lubMember class class ClubMember: #__init_ function def __init__(self,id,name,gender,weight,phone): self.id = id self.name = name self.gender = gender self.weight = weight self.phone = phone #__str__ function def __str__(self): return "Id:"+str(self.id)+",Name:"+self.name+",
#ClubMember class
class ClubMember:
#__init_ function
def __init__(self,id,name,gender,weight,phone):
self.id = id
self.name = name
self.gender = gender
self.weight = weight
self.phone = phone
#__str__ function
def __str__(self):
return "Id:"+str(self.id)+",Name:"+self.name+",Gender:"+self.gender+",Weight:"+str(self.weight)+",Phone:"+str(self.phone)
#Club class
class Club:
#__init__ function
def __init__(self,name):
self.name = name
self.members = {}
self.membersCount = 0
def run(self):
print("Welcome to "+self.name+"!")
while True:
print("""
1. Add New Member
2. View Member Info
3. Search for a member
4. Browse All Members
5. Edit Member
6. Delete a Member
7. Exit
""")
choice = int(input("Choice:"))
if choice == 1:
self.membersCount = self.membersCount+1
id = self.membersCount
name = input("Member name:")
gender = input("Gender:")
weight = int(input("Weight:"))
phone = int(input("Phone No."))
member = ClubMember(id,name,gender,weight,phone)
self.members[id] = member
elif choice == 2:
name = input("Enter name of member:")
members= self.members
for id,member in members.items():
if name == member.name:
print(member)
break
elif choice == 3:
id = int(input("Enter member id to search:"))
member = self.members[id]
print(member)
elif choice == 4:
print("Details of All members:")
for member in self.members.values():
print(member)
elif choice == 5:
id = int(input("Enter id of member to edit:"))
self.members[id].weight = int(input("Enter new weight of member:"))
self.members[id].phone = int(input("Enter new phone no. of member:"))
elif choice == 6:
id = int(input("Enter id of member to delete:"))
del self.members[id]
elif choice == 7:
break
my code is still providing indent errors and when i feel like ive done all the indents it still gives me errors


Step by step
Solved in 2 steps

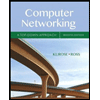
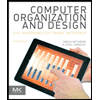
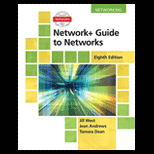
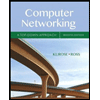
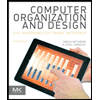
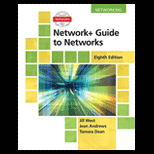
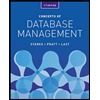
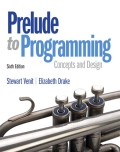
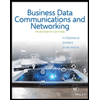