getter function. A data field named departureTime of the GregorianCalendar type with getter and setter functions. A data field named arrivalTime of the GregorianCalendar type with getter and setter functions. A constructor that creates a Flight with the specified number, departureTime, and arrivalTime. A function named getFlightTime() that returns the flight time in minutes. The Itinerary class stores the information about the itinerary with the following members: A data field named flights of the List type. The list contains the flights for the itinerary in increasing order of departureTime. A constructor that creates an Itinerary with the specified fights. A function named getTotalTime() that returns the total travel time in minutes from the departure time and the first flight to the arrival time of the last flight in the itinerary. Implement these two classes and use the following program to test these classes. int main() { vector flights; flights.push_back(Flight("US230", Time(2014, 4, 5, 5, 5, 0), Time(2014, 4, 5, 6, 15, 0))); flights.push_back(Flight("US235", Time(2014, 4, 5, 6, 55, 0), Time(2014, 4, 5, 7, 45, 0))); flights.push_back(Flight("US237", Time(2014, 4, 5, 9, 35, 0), Time(2014, 4, 5, 12, 55, 0))); Itinerary itinerary(flights); cout << itinerary.getTotalTravelTime() << endl; cout << itinerary.getTotalFlightTime() << endl; return 0; } Analysis: (Describe the problem, including input and output in your own words.) Design: (Describe the major steps for solving the problem.) Coding: Using Visual Studio, create the following program. Include the following in the .cpp file: Using comments at the top of the file, your first and last name, date, and purpose of the program Meaningful comments to explain the code throughout the program. Place your initials in front of all variable names (ex. BWflightNo) Place your initials in front of all function names (ex. BWgetTotalTraveltime()) Submit the FirstNameLastNameAnagrams.cpp (BridgetWillisFlightTimes.cpp) file to the drop box Testing: (Describe how you test this program) Submit the following items: Submit the Word file Submit the .cpp file
I need a step by step explanation on how to create this project.
Project: Flight Time
Problem Description:
Design two classes: Flight and Itinerary. The Flight class stores the information about a flight with the following members:
- A data field named flightNo of the String type with getter function.
- A data field named departureTime of the GregorianCalendar type with getter and setter functions.
- A data field named arrivalTime of the GregorianCalendar type with getter and setter functions.
- A constructor that creates a Flight with the specified number, departureTime, and arrivalTime.
- A function named getFlightTime() that returns the flight time in minutes.
The Itinerary class stores the information about the itinerary with the following members:
- A data field named flights of the List<Flight> type. The list contains the flights for the itinerary in increasing order of departureTime.
- A constructor that creates an Itinerary with the specified fights.
- A function named getTotalTime() that returns the total travel time in minutes from the departure time and the first flight to the arrival time of the last flight in the itinerary.
Implement these two classes and use the following
int main()
{
vector<Flight> flights;
flights.push_back(Flight("US230",
Time(2014, 4, 5, 5, 5, 0),
Time(2014, 4, 5, 6, 15, 0)));
flights.push_back(Flight("US235",
Time(2014, 4, 5, 6, 55, 0),
Time(2014, 4, 5, 7, 45, 0)));
flights.push_back(Flight("US237",
Time(2014, 4, 5, 9, 35, 0),
Time(2014, 4, 5, 12, 55, 0)));
Itinerary itinerary(flights);
cout << itinerary.getTotalTravelTime() << endl;
cout << itinerary.getTotalFlightTime() << endl;
return 0;
}
Analysis:
(Describe the problem, including input and output in your own words.)
Design:
(Describe the major steps for solving the problem.)
Coding: Using Visual Studio, create the following program. Include the following in the .cpp file:
- Using comments at the top of the file, your first and last name, date, and purpose of the program
- Meaningful comments to explain the code throughout the program.
- Place your initials in front of all variable names (ex. BWflightNo)
- Place your initials in front of all function names (ex. BWgetTotalTraveltime())
- Submit the FirstNameLastNameAnagrams.cpp (BridgetWillisFlightTimes.cpp) file to the drop box
Testing: (Describe how you test this program)
Submit the following items:
- Submit the Word file
- Submit the .cpp file

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

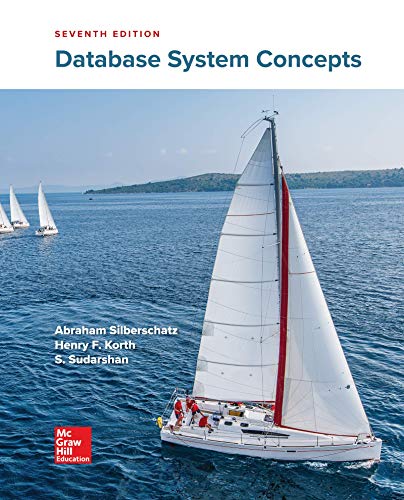
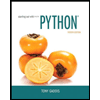
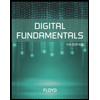
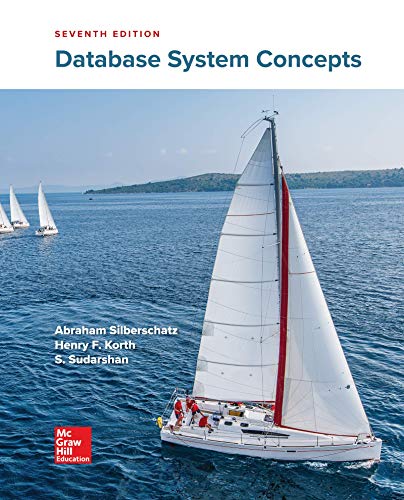
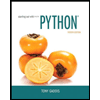
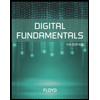
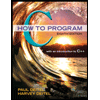
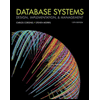
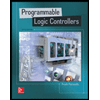