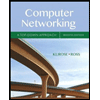
make structure chart for this code plz
#include <stdio.h>
#include <term.h>
char box[10]={'0','1','2','3','4','5','6','7','8','9'};
void create_board();
void make_board(int, char);
int check_win();
int main()
{
int choice,player=1,i;
char mark;
do{
create_board();
player= (player % 2) ? 1: 2;
printf("Player %d, enter a number: ",player);
scanf("%d",&choice);
mark = (player==1) ? 'X' : 'O';
make_board(choice,mark);
i=check_win();
player++;
}while(i == -1);
create_board();
if(i==1)
printf("Player %d you have won the game",--player);
else
printf("< Draw >");
return 0;
}
void create_board()
{
clear_screen;
printf("\n\n\tTic Tac Toe\n\n");
printf("Player 1 (X) -- Player 2 (O)\n\n");
printf(" | | \n");
printf(" %c | %c | %c \n",box[1],box[2],box[3]);
printf("__________|________|__\n");
printf(" | | \n");
printf(" %c | %c | %c \n",box[4],box[5],box[6]);
printf("__________|__________|__\n");
printf(" | | \n");
printf(" %c | %c | %c \n",box[7],box[8],box[9]);
printf("____________|___________|__\n");
printf(" | | \n");
}
void make_board( int choice, char mark)
{
if(choice==1 && box[1]=='1')
box[1]=mark;
else if(choice==2 && box[2]=='2')
box[2]=mark;
else if(choice==3 && box[3]=='3')
box[3]=mark;
else if(choice==4 && box[4]=='4')
box[4]=mark;
else if (choice==5 && box[5]=='5')
box[5]=mark;
else if (choice==6 && box[6]=='6')
box[6]=mark;
else if (choice==7 && box[7]=='7')
box[7]=mark;
else if (choice==8 && box[8]=='8')
box[8]=mark;
else if (choice==9 && box[9]=='9')
box[9]=mark;
else
{
printf("Invalid move");
}
}
int check_win()
{
if(box[1]==box[2] && box[2]==box[3])
return 1;
else if(box[4]==box[5] && box[5]==box[6])
return 1; // horizontal match
else if (box[7]==box[8] && box[8]==box[9])
return 1;
else if (box[1]==box[4] && box[4]==box[7])
return 1;
else if(box[2]==box[5] && box[5]==box[8]) // vertical match
return 1;
else if(box[3]==box[6] && box[6]==box[9])
return 1;
else if(box[1]==box[5] && box[5]==box[9])
return 1;
else if(box[3]==box[5] && box[5]==box[7]) //diagonal match
return 1;
else if(box[1]!= '1' && box[2]!= '2' && box[3]!= '3' && box[4]!= '4'&& box[5]!= '5' && box[6]!= '6'&& box[7]!= '7' && box[8]!= '8' && box[9]!='9') //no match
return 0;
else
return -1;

Step by stepSolved in 2 steps with 1 images

- #include #include #include "Product.h" using namespace std; int main() { vector productList; Product currProduct; int currPrice; string currName; unsigned int i; Product resultProduct; cin>> currPrice; while (currPrice > 0) { } cin>> currPrice; main.cpp cin>> currName; currProduct.SetPriceAndName (currPrice, currName); productList.push_back(currProduct); resultProduct = productList.at (0); for (i = 0; i < productList.size(); ++i) { Type the program's output Product.h 1 CSE Scanned Product.cpp if (productList.at (i).GetPrice () < resultProduct.GetPrice ()) { resultProduct = productList.at(i); } AM cout << "$" << resultProduct.GetPrice() << " " << resultProduct. GetName() << endl; return 0; Input 10 Cheese 6 Foil 7 Socks -1 Outputarrow_forwardC++ Program #include <iostream>#include <cstdlib>#include <ctime>using namespace std; int getData() { return (rand() % 100);} class Node {public: int data; Node* next;}; class LinkedList{public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val);private: Node* head;}; // function to check data exist in a listbool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false;} // function to delete all data in a listvoid LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete…arrow_forwardc++ data structurearrow_forward
- Write a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; struct Teletype { string name; string phonenum; Teletype *nextaddr; }; void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create bool check(); int main() { int location = 0; int count = 0; char answery_n; Teletype *list, *current; list = new Teletype; current = list; cout << "Please "; do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr;…arrow_forward
- Describe how arrays are used in a struct.arrow_forwardC++ program #include<iostream> #include<fstream> #include<string> using namespace std; const int NAME_SIZE = 20; const int STREET_SIZE = 30; const int CITY_SIZE = 20; const int STATE_CODE_SIZE = 3; struct Customers { long customerNumber; char name[NAME_SIZE]; char streetAddress_1[STREET_SIZE]; char streetAddress_2[STREET_SIZE]; char city[CITY_SIZE]; char state[STATE_CODE_SIZE]; int zipCode; char isDeleted; char newLine; }; void add_data(int no_of_records, ofstream& fout) { struct Customers c; cout << "Enter the Customer data:" << endl; cout << "Name:"; cin >> c.name; cout << "Street Address 1:"; cin >> c.streetAddress_1; cout << "Street Address 2:"; cin >> c.streetAddress_2; cout << "City:"; cin >> c.city; cout << "State:"; cin >> c.state; cout << "Zip Code:"; cin >> c.zipCode; cout << endl; c.customerNumber = no_of_records; c.isDeleted = 'N'; c.newLine = '\n'; cout…arrow_forwardDeclare a student structure that contains : Student's first and last nameStudent IDCreate the following functions: getStudentInfo(void) Declares a single student, uses printf()/scanf() to get inputReturns the single student backprintStudentInfo(student_t *st_ptr) Takes the pointer to a student (to avoid making a copy)Prints out all of the student informationDeclare an array of five studentsUsing a for loop and getStudentInfo() function, get input of all the studentsUsing a for loop and printStudentInfo() function, print all the output of all students.arrow_forward
- C++main.cc file #include <iostream>#include <map>#include <vector> #include "bank.h" int main() { // =================== YOUR CODE HERE =================== // 1. Create a Bank object, name it anything you'd like :) // ======================================================= // =================== YOUR CODE HERE =================== // 2. Create 3 new accounts in your bank. // * The 1st account should belong to "Tuffy", with // a balance of $121.00 // * The 2nd account should belong to "Frank", with // a balance of $1234.56 // * The 3nd account should belong to "Oreo", with // a balance of $140.12 // ======================================================= // =================== YOUR CODE HERE =================== // 3. Deactivate Tuffy's account. // ======================================================= // =================== YOUR CODE HERE =================== // 4. Call DisplayBalances to print out all *active* // account…arrow_forwardC++ Program: #include <iostream>#include <string> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"}; int main(){ // define stack (or queue ) here string origin; string dest; string citypair; cout << "Loading the CONTAINER ..." << endl; // LOAD THE STACK ( or queue) HERE // Create all the possible Airport combinations that could exist from the list provided. // i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ... // DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic. Using the provided 3 char airport codes, create a 6 character string that is the origin &…arrow_forward#ifndef INT_SET_H#define INT_SET_H #include <iostream> class IntSet{public: static const int DEFAULT_CAPACITY = 1; IntSet(int initial_capacity = DEFAULT_CAPACITY); IntSet(const IntSet& src); ~IntSet(); IntSet& operator=(const IntSet& rhs); int size() const; bool isEmpty() const; bool contains(int anInt) const; bool isSubsetOf(const IntSet& otherIntSet) const; void DumpData(std::ostream& out) const; IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const; void reset(); bool add(int anInt); bool remove(int anInt); private: int* data; int capacity; int used; void resize(int new_capacity);}; bool operator==(const IntSet& is1, const IntSet& is2); #endifarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
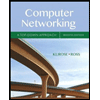
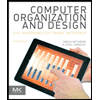
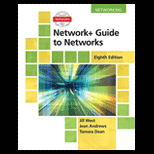
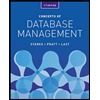
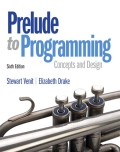
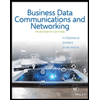